Using Ajax to implement asynchronous requests in PHP
In modern Web application development, Ajax has become a very important technology. Through Ajax, asynchronous requests can be sent to the server without refreshing the page, achieving the effects of partial refresh and dynamic loading of data. As one of the most popular web development languages at present, PHP also provides a wealth of functions and class libraries to support the use of Ajax. This article will introduce how to use Ajax in PHP to implement asynchronous requests.
1. What is Ajax
The full name of Ajax is Asynchronous Javascript And XML, which is asynchronous JavaScript and XML. It is a technology that implements asynchronous communication between the client and the server, and realizes data transmission and interaction through the XMLHttpRequest object. It can make web applications more flexible and interactive, able to update part of the content without refreshing the entire page, reducing user waiting time and server pressure. Ajax is essentially a combination of technologies, including HTML, CSS, JavaScript, XML and other technologies. As a server-side scripting language, PHP can cooperate with Ajax code to achieve dynamic effects of web applications and improve user experience.
2. Use Ajax to make asynchronous requests
Making Ajax asynchronous requests in PHP generally requires the following steps:
- Create an XMLHttpRequest object
In JavaScript, we can implement Ajax asynchronous requests by creating an XMLHttpRequest object. The specific code is as follows:
var xmlhttp; if (window.XMLHttpRequest) { xmlhttp = new XMLHttpRequest(); } else { // 兼容早期版本的IE浏览器 xmlhttp = new ActiveXObject("Microsoft.XMLHTTP"); }
- Send a request
After creating the XMLHttpRequest After the object, we need to send a request through it. Generally, we will send a GET request or a POST request. The specific code is as follows:
xmlhttp.open("GET","demo.php",true); xmlhttp.send();
Here, we use the open() method to specify the request type and request URL. and whether to send the request asynchronously (true means sending the request asynchronously), and then use the send() method to send the request.
- Receive response
When the request is sent, the XMLHttpRequest object will automatically trigger an onreadystatechange event. We can obtain the data returned by the server by listening to this event, and Carry out corresponding processing.
xmlhttp.onreadystatechange=function() { if (xmlhttp.readyState==4 && xmlhttp.status==200) { // 接收到服务器返回的数据,进行相应的处理 document.getElementById("demo").innerHTML=xmlhttp.responseText; } }
Here, we first detect the current state of the XMLHttpRequest object through the readyState attribute. When readyState is 4, it means that the server has correctly processed our request and returned a response. Next, we use the status attribute to detect whether the HTTP status code returned by the server is 200. 200 indicates that the request was successful, and other status codes indicate that there are some problems with the request. Finally, we can obtain the data returned by the server through the responseText attribute and process it accordingly.
3. Use jQuery to encapsulate Ajax requests
If you want to make Ajax asynchronous requests more conveniently and quickly, you can use the Ajax function provided by the jQuery framework. jQuery's Ajax function encapsulates and optimizes the XMLHttpRequest object, allowing us to make asynchronous requests more simply, reducing the amount of code and the possibility of errors.
The following is an example of a jQuery Ajax request:
$.ajax({ type: "POST", // 请求方式,可以是"GET"或"POST" url: "demo.php", // 请求的URL地址 data: {"name": "Jack", "age": 25}, // 发送到服务器的数据 dataType: "json", // 服务器返回的数据类型 success: function(data){ // 请求成功,获取到服务器返回的数据,并进行相应的处理 console.log(data.name); }, error: function(XMLHttpRequest, textStatus, errorThrown){ // 请求失败,输出错误信息 console.log(textStatus); } });
In this example, we use the $.ajax() function to send an asynchronous request, which receives a JavaScript object as a parameter. Configuration request. Among them, the type attribute specifies the request type, which can be GET or POST; the url attribute specifies the URL address of the request; the data attribute specifies the data sent to the server; the dataType attribute specifies the data type returned by the server, we can specify "json", "xml" ", "html" and other formats; the success attribute specifies the callback function when the request is successful. This function will be called after the server responds successfully. We can get the data returned by the server here and process it accordingly; the error attribute specifies the request Callback function on failure. This function will be called after the server response fails. We can output relevant error information here to facilitate debugging and troubleshooting.
Summary
Through the above introduction, we can find that using Ajax to implement asynchronous requests in PHP is very convenient and flexible. Whether it is encapsulated based on native JavaScript or jQuery, it can be implemented for Asynchronous request functions for different business scenarios. In the process of using Ajax, we need to pay attention to mastering the basic Ajax technical principles and related request parameters so that they can be used flexibly in actual scenarios.
The above is the detailed content of Using Ajax to implement asynchronous requests in PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


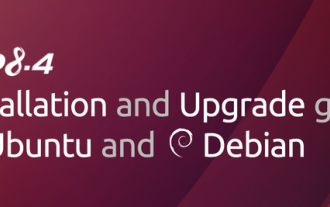
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
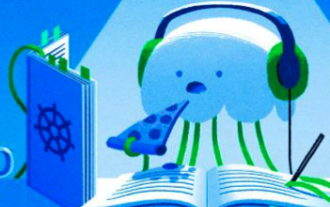
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
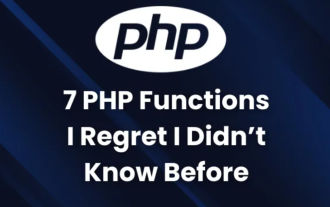
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
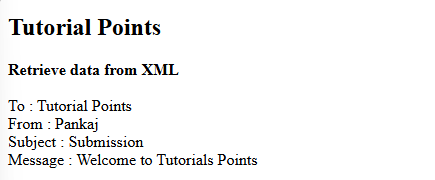
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
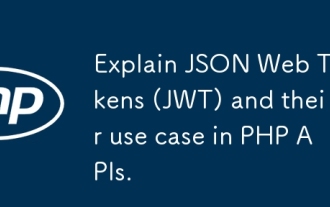
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
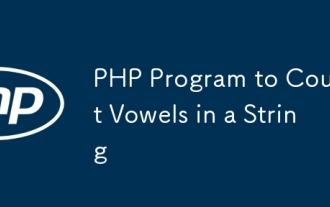
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
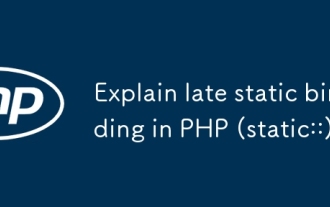
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
