golang map remove elements
Golang is a strongly typed, statically compiled language, and it is also a language with relatively high development efficiency. In development, collection data structures are often needed, and map in golang is a powerful collection data structure. Map stores a set of key-value pairs, and the corresponding value can be quickly located through the key. When using map, sometimes you need to remove elements. Next, let's talk about how to remove map elements in golang.
map in golang
The map in golang is a reference type, which is similar to a dictionary or hash table in other languages. It stores a set of key-value pairs, where the key Is unique, non-repeatable, and the value can be repeated. The bottom layer of the data structure of map in golang is a hash table. When using map, you need to pay attention to the following points:
- map is a reference type and needs to be created using the make function.
- map can be iterated using for-range syntax.
- The keys of the map can be any type that can be compared for equality, such as integers, strings, structures, etc.
- Map is unordered, and the order of each traversal may be different.
How to remove map elements in golang
In golang, we can remove map elements by using the delete()
function. delete()
The syntax format of the function is as follows:
delete(map, key)
Among them, map represents the map to be operated, and key represents the key to be deleted. When using the delete function, you need to pay attention to the following points:
- Deleting non-existent key-value pairs will not report an error.
- If you accidentally delete a non-existent key-value pair, it will not cause the program to crash.
- When multiple goroutines operate map at the same time, locking is required to avoid competition.
- If a large number of key-value pairs are deleted, the hash table of the map may be rehashed, affecting performance.
Sample code for removing map elements in golang
Let's use the sample code to learn more about how to remove map elements in golang.
package main import ( "fmt" ) func main() { // 创建一个map testMap := make(map[string]int) testMap["apple"] = 1 testMap["banana"] = 2 testMap["orange"] = 3 // 删除map中的某个元素 delete(testMap, "apple") // 遍历map for key, value := range testMap { fmt.Println(key, value) } }
The output results are as follows:
banana 2 orange 3
In the above example code, we created a map named testMap
and added three key-value pairs to it . Then, we use the delete()
function to remove the element with the key "apple" from the testMap
. Finally, we use the for-range syntax to traverse testMap
and output the remaining elements in the map. As can be seen from the output, the element with the key "apple" has been successfully removed.
Regarding the removal of map elements, in addition to using the delete()
function, you can also set the value corresponding to the key that needs to be removed to a specific zero value (such as 0, " ", nil, etc.). However, this method only applies to cases where the value is a basic type. For cases where the value is a composite type (such as pointers, structures, etc.), you need to use the delete()
function to remove it.
Summary
The map in golang is a powerful collection data structure that can be used to store a set of key-value pairs. When using map, sometimes you need to remove elements. In this case, you can use the delete()
function to complete it. It should be noted that locking is required when multiple goroutines operate the map at the same time to avoid competition. At the same time, if elements need to be deleted frequently, it is recommended to use other data structures instead of map.
The above is the detailed content of golang map remove elements. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


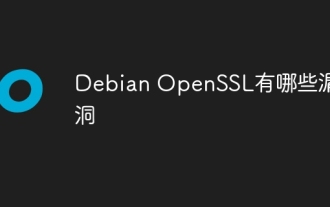
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
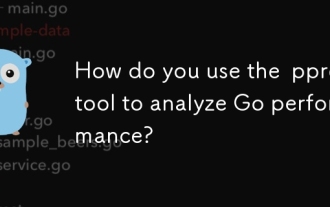
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
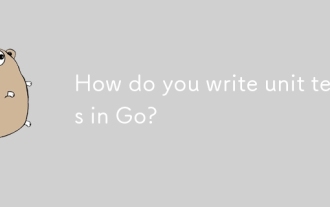
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
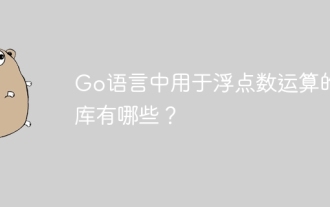
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
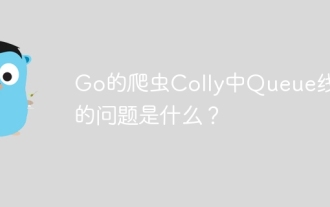
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
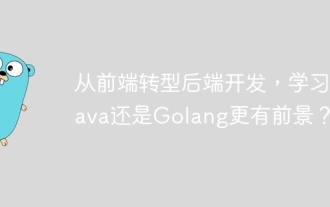
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
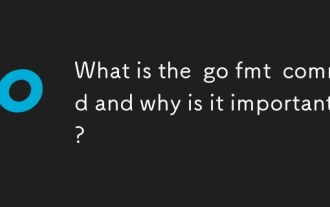
The article discusses the go fmt command in Go programming, which formats code to adhere to official style guidelines. It highlights the importance of go fmt for maintaining code consistency, readability, and reducing style debates. Best practices fo
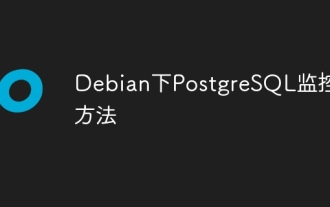
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
