##Same class in the same package | ✓ | ✓ | ✓ | ✓ |
##Different classes in the same package
|
✓ |
✓ |
✓ |
|
Subclasses in different packages
|
|
✓ |
✓ | |
Non-subclasses in different packages
|
|
|
✓ |
|
???? (Public) public: The members, methods or classes it modifies can be accessed anywhere.
????(inherited) protected: see the back,
????(default) default: access in the same package
???? (Private) private: can only be accessed in the current class
public class TestDemo01 {
int year;
//默认权限,可以省略default
private int month;
//私有权限,只能在同一个类中访问
public int day;
//公有权限,那里都能访问
}
Copy after login
Demonstrate below
In the first class TestDemo01
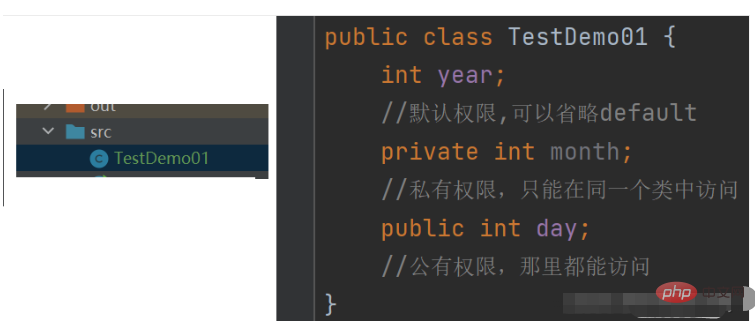
In the two classes TestDemo02
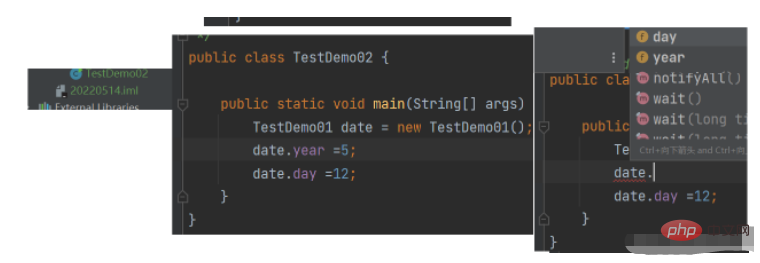
we found that month cannot be found. This is because we wrote its access permission as private and made it private. The class cannot be accessed. This is the implementation of encapsulation, month can only be used in TestDemo01.
We implement encapsulation in order to leave only the interface and not show the details.
Let’s demonstrate the encapsulated interface
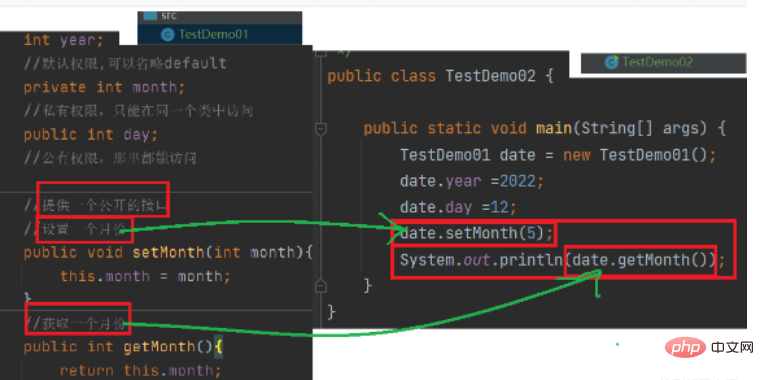
If It is said that many members are private. We don’t need to write these two interfaces one by one. We can use the function of idea to write them quickly.
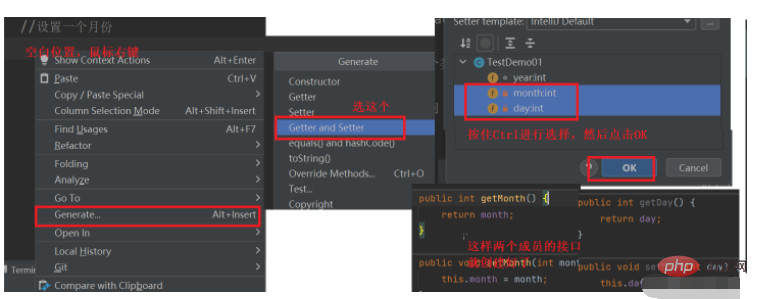
3. Understand the requirements for encapsulation To know - package
3.1 Understand the concept of package
The existence of package is to better manage classes, collect multiple classes together into a group, group them and similar directories
Package is the embodiment of the encapsulation mechanism of classes, interfaces, etc. It is a good way of organizing classes or interfaces, etc.
Package also has a very important role in that it can be used in the same project Classes with the same name are allowed in , as long as they are in different packages.
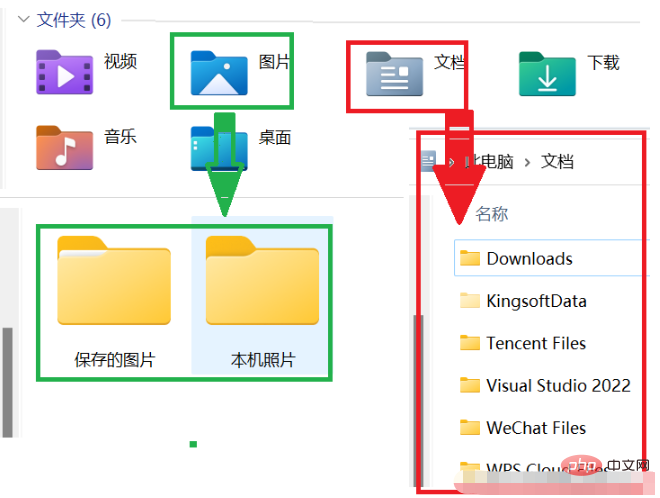
3.2 Import classes in the package
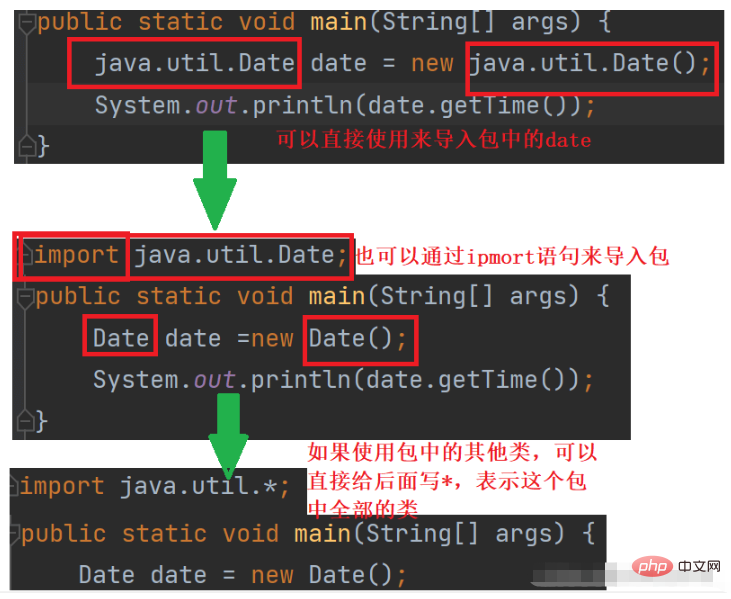
Let’s see why the error is reported
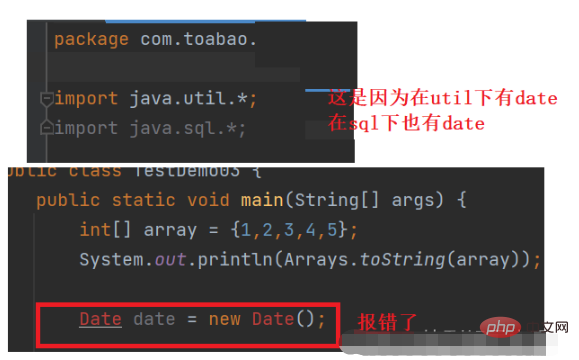
How to solve this problem, you need to specify a specific package
package com.toabao.www;
import java.util.*;
import java.sql.*;
public class TestDemo03 {
public static void main(String[] args) {
int[] array = {1,2,3,4,5};
System.out.println(Arrays.toString(array));
java.util.Date date = new java.util.Date();
java.sql.Date date1 = new java.sql.Date(12);
}
}
Copy after login
Use import static to import static methods and fields in the package
import static java.lang.Math.*;
public class TestDemo01 {
public static void main(String[] args) {
double x = 13;
double y = 14;
double result = sqrt(pow(x,2)+pow(y,2));
}
}
Copy after login
3.3 Customize the package
Look at the steps first. If you don’t know how, you can try to create a package yourself
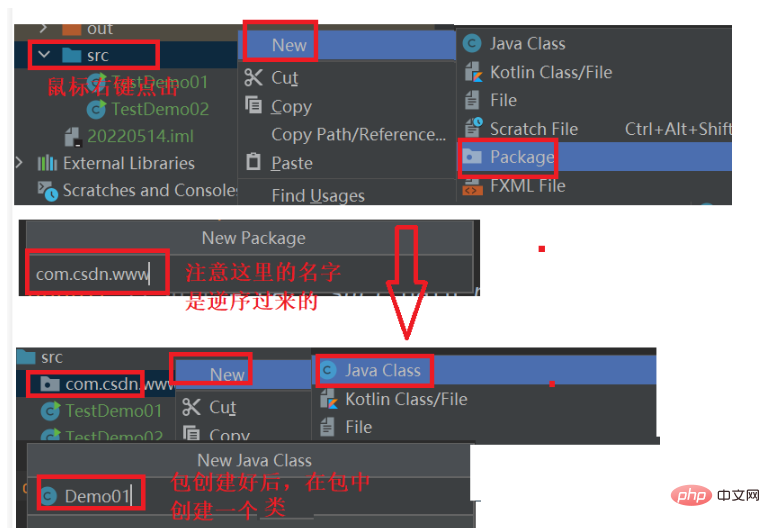
Note:
1. After the package is created , in the file, there must be a package statement at the top to indicate which package the file is in (idea will automatically add it)

2. The naming of the package is usually For example, it is the reverse name of the domain name
3. We can take a look at the location where the code is stored. The package we just created

3.4 Access permission control
The same class in the same package
package Demo01;
public class Test01 {
int count = 10;
public void func(){
System.out.println(count);
}
}
Copy after login
Access of different classes in the same package
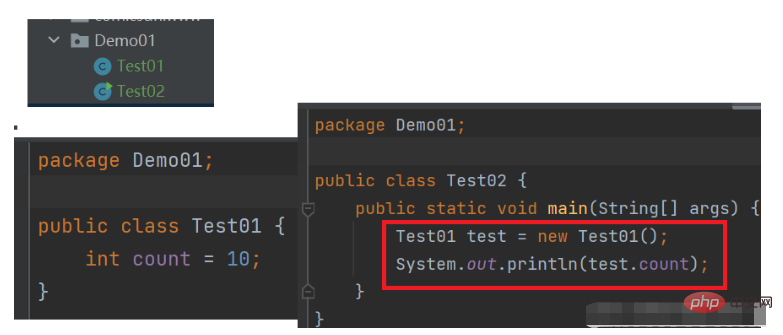
In different packages The access permissions of the subclasses
the previous count are default, that is, default. Subclasses and non-subclasses in different packages cannot be accessed
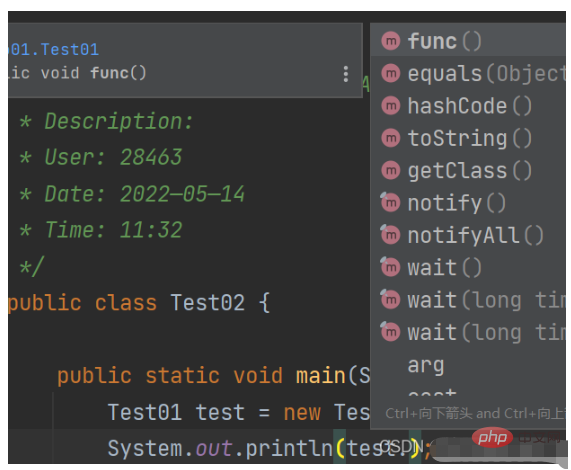
If you must access, you can change the access permission to public,
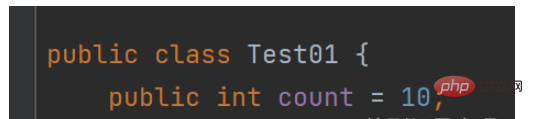
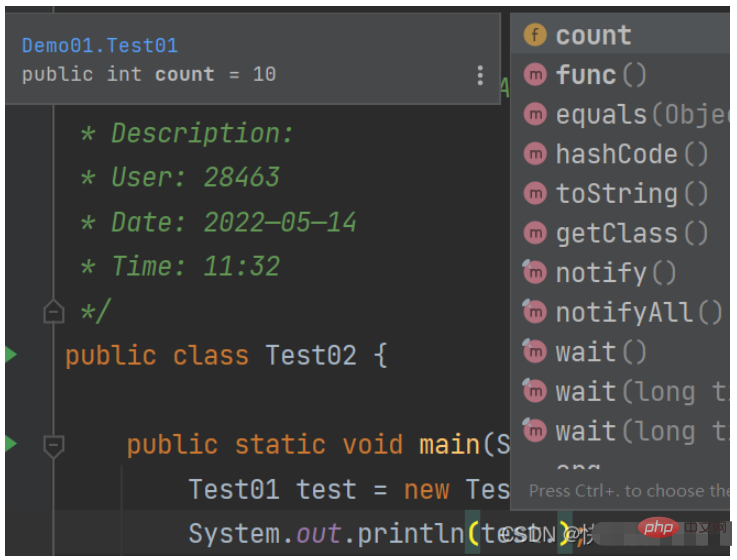
3.5 Common packages in java
1. java.lang: Commonly used basic classes in the system (String, Object), you don’t need to write them after JDK1.1, they will be imported automatically,
2. java.lang.reflflect:java reflection programming package
3. java.net: Network programming development package
4. java.sql: Support package for database development
5. java.util: It is a tool package provided by java
6. java.io:I/O programming development package