Add, delete, modify and check vue list page
As Vue.js becomes more and more popular, the demand for Vue applications will inevitably increase. As the importance of list pages continues to increase in most web applications, Vue list page addition, deletion, and modification has not only become a very important application requirement, but also one of the most important things that Vue learners should learn. This article will introduce how to use Vue.js to perform basic add, delete, modify and check operations on list pages.
Preparation:
Before starting this article, please make sure you have enough understanding of the basics of HTML and JavaScript, because we will use Vue.js, HTML, and JavaScript to create a list application program.
This article covers the following topics:
1. Creating a Vue application
2. Creating a list
3. Adding new entries
4. Edit entry
5. Delete entry
1. Create Vue application
We need to install Vue.js. It can be installed via CDN or via the npm package manager. If you want to install via a CDN, you can get it from: https://unpkg.com/vue@next/dist/vue.global.js. If you want to install using the npm package manager, you can use the following command in the terminal:
npm install vue
After the installation is complete, now we can create a Vue instance as follows:
const app = Vue.createApp({ });
Next , we will define templates, data, methods and computed properties in the instance.
2. Create a list
We will use the v-for instruction to loop through the list data. Suppose we have an array that stores list item data. We can declare this array as a data model in the Vue instance as follows:
const app = Vue.createApp({ data() { return { items: [ { id: 1, name: 'Item 1' }, { id: 2, name: 'Item 2' }, { id: 3, name: 'Item 3' }, { id: 4, name: 'Item 4' }, { id: 5, name: 'Item 5' } ] } } });
Now, we need to use the v-for directive in the template to iterate through this array and display the data for each item. To do this, we will add the following code to the Vue template:
<template> <ul> <li v-for="item in items" :key="item.id">{{ item.name }}</li> </ul> </template>
The above code will use the v-for directive to loop through the data array and use the keyword:key to provide a unique identifier for each item element.
Now we have finished creating the list.
3. Add new entries
We will use the v-model
directive provided by Vue to create a new list input and submission form. The v-model
directive allows binding input values to Vue components. Using the v-model
directive is actually how we generally use text input boxes, and it will automatically update the two-way data binding.
In order to add a new list item, we need to display a form and collect new data from the form. We use Vue.js to create the form as follows:
<template> <div> <form @submit.prevent="addItem"> <input type="text" v-model="newItem" placeholder="Add a new item"> <button type="submit">Add</button> </form> </div> </template>
We use the @submit.prevent
event modifier on the form so that the form is never actually submitted. Instead, it triggers the event handler we bound to the addItem
method. This method will add a new item to the data array.
Herev-model
How is the instruction bound to the input reserved text box? We need to define a new variable newItem
in the data model of the Vue component, as follows:
const app = Vue.createApp({ data() { return { items: [ { id: 1, name: 'Item 1' }, { id: 2, name: 'Item 2' }, { id: 3, name: 'Item 3' }, { id: 4, name: 'Item 4' }, { id: 5, name: 'Item 5' } ], newItem: '' } }, methods: { addItem() { this.items.push({id: this.items.length + 1, name: this.newItem}); this.newItem = ''; } } });
In the addItem
method, we insert it at the end of the array A new project object and give the new project object a unique ID. After adding the item, we will reset newItem
with an empty string. Now we have implemented the functionality of adding new items to an array.
4. Edit entries
Our current goal is to add an edit button to each item. When the button is clicked, the name of the item can be modified. This editing functionality is achieved by binding v-model
to the current item in the data model and displaying a save button. You can put an edit button next to each item that switches to edit mode when clicked.
First, we need to define a currentItem
variable. This variable will specify the item currently being edited. We also need to define the editing
variable to hide the display of the entry name in editing mode like this:
const app = Vue.createApp({ data() { return { items: [ { id: 1, name: 'Item 1' }, { id: 2, name: 'Item 2' }, { id: 3, name: 'Item 3' }, { id: 4, name: 'Item 4' }, { id: 5, name: 'Item 5' } ], newItem: '', editing: false, currentItem: null } }, methods: { addItem() { this.items.push({id: this.items.length + 1, name: this.newItem}); this.newItem = ''; }, editItem(item) { this.editing = true; this.currentItem = item; }, saveItem() { this.editing = false; this.currentItem = null; } } });
Now we need to show the edit button in every item element. When the edit button is clicked, we need to switch to edit mode so we can start editing the current project. We can set edit buttons for each item using the following code:
<template> <ul> <li v-for="item in items" :key="item.id"> <span v-if="!editing || currentItem !== item">{{ item.name }}</span> <span v-else><input type="text" v-model="currentItem.name"></span> <button @click="editItem(item)" v-if="!editing">Edit</button> <button @click="saveItem()" v-if="editing">Save</button> </li> </ul> </template>
We toggle the fallback text element and edit text input box to show or hide items by using the v-if
directive The name. When we use edit mode, enter or edit the project name in the edit text box. Edit mode can be exited by clicking the Save button.
5. Delete items
We now need to add a function to delete items. We need to add a delete button for each item. When the delete button is clicked, we will delete this item from the data array. We will use JavaScript's Array.prototype.indexOf() method to find and delete items.
const app = Vue.createApp({ data() { return { items: [ { id: 1, name: 'Item 1' }, { id: 2, name: 'Item 2' }, { id: 3, name: 'Item 3' }, { id: 4, name: 'Item 4' }, { id: 5, name: 'Item 5' } ], newItem: '', editing: false, currentItem: null } }, methods: { addItem() { this.items.push({id: this.items.length + 1, name: this.newItem}); this.newItem = ''; }, editItem(item) { this.editing = true; this.currentItem = item; }, saveItem() { this.editing = false; this.currentItem = null; }, deleteItem(item) { const index = this.items.indexOf(item); if (index > -1) { this.items.splice(index, 1); } } } });
Now we can add a delete button to each item. When the delete button is clicked, we can call the deleteItem() method.
<template> <ul> <li v-for="item in items" :key="item.id"> <span v-if="!editing || currentItem !== item">{{ item.name }}</span> <span v-else><input type="text" v-model="currentItem.name"></span> <button @click="editItem(item)" v-if="!editing">Edit</button> <button @click="saveItem()" v-if="editing">Save</button> <button @click="deleteItem(item)">Delete</button> </li> </ul> <div> <form @submit.prevent="addItem"> <input type="text" v-model="newItem" placeholder="Add a new item"> <button type="submit">Add</button> </form> </div> </template>
We have learned how to use Vue.js to add, delete, modify and check the list page. Vue's simplicity and ease of use make it a very powerful choice to easily handle most operations for list applications.
Vue not only makes code easier to understand, but also allows us to quickly create powerful web applications. Creating a list page for any project has become easier with the help of Vue.js.
The above is the detailed content of Add, delete, modify and check vue list page. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics

React combines JSX and HTML to improve user experience. 1) JSX embeds HTML to make development more intuitive. 2) The virtual DOM mechanism optimizes performance and reduces DOM operations. 3) Component-based management UI to improve maintainability. 4) State management and event processing enhance interactivity.
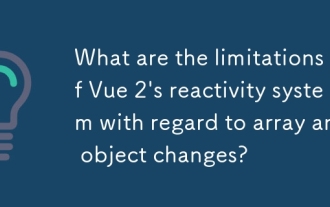
Vue 2's reactivity system struggles with direct array index setting, length modification, and object property addition/deletion. Developers can use Vue's mutation methods and Vue.set() to ensure reactivity.

React components can be defined by functions or classes, encapsulating UI logic and accepting input data through props. 1) Define components: Use functions or classes to return React elements. 2) Rendering component: React calls render method or executes function component. 3) Multiplexing components: pass data through props to build a complex UI. The lifecycle approach of components allows logic to be executed at different stages, improving development efficiency and code maintainability.

React is the preferred tool for building interactive front-end experiences. 1) React simplifies UI development through componentization and virtual DOM. 2) Components are divided into function components and class components. Function components are simpler and class components provide more life cycle methods. 3) The working principle of React relies on virtual DOM and reconciliation algorithm to improve performance. 4) State management uses useState or this.state, and life cycle methods such as componentDidMount are used for specific logic. 5) Basic usage includes creating components and managing state, and advanced usage involves custom hooks and performance optimization. 6) Common errors include improper status updates and performance issues, debugging skills include using ReactDevTools and Excellent
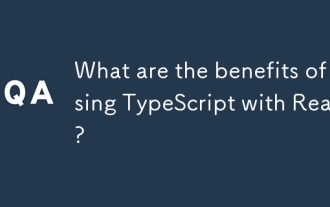
TypeScript enhances React development by providing type safety, improving code quality, and offering better IDE support, thus reducing errors and improving maintainability.
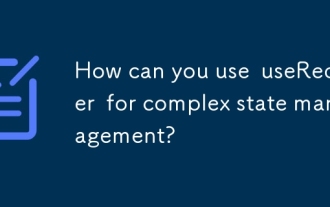
The article explains using useReducer for complex state management in React, detailing its benefits over useState and how to integrate it with useEffect for side effects.
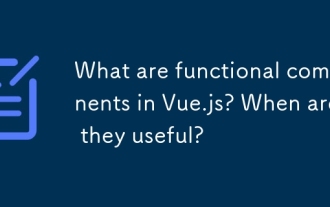
Functional components in Vue.js are stateless, lightweight, and lack lifecycle hooks, ideal for rendering pure data and optimizing performance. They differ from stateful components by not having state or reactivity, using render functions directly, a

React is a JavaScript library for building user interfaces, with its core components and state management. 1) Simplify UI development through componentization and state management. 2) The working principle includes reconciliation and rendering, and optimization can be implemented through React.memo and useMemo. 3) The basic usage is to create and render components, and the advanced usage includes using Hooks and ContextAPI. 4) Common errors such as improper status update, you can use ReactDevTools to debug. 5) Performance optimization includes using React.memo, virtualization lists and CodeSplitting, and keeping code readable and maintainable is best practice.
