c golang type conversion
In Golang, type conversion is the process of converting a value of one data type into a value of another data type. Since Golang's type system is static, the types that need to be converted must be specified, and great care should be taken when doing type conversions to avoid errors and unexpected behavior.
This article will introduce the basic knowledge of type conversion in Golang, including how to perform type conversion, problems that may arise in type conversion, best practices for type conversion, etc.
1. Basic knowledge of type conversion
In Golang, the syntax of type conversion is as follows:
var1 := type1(value) var2 := type2(var1)
Among them, type1 and type2 respectively represent the data type that needs to be converted, value is the value that needs to be converted. It is worth noting that if the value to be converted is a constant, it must be converted to a variable before type conversion.
Let us use some examples to gain a deeper understanding of the use of type conversion.
1. Convert integer type to floating point type.
var a int = 10 var b float64 = float64(a)
2. Convert string type to integer type.
var str string = "1024" var num int, err = strconv.Atoi(str) if err == nil { fmt.Println(num) }
2. Frequently Asked Questions about Type Conversion
Some problems may arise when performing type conversion. Some of the most common problems are listed below.
1. Invalid conversion
When an invalid conversion is performed, the Golang program will generate a compile-time error, because the type conversion is performed at compile time, if one type cannot be converted to another type , an error will be reported.
2. Truncation
If the value of the integer type is greater than the maximum value that can be represented by the target type, truncation will occur during type conversion, and precision will be lost at this time, and the result may appear Unexpected results.
3. Precision loss
When converting integers to floating point numbers, if the value of the original integer variable exceeds the representation range of the target floating point number type, there will be a problem of precision loss, resulting in The results are inaccurate.
4. Data overflow
When performing large-range numerical conversion, if the target type cannot represent the value of the original data type, data overflow problems will occur, resulting in incorrect results.
3. Best practices for type conversion
The following are the best practices that need to be followed when performing type conversion.
1. Always use type conversion
When performing type conversion, you should always use the type(value) syntax instead of the mandatory assertion (type_assertion) method. type_assertion can only be used for values of interface types, and if the type assertion fails, the program will crash.
2. Use the strconv standard library to convert strings and numeric types
When converting string types to numeric types, you should use the functions in the strconv standard library, such as Atoi and Itoa and ParseInt et al. Because strconv can detect errors during conversion, it avoids unknown errors when the program is running.
3. Avoid conversions that cause precision loss
When numerical conversion is required, precision loss issues should be avoided as much as possible. You should try to use a data type that can represent a larger range, such as float64 instead of float32, to ensure conversion accuracy.
4. Avoid data overflow problems
When converting a large range of numerical types, data inspection and overflow judgment should be performed. You can use variables and constants in the Golang language to understand the maximum value that the target type can represent to ensure correctness.
Conclusion
Type conversion is a very important part of Golang, because it can convert between different data types, providing more flexibility for program development. But when doing type conversion, you need to pay attention to follow best practices to avoid unnecessary errors and problems. Through the introduction of this article, I believe that readers have a deeper understanding of the type conversion mechanism in Golang, which will help you perform type conversion more flexibly and efficiently in future programming development.
The above is the detailed content of c golang type conversion. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
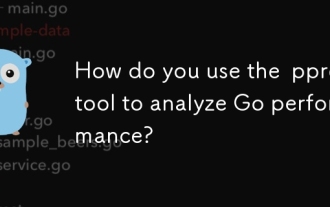
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
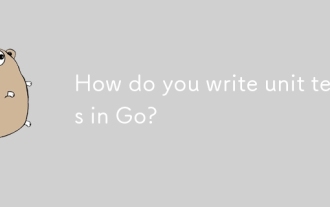
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
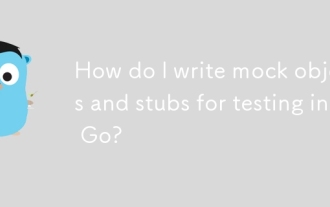
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
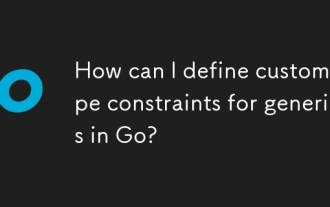
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
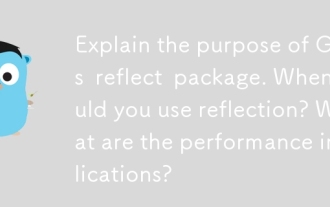
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
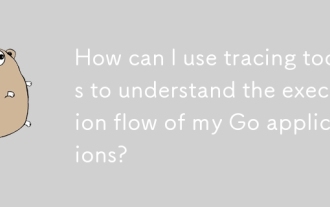
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
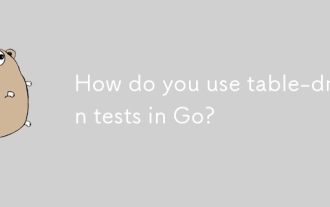
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
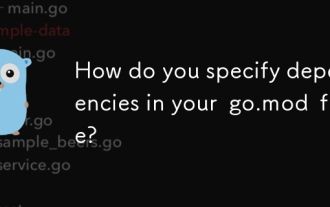
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.
