golang type conversion ok
Go language (Golang) is an open source programming language influenced by languages such as C, Java, and Python. It was developed by Google to create efficient and reliable software. A program is closely related to the language in which it is written. Like the language that expresses emotions and thoughts, programming languages also have their own unique characteristics. Type conversion is a very important concept in the Go language, because type conversion is used in many situations. This article will introduce the relevant knowledge of Golang type conversion.
1. Overview of type conversion
Type conversion is the process of converting a value of one data type into a value of another data type. In Go language, data types are divided into basic data types and composite types. Basic data types include int, float, string, bool and other types. Composite types include arrays, slices, dictionaries, structures, interfaces, and functions. In the Go language, values between different types cannot be directly operated and compared. Therefore, it is necessary to type-convert values between different types. Golang supports conversion of signed integers, unsigned integers, floating point numbers, Boolean, strings and other types. The syntax for type conversion is: T(x), where T represents the type to be converted and x represents the value to be converted.
2. Basic data type conversion
1. Integer type conversion
In Go language, integer types include signed integers and unsigned integers. The integer types that support conversion are int8, int16, int32, int64, uint8, uint16, uint32 and uint64. Among them, int8 and uint8 are called byte types, int16 and uint16 are called short integer types, int32 and uint32 are called long integer types, and int64 and uint64 are called long integer types.
You need to pay attention to the following two points when converting integer types:
- When converting, if the value range exceeds the range of the type value to be converted, it will overflow, resulting in inaccurate results. For example, converting a value larger than the int8 range to the int8 type will result in inaccurate values in the range [-128, 127]. Overflow problems with integer types need to be avoided.
- Only conversions of the same type or from low-precision types to high-precision types are safe. For example, converting from int8 to int16 is safe, but converting from int16 to int8 is unsafe because some data may be intercepted when converting to int8, resulting in inaccurate results.
The following are some examples of integer type conversion:
package main import "fmt" func main() { var a int32 = 100 var b int64 = int64(a) // int32转换成int64 var c int8 = int8(a) // int32转换成int8,可能溢出 var d uint16 = uint16(a) // int32转换成uint16 fmt.Println(b, c, d) }
The output result is:
100 100 100
2. Floating point type conversion
In Go In the language, floating-point number types include float32 and float64, and the only floating-point number types that support conversion are float32 and float64. There are two points to note when converting floating-point number types:
- If the value range is too large or too small during conversion, overflow may occur.
- Can only be converted from a low-precision type to a high-precision type. Conversion from a high-precision type to a low-precision type may result in loss of precision.
The following is an example of floating point type conversion:
package main import "fmt" func main() { var a float32 = 3.1415926 var b float64 = float64(a) // float32转换成float64 fmt.Println(b) }
The output result is:
3.1415927410125732
3. Boolean type conversion
In Go In the language, the Boolean type has only two values: true and false, and the only types that support conversion are int and string types. When converting a Boolean value to an int, true is converted to 1 and false is converted to 0. When converting a Boolean value to a string, true is converted to "true" and false is converted to "false".
The following is an example of Boolean type conversion:
package main import "fmt" func main() { var a bool = true var b int = int(a) // true转换成int,值为1 var c string = string(a) // true转换成字符串,值为"true" fmt.Println(b, c) }
The output result is:
1 true
4. String type conversion
In Go language, A string is an (immutable) array composed of character sequences, and the only types that support conversion are primitive types. String conversion can be achieved through the strconv package. When converting integers to strings, you can use the strconv.Itoa() function, and when converting floating-point numbers to strings, you can use the strconv.FormatFloat() function.
The following is an example of string type conversion:
package main import ( "fmt" "strconv" ) func main() { var a int = 100 var b string = strconv.Itoa(a) // 将整数转换成字符串 var c float64 = 3.1415926 var d string = strconv.FormatFloat(c, 'f', 6, 64) // 将浮点数转换成字符串,保留6位小数 fmt.Println(b, d) }
The output result is:
100 3.141593
3. Composite type conversion
1. Array type conversion
In the Go language, an array is a fixed-length sequence composed of elements of the same type. Values can be assigned directly between arrays, but it should be noted that the size and type of the arrays must be the same, otherwise a compilation error will occur.
The following is an example of array type conversion:
package main import "fmt" func main() { var a [3]int = [3]int{1, 2, 3} var b [3]int = a // 数组之间可以直接赋值 fmt.Println(b) }
The output result is:
[1 2 3]
2. Slice type conversion
In Go language, slice It is a structure that contains a pointer to an array, the length and capacity of the slice, and is a variable-length sequence. Values can be assigned directly between slices, but it should be noted that the element types of the slices must be the same.
The following is an example of slice type conversion:
package main import "fmt" func main() { a := []int{1, 2, 3, 4} b := a // 切片之间可以直接赋值 fmt.Println(b) }
The output result is:
[1 2 3 4]
3. Dictionary type conversion
In Go language, dictionary Is a collection of key-value pairs. Values can be assigned directly between dictionaries, but it should be noted that the key and value types of the dictionaries must be the same.
The following is an example of dictionary type conversion:
package main import "fmt" func main() { a := map[string]int{"apple": 1, "banana": 2} b := a // 字典之间可以直接赋值 fmt.Println(b) }
The output result is:
map[apple:1 banana:2]
4. Summary
In the Go language, type conversion is a very important concept. Type conversion can convert values between different types to meet the needs of the program. However, it should be noted that when performing type conversion, it is necessary to avoid data type range overflow and precision loss problems, and at the same time, it is necessary to ensure that the type after conversion is compatible with the type before conversion. Type conversion is applied between basic data types and composite data types. Mastering the relevant knowledge of type conversion is very important for Golang programming.
The above is the detailed content of golang type conversion ok. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
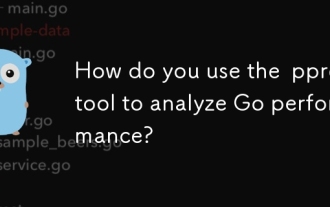
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
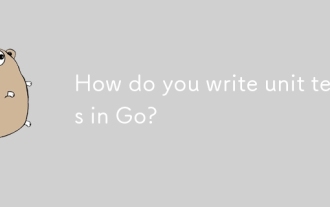
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
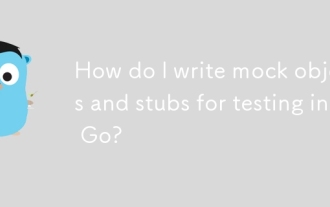
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
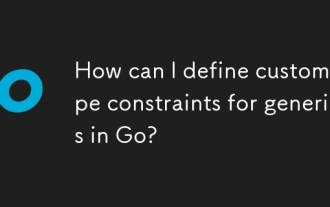
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
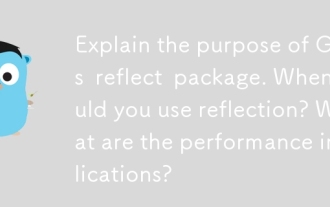
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
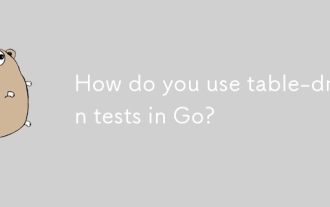
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
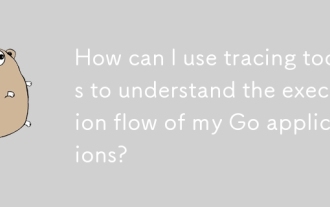
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
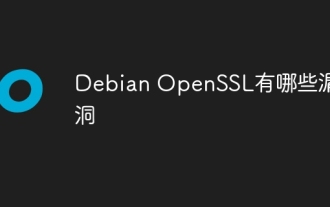
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
