How to implement custom types in Go?
Go is an object-oriented, statically typed programming language, thus allowing developers to simplify code and improve readability through custom types. In this article, we will explore how to implement custom types in Go so that developers can better take advantage of this feature.
- Structure
A structure in Go is a custom type that allows developers to implement more complex structures by combining variables of different types. data structure. The following is an example of a structure:
type Person struct { name string age int address string }
In this example, we define a structure named Person, which contains three member variables: name, age, and address. These variables are of different types, but they are grouped together to represent information about a person. We can create a new Person object using the following method:
person1 := Person{name: "John Doe", age: 30, address: "123 Main St."}
In this example, we use a structure literal to initialize the person1 object. Then, we can use dot notation to access the value of the structure member variable:
fmt.Println(person1.name) // John Doe fmt.Println(person1.age) // 30 fmt.Println(person1.address) // 123 Main St.
- Type alias
Type alias is a method of naming an existing type into a new type Way. It can be used to simplify code or define more descriptive names for existing types. Here is an example of a type alias:
type Celsius float64
In this example, we define a type alias called Celsius, which is actually a float64 type. We can then create a new Celsius object using:
temperature := Celsius(23.5)
In this example, we used type conversion to convert the float64 type 23.5 to the Celsius type. We can then access the value of the temperature variable using dot notation:
fmt.Println(temperature) // 23.5
- Custom Type
A custom type is a wrapper of an existing type in a new type way. This can be used to define a more descriptive name, add new methods, or change the behavior of the type. The following is an example of a custom type:
type Feet float64 func (f Feet) Inches() float64 { return float64(f * 12) }
In this example, we define a custom type called Feet, which is actually a float64 type. We then define a method on the Feet type called Inches that converts a Feet object to inches and returns that value. Here is an example of how to use the Feet object:
distance := Feet(10.5) fmt.Println(distance.Inches()) // 126
In this example, we have used the Inches method of the Feet object to calculate the distance in inches. This way we can use more descriptive names in our code and add custom behavior to existing types.
In short, custom types are a very useful feature in Go, which can help developers simplify code and improve readability. Through structures, type aliases, and custom types, developers can easily define new data types and add custom behaviors to them.
The above is the detailed content of How to implement custom types in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


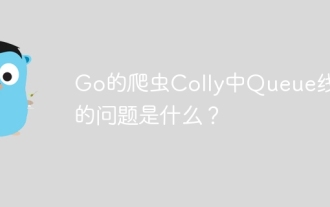
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
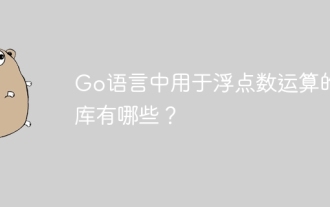
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
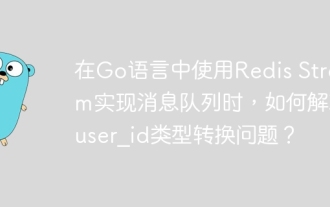
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
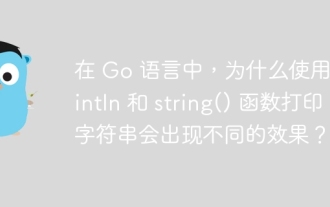
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
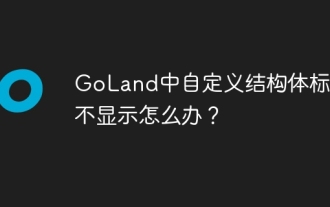
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
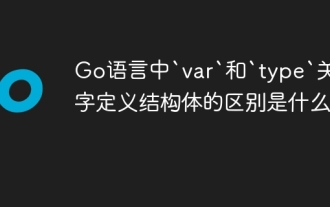
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
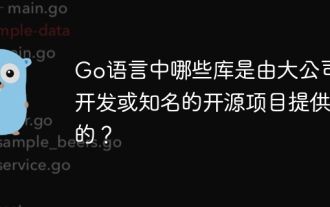
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
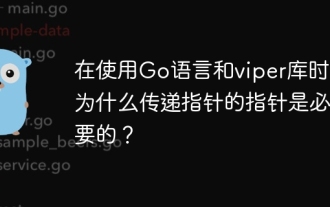
Go pointer syntax and addressing problems in the use of viper library When programming in Go language, it is crucial to understand the syntax and usage of pointers, especially in...
