javascript json to str
1. What is JSON?
JSON (JavaScript Object Notation) is a lightweight data exchange format derived from the object and array formats in the JavaScript language. It has become one of the most commonly used data exchange formats in modern applications because it is easy to read and write, as well as easy for machines to parse and generate.
JSON is essentially a string that represents complex data in a way that has simple syntax, is self-descriptive, and is easy to read and understand. In web applications, JSON is often used instead of XML as the format for data exchange.
2. Convert JSON to String
Converting a JSON object to a string is usually called "serialization". In JavaScript, it's easy to serialize a JSON object into a string. JSON.stringify() can accomplish this task. The syntax is as follows:
JSON.stringify(value[, replacer[, space]])
Among them:
- value: required, the json object to be converted
- replacer: optional, parameters used to control the conversion process, you can Is a function or an array
- space: Optional, the number of spaces used to indent the output.
Here is a simple example:
var obj = {name: "John", age: 30, city: "New York"}; var str = JSON.stringify(obj); console.log(str); //输出{"name":"John","age":30,"city":"New York"}
In this example, we serialize the JavaScript object obj into a JSON string. You can see that a JSON formatted string is output.
3. Convert JSON string to object
The JSON.parse() method in JavaScript can convert a string in JSON format into a JavaScript object. The syntax is as follows:
JSON.parse(text[, reviver])
Where:
- text: required, the JSON string to be parsed
- reviver: optional, the parser is used to convert each character in the result A (key, value) pair is returned after trimming
Here is a simple example:
var str = '{"name":"John","age":30,"city":"New York"}'; var obj = JSON.parse(str); console.log(obj); //输出{name: "John", age: 30, city: "New York"}
In this example, we parse a JSON string into a JavaScript object. You can see that a JavaScript object is output.
4. Complete example
The following is a complete example that demonstrates how to convert a JSON object to a string and then convert it back to the original object:
// 定义JSON对象 var person = { "name": "John", "age": 30, "city": "New York", "hobbies": ["reading", "sports", "music"], "married": false, "salary": null } // 序列化JSON对象为字符串 var jsonString = JSON.stringify(person); console.log(jsonString); // 将JSON字符串解析回对象 var personObject = JSON.parse(jsonString); console.log(personObject);
In this example, we first define a JSON object person, which contains various different types of attributes. We then use the JSON.stringify() method to serialize the object into a string and then use JSON.parse() to parse it back to the original object. The result is the same JSON object.
5. Summary
JSON format data can be easily processed using JavaScript's JSON.stringify() and JSON.parse() methods. These methods are also used for data exchange in Web APIs because JSON is a format that is human-friendly and easy to read and write, and does not require the use of additional markup to describe the data like XML does. Therefore, in web development, data exchange using JSON format has become a common standard.
The above is the detailed content of javascript json to str. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics



React combines JSX and HTML to improve user experience. 1) JSX embeds HTML to make development more intuitive. 2) The virtual DOM mechanism optimizes performance and reduces DOM operations. 3) Component-based management UI to improve maintainability. 4) State management and event processing enhance interactivity.
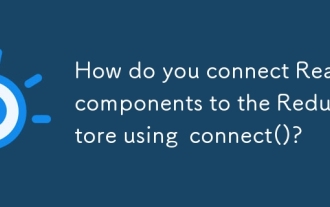
Article discusses connecting React components to Redux store using connect(), explaining mapStateToProps, mapDispatchToProps, and performance impacts.
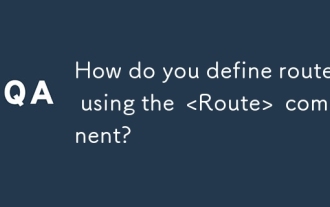
The article discusses defining routes in React Router using the <Route> component, covering props like path, component, render, children, exact, and nested routing.
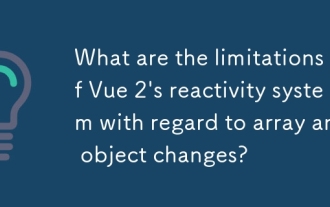
Vue 2's reactivity system struggles with direct array index setting, length modification, and object property addition/deletion. Developers can use Vue's mutation methods and Vue.set() to ensure reactivity.
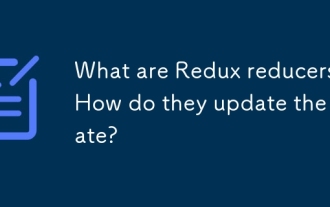
Redux reducers are pure functions that update the application's state based on actions, ensuring predictability and immutability.
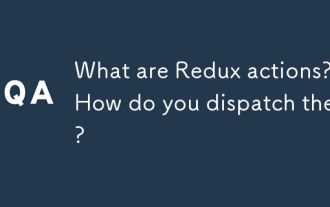
The article discusses Redux actions, their structure, and dispatching methods, including asynchronous actions using Redux Thunk. It emphasizes best practices for managing action types to maintain scalable and maintainable applications.
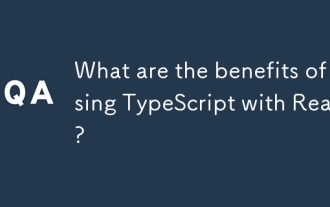
TypeScript enhances React development by providing type safety, improving code quality, and offering better IDE support, thus reducing errors and improving maintainability.

React components can be defined by functions or classes, encapsulating UI logic and accepting input data through props. 1) Define components: Use functions or classes to return React elements. 2) Rendering component: React calls render method or executes function component. 3) Multiplexing components: pass data through props to build a complex UI. The lifecycle approach of components allows logic to be executed at different stages, improving development efficiency and code maintainability.
