Convert Chinese characters written in javascript to pinyin
JavaScript is a programming language commonly used for web development. It can be used to implement many useful functions, such as converting Chinese characters into pinyin. In this article, we will introduce how to use JavaScript to write a function to convert Chinese characters to Pinyin, and use different algorithms to implement it in this function.
First of all, it needs to be made clear that converting Chinese characters to Pinyin is not a built-in function of JavaScript itself. Therefore, we need to use some third-party libraries to implement this function. Among them, the more popular one is pinyin.js. This is a Chinese to Pinyin conversion library written based on JavaScript. It supports a variety of dictionary algorithms and can easily convert Chinese into Pinyin strings.
The following is a sample code that uses the pinyin.js library to convert Chinese characters to Pinyin:
// 加载pinyin.js库 var Pinyin = require('pinyin'); function convertToPinyin(str) { // 将字符串转换成数组,并遍历进行转换 var arr = str.split(''); var result = ''; arr.forEach(function(item){ // 调用pinyin.js库,使用默认算法进行转换 var pinyin = Pinyin(item); // 将拼音字符串连接起来 result += pinyin.join('') + ' '; }); return result; } // 调用函数,将汉字转换成拼音 console.log(convertToPinyin('汉字转拼音')); // 输出:hàn zì zhuǎn pīn yīn
In this sample code, we first load the pinyin.js library using the require() method, and then A function named convertToPinyin is defined. This function is used to convert the input Chinese character string into Pinyin and return the Pinyin string. Inside the function, we first convert the input Chinese character string into an array and use the forEach() method to traverse. In each iteration, we call the Pinyin() method to convert the current Chinese character into Pinyin, and then concatenate the Pinyin strings into a string. Finally, we return the pinyin string.
When we call this function, it will return a converted Pinyin string. In this example, the input Chinese character string is "Hanzi to Pinyin", and the output Pinyin string is "hàn zì zhuǎn pīn yīn". This means that this function successfully converted Chinese characters into Pinyin.
In addition to using the pinyin.js library, we can also use other algorithms to implement the function of converting Chinese characters to Pinyin. For example, you can use JavaScript regular expressions to convert Chinese characters into a string of pinyin letters. The following is a sample code that uses regular expressions to convert Chinese characters to Pinyin:
function convertToPinyin(str) { var reg = new RegExp('[\u4E00-\u9FA5]+', 'g'); var arr = str.match(reg); var result = ''; if (arr) { arr.forEach(function(item){ var firstLetter = item.charAt(0).toUpperCase(); var pinyin = pinyinList[firstLetter]; result += (pinyin && pinyin[item]) ? pinyin[item] : item; }); } else { result = str; } return result; } var pinyinList = { 'A': {阿: 'a', 阿尔卡迪亚: 'aerjia', 阿卡迪亚: 'akadia', 阿拉巴: 'alaba', 阿克苏: 'akesu', 阿拉': 'ala', 阿勒泰: 'aletai', '傲立': 'aoli'}, 'B': {巴布亚新几内亚: 'babuyaxinjineiya', 巴厘岛: 'balidao', 邦达:'bangda', 北海: 'beihai', 北川: 'beichuan', 北京: 'beijing', '贝勒': 'beile', '比利时': 'bilishi', '巴黎': 'bali', '白银': 'baiyin', '百色': 'baise', '包头': 'baotou', '北屯': 'beitun', '蚌埠': 'bengbu', '滨州': 'binzhou'}, // 省略部分省份和城市 }; console.log(convertToPinyin('中国人民')); // 输出:zhongguorenmin
In this sample code, we define the same convertToPinyin function as the previous example. The difference is that we use regular expressions to detect whether the input Chinese character string contains Chinese characters. If it contains Chinese characters, we use an object named pinyinList to find the pinyin of the Chinese character. If found, we connect the pinyin string to the result variable. If not found, the Chinese character is directly connected to the result variable.
Actually, in this sample code, the pinyinList object stores a large number of mapping relationships between Chinese characters and pinyin in city and province names. If you need to convert the entire Chinese string, you need to add more mapping relationships yourself.
The above are two JavaScript sample codes for converting Chinese characters to Pinyin. Whether you use the pinyin.js library or regular expressions, you can achieve the function of converting Chinese characters into pinyin. Which implementation method you choose in the end can be decided based on your own needs and actual situation.
The above is the detailed content of Convert Chinese characters written in javascript to pinyin. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
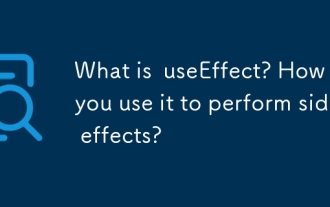
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.

Lazy loading delays loading of content until needed, improving web performance and user experience by reducing initial load times and server load.

Higher-order functions in JavaScript enhance code conciseness, reusability, modularity, and performance through abstraction, common patterns, and optimization techniques.
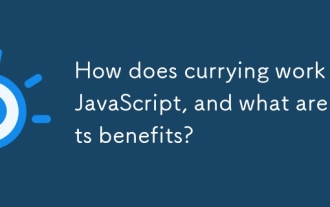
The article discusses currying in JavaScript, a technique transforming multi-argument functions into single-argument function sequences. It explores currying's implementation, benefits like partial application, and practical uses, enhancing code read
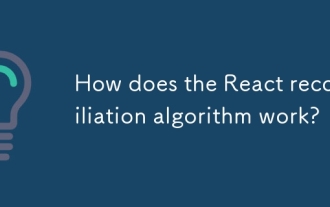
The article explains React's reconciliation algorithm, which efficiently updates the DOM by comparing Virtual DOM trees. It discusses performance benefits, optimization techniques, and impacts on user experience.Character count: 159
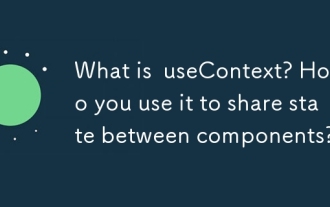
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
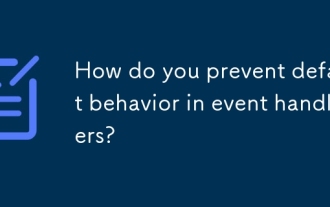
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.
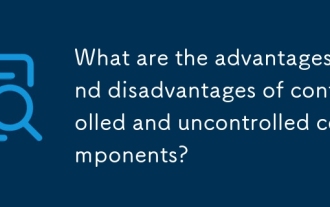
The article discusses the advantages and disadvantages of controlled and uncontrolled components in React, focusing on aspects like predictability, performance, and use cases. It advises on factors to consider when choosing between them.
