The difference between golang pointer methods
Golang is a language that supports object-oriented programming, and pointers and methods are often used in actual development. A pointer is a variable that stores the memory address of a variable. A method is a function that specifies a type. There is a difference between pointer methods and non-pointer methods in Golang. This article will explore the differences and uses of these two methods.
1. Pointer method
The pointer method is a method bound to a structure pointer. Pointer methods have the following characteristics:
- The receiver type of a pointer method is a pointer to a certain type.
- Pointer methods can modify the value pointed to by the receiver.
- If a type's method needs to modify its receiver itself, it must use pointer methods.
For example, we define a structure type:
type Rectangle struct { width float64 height float64 }
We can use pointer methods to modify instances of this structure:
func (r *Rectangle) SetProperty(width, height float64) { r.width = width r.height = height }
In the above method, Receiver (r *Rectangle)
is a pointer to a Rectangle structure. This method is used to set the properties of Rectangle.
2. Non-pointer method
The non-pointer method is a method bound to a structure. It accepts a copy of the structure as the receiver parameter. The non-pointer method has the following characteristics :
- The receiver type of a non-pointer method is a copy of the value of the type.
- Non-pointer methods cannot modify the value of the receiver.
For example, we define a structure type:
type Rectangle struct { width float64 height float64 }
We can use non-pointer methods to calculate the area of the structure:
func (r Rectangle) GetArea() float64 { return r.width * r.height }
In the above method , the receiver r Rectangle
is a copy of the value of the Rectangle structure. This method returns the area of the rectangle.
3. The difference between pointer methods and non-pointer methods
- Pointer methods can modify the properties in the receiver, but non-pointer methods cannot.
- Pointer methods can avoid copying the object when called because they can directly access the address of the object. This feature is particularly useful when working with large structures.
- Non-pointer methods must copy the entire object when called, which may cause performance issues.
- When defining a method, you can use non-pointer methods if you do not need to modify the receiver itself, otherwise you must use pointer methods.
For example, we can use pointer methods to modify the structure:
func main() { rect := Rectangle{width: 10, height: 5} fmt.Println("Width:", rect.width, "Height:", rect.height) rect.SetProperty(20, 10) fmt.Println("Width:", rect.width, "Height:", rect.height) }
Output result:
Width: 10 Height: 5 Width: 20 Height: 10
4. Summary
In Golang Among them, pointer methods and non-pointer methods have their own advantages, disadvantages and usage scenarios. When defining methods, you need to consider the usage of the object and business needs, and choose an appropriate method to write code. Proper use of both methods can improve code readability and performance.
The above is the detailed content of The difference between golang pointer methods. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
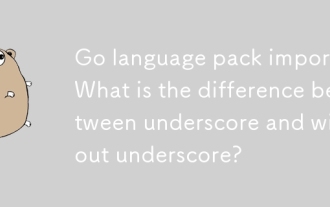
This article explains Go's package import mechanisms: named imports (e.g., import "fmt") and blank imports (e.g., import _ "fmt"). Named imports make package contents accessible, while blank imports only execute t

This article explains Beego's NewFlash() function for inter-page data transfer in web applications. It focuses on using NewFlash() to display temporary messages (success, error, warning) between controllers, leveraging the session mechanism. Limita
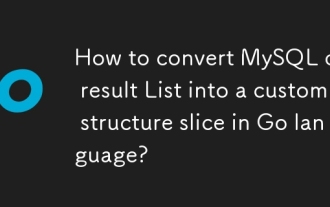
This article details efficient conversion of MySQL query results into Go struct slices. It emphasizes using database/sql's Scan method for optimal performance, avoiding manual parsing. Best practices for struct field mapping using db tags and robus
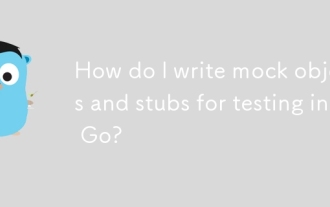
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
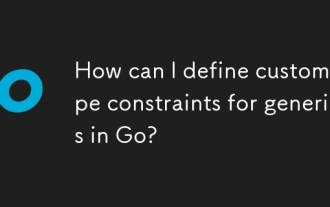
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
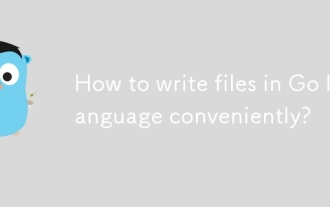
This article details efficient file writing in Go, comparing os.WriteFile (suitable for small files) with os.OpenFile and buffered writes (optimal for large files). It emphasizes robust error handling, using defer, and checking for specific errors.
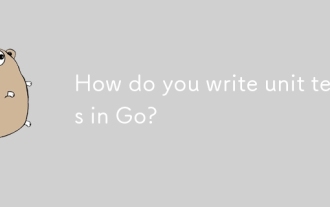
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
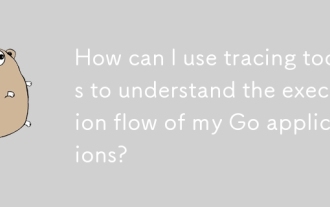
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
