golang text to image
Recently, more and more websites and applications have begun to use the text-to-image function to protect users' privacy and ensure the security of information. Therefore, learning how to convert text into images using Golang is a very valuable skill. This article will show you how to implement this functionality in just a few lines of code using Golang.
First, we need to import the following libraries:
import ( "bytes" "image" "image/color" "image/draw" "image/png" "golang.org/x/image/font" "golang.org/x/image/font/basicfont" "golang.org/x/image/math/fixed" )
After importing the required libraries, we need to create a function named GenerateTextImage()
to accept parameters and generate image. This function will return an image of type []byte
.
func GenerateTextImage(text string, width int, height int) []byte { // create a new image img := image.NewRGBA(image.Rect(0, 0, width, height)) // set image background color to white white := color.RGBA{255, 255, 255, 255} draw.Draw(img, img.Bounds(), &image.Uniform{white}, image.ZP, draw.Src) // add text to image c := freetype.NewContext() c.SetDPI(72) c.SetFont(basicfont.NewDrawFont()) c.SetFontSize(20) c.SetClip(img.Bounds()) c.SetDst(img) c.SetSrc(image.Black) pt := freetype.Pt(10, 10+int(c.PointToFixed(20)>>6)) _, err := c.DrawString(text, pt) if err != nil { fmt.Println(err) } // encode the image to png format and return the bytes var buff bytes.Buffer png.Encode(&buff, img) return buff.Bytes() }
In the above code block, we first create a blank RGBA image. We also set the color of the image background so that it is white.
Next, we add text to the image. We use the NewContext()
function from the freetype font library to create a new context and set the font and font size. We also specify the window size to which the text will be added. We then specify black as the font color and the text position on the image.
Finally, we encode the image into PNG format and return it as a byte slice.
To call this function, you just need to pass the text you want to add to the image, the width and height of the image. For example:
text := "Hello, World!" width := 200 height := 50 imageBytes := GenerateTextImage(text, width, height)
In the above code block, we add "Hello, World!" to the image, set the width of the image to 200 pixels and the height to 50 pixels, and store the resulting image byte array in the imageBytes
variable.
This is a simple way to convert text to image using Golang. By using the freetype library and the image packages from the standard library, we can easily create high-quality text images.
The above is the detailed content of golang text to image. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


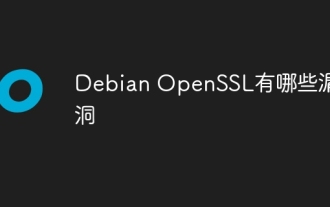
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
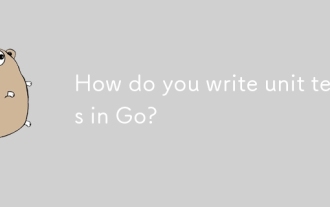
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
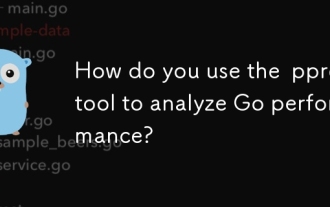
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
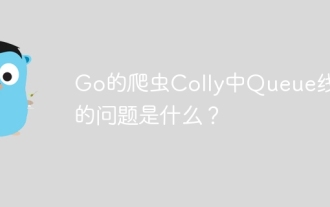
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
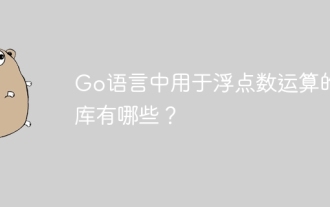
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
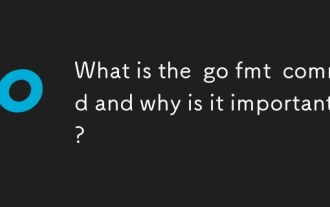
The article discusses the go fmt command in Go programming, which formats code to adhere to official style guidelines. It highlights the importance of go fmt for maintaining code consistency, readability, and reducing style debates. Best practices fo
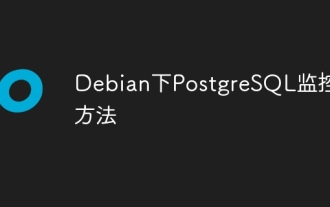
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
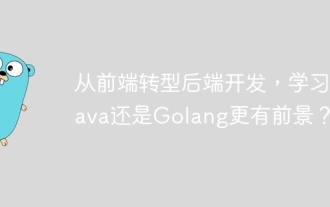
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
