How to use audio processing in Go?
With the popularity of audio processing in various application scenarios, more and more programmers are beginning to use Go to write audio processing programs. As a modern programming language, Go language has excellent concurrency and high efficiency characteristics. It is very convenient to use it for audio processing. This article will introduce how to use audio processing technology in Go, including reading, writing, processing and analyzing audio data.
1. Reading audio data
There are many ways to read audio data in Go. One of the more commonly used is to use third-party libraries for reading, such as go-sox and go-wave. The following takes the go-sox library as an example to introduce how to read audio data in Go.
First you need to install the go-sox library. You can install it using the following command:
go get github.com/krig/go-sox
Next, you can use the following code to read a wav file:
package main import ( "log" "github.com/krig/go-sox" ) func main() { // Open the input file input, err := sox.OpenRead("input_file.wav") if err != nil { log.Fatalf("Failed to open input file: %v", err) } defer input.Release() // Read the input file into a buffer buffer, err := input.Read(nil) if err != nil { log.Fatalf("Failed to read input file: %v", err) } }
In this example, the OpenRead
function opens a wav file and use it as an input file. If an error occurs, the corresponding error message will be returned. Read
The function reads audio file data, and the read data is returned in the form of a buffer.
2. Writing audio data
Similar to reading audio data, you can also use some third-party libraries to write audio data in Go. For example, the go-wave library can easily read and write wav files. The following takes the go-wave library as an example to introduce how to write audio data in Go.
First you need to install the go-wave library. It can be installed using the following command:
go get github.com/gerow/go-wave
Next, the audio data can be written to a wav file using the following code:
package main import ( "log" "github.com/gerow/go-wave" ) func main() { // Create a wave file w, err := wave.Create("output_file.wav") if err != nil { log.Fatalf("Failed to create output file: %v", err) } defer w.Close() // Get the audio format format := wave.Format{ Channels: 1, SampleRate: 44100, SignificantBits: 16, ByteRate: 88200, BlockAlign: 2, } // Set the audio format err = w.SetFormat(format) if err != nil { log.Fatalf("Failed to set format: %v", err) } // Write the audio data data := make([]byte, 1024) for i := range data { data[i] = 0xff } _, err = w.Write(data) if err != nil { log.Fatalf("Failed to write audio data: %v", err) } }
In this example, we use Create
The function creates a wav file and sets it as the output file. If there is an error, the corresponding error message will be returned. Use the SetFormat
function to set the audio format. Use the Write
function to write audio data.
3. Processing audio data
For the processing of audio data, Go language provides many libraries, such as go-portaudio and goaudio. Let's take the goaudio library as an example to introduce how to process audio data in Go.
First you need to install the goaudio library. You can install it using the following command:
go get github.com/cryptix/goaudio
Next, you can use the following code to process the audio data:
package main import ( "fmt" "math" "github.com/cryptix/goaudio/snd" "github.com/cryptix/goaudio/sndfile" ) func main() { filename := "input_file.wav" // Open the file for reading sf, err := sndfile.Open(filename, sndfile.Read, nil) if err != nil { panic(err) } defer sf.Close() // Get the number of frames and channels frameCount := sf.Samples channelCount := sf.Channels // Create a buffer to hold the samples buffer := make([]float64, frameCount*channelCount) // Read the samples into the buffer if err := snd.ReadInto(sf, buffer); err != nil { panic(err) } // Apply a sine wave to the samples for i := 0; i < len(buffer); i += channelCount { sample := buffer[i] angle := float64(i) * 2.0 * math.Pi / float64(sf.SampleRate) buffer[i] = sample * math.Sin(angle) } // Create a new file for writing newFilename := "output_file.wav" newSf, err := sndfile.Open(newFilename, sndfile.Write, &sf.Info) if err != nil { panic(err) } defer newSf.Close() // Write the modified samples if err := snd.Write(newSf, buffer); err != nil { panic(err) } fmt.Printf("Done") }
In this example, we open a wav file and read it into a buffer. Then a simple process was performed on the samples in the buffer: a sine wave was applied to the audio data. After that, we wrote the processed audio data into a new wav file.
4. Analyze audio data
There are many libraries for audio analysis in Go language, such as go-dsp and gonum. The following is an introduction using the go-dsp library.
First you need to install the go-dsp library. You can use the following command to install:
go get github.com/mjibson/go-dsp
Next, you can use the following code to obtain the audio recording data and analyze it:
package main import ( "fmt" "os" "time" "github.com/Twister915/go-dsp/wavio" ) func main() { filename := "recording.wav" // Open the wave file f, err := os.Open(filename) if err != nil { panic(err) } defer f.Close() // Parse the wave file w, err := wavio.Read(f) if err != nil { panic(err) } // Print the sample rate, duration, and length of the data fmt.Printf("Sample rate: %d Duration: %s Data length: %d ", w.Original.SampleRate, time.Duration(float64(w.Len()) / float64(w.Original.ByteRate) * float64(time.Second)), w.Len()) // Analyze the data var sum float64 var max float64 for _, s := range w.Data { sum += float64(s) if float64(s) > max { max = float64(s) } } average := sum / float64(len(w.Data)) fmt.Printf("Peak amplitude: %f ", max) fmt.Printf("Average amplitude: %f ", average) }
In this example, we open A wav file and analyzed the sampled data. The maximum and average values of the audio data were calculated respectively. This process can help us better understand the audio data we are processing so that we can formulate corresponding processing strategies.
Summary
This article mainly introduces how to use audio processing technology in Go, including reading, writing, processing and analyzing audio data. Audio processing is a vast field, and this article only covers some basic techniques. With this basic knowledge, readers can have an in-depth understanding of more complex audio processing technologies and develop and implement them in the Go language.
The above is the detailed content of How to use audio processing in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


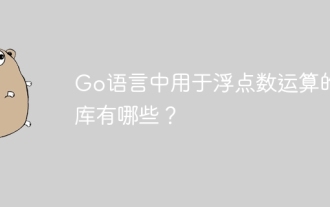
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
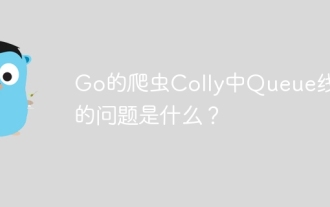
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
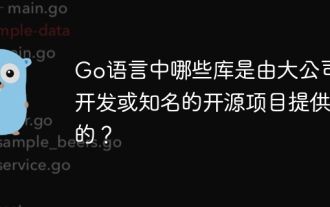
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
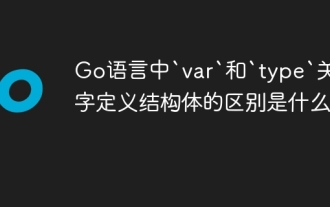
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
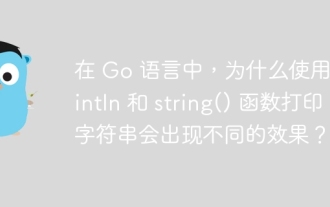
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
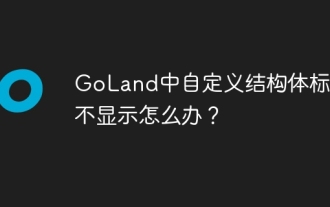
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
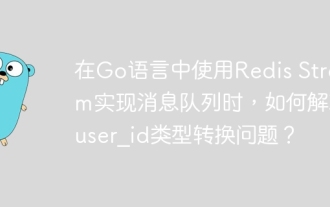
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
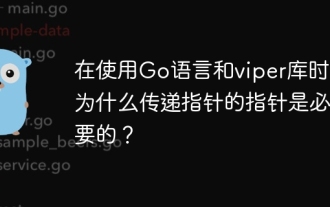
Go pointer syntax and addressing problems in the use of viper library When programming in Go language, it is crucial to understand the syntax and usage of pointers, especially in...
