How to use JSON in Go?
JSON (JavaScript Object Notation) is a lightweight data exchange format that is often used to send data from the server to the client, and to pass data between different systems. In the Go language, using JSON is also very simple. This article will introduce how to use Go to process JSON data and provide readers with best practices when using JSON in Go.
There is a built-in JSON package in the Go language that can marshal or unmarshal JSON data into Go values, similar to the json module in Python or JSON objects in JavaScript. Working with JSON in Go is divided into two important steps: marshalling and unmarshalling.
Marshalling JSON
Malesling JSON means converting a Go object into JSON format so that it can be transmitted over the network. In Go, use the Marshal() method to marshal JSON.
For example, if we have a struct called Person:
type Person struct { Name string `json:"name"` Age int `json:"age"` }
We can use json.Marshal() to marshal it into JSON format:
p := Person{Name: "Tom", Age: 27} jsonData, err := json.Marshal(p) if err != nil { log.Println("Error in marshalling JSON") } else { log.Println(string(jsonData)) }
above In the example, we create a Person object named p, call the json.Marshal() method to marshal it into a JSON string, and then print the JSON string. The output should be as follows:
{"name":"Tom","age":27}
As you can see, the output string contains the key-value pairs corresponding to the Person structure. Note that if your structure has private fields, these fields will not be marshaled into JSON. Public fields should be used when marshalling. Additionally, when marshalling using the Marshal() method, you should check for errors if the marshaling fails. If there are no errors, the marshalled JSON string is returned.
Unmarshaling JSON
Unmarshalling JSON means parsing JSON data into Go objects. In Go, use the Unmarshal() method to unmarshal JSON.
For example, if we have a struct called Person:
type Person struct { Name string `json:"name"` Age int `json:"age"` }
We can use json.Unmarshal() to unmarshal it:
var person Person jsonString := `{"name":"Tom","age":27}` err := json.Unmarshal([]byte(jsonString), &person) if err != nil { log.Println("Error in unmarshalling JSON") } else { log.Println(person.Name) log.Println(person.Age) }
In the above example , we pass the JSON string of the Person object to the json.Unmarshal() method and use &person to store the unmarshaled value in the person object. Note that when unmarshalling, we should use the same name as the field in the structure to decode the JSON key to avoid decoding failure. If an error occurred while decoding the JSON, an error message is returned, otherwise the decoded JSON value is stored in the person object.
Best Practices
When using JSON in Go, follow these practices:
- Handle errors properly
When using JSON in Go When an error occurs while marshalling or unmarshalling JSON, the error should be handled appropriately and the user should be notified in an appropriate manner. For example, we can write error logs to a log file or return error messages to the user.
- Using JSON tags
You can use json tags to add member tags to struct fields to marshal Go object data into the expected JSON format. The json tag usually contains JSON segment keys to allow developers to specify their property names when marshalling.
- Avoid using empty structures
Use empty structures in Go to represent data without content. When marshalling JSON data, do not use empty structures, use nil instead. If you use an empty structure, the marshalled JSON may be too verbose.
- Explicit Type Assertion
When unmarshalling JSON data, always use explicit type assertions to ensure that the type of the data matches the type in Go. If you want to handle multiple JSON types, use type switch statements to avoid unnecessary errors.
- Use third-party libraries
If you need other advanced features, you should use the available third-party JSON libraries. Some commonly used third-party JSON libraries include json-iterator/go, ghodss/yaml, easyjson, etc.
Summary
In this article, we have introduced the basic operations of using JSON in Go language, including marshalling and unmarshaling JSON data. Following best practices ensures your code is readable, maintainable, and extensible. Using these practices, you will be able to take advantage of JSON capabilities in Go and become better at writing JSON-related code.
The above is the detailed content of How to use JSON in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


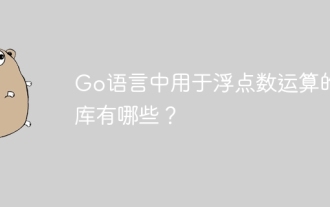
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
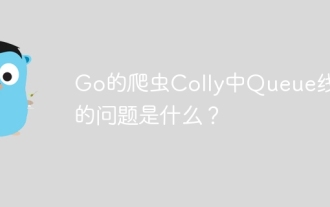
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
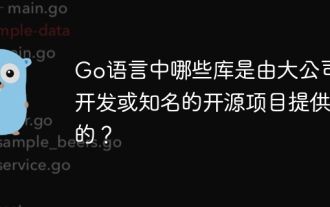
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
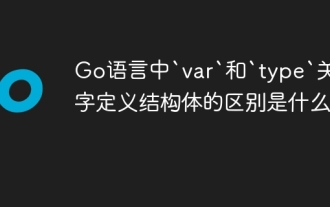
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
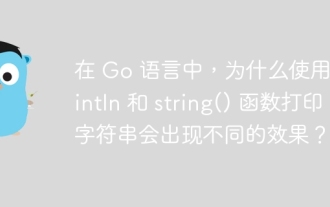
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
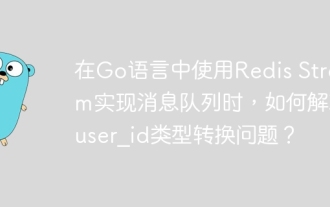
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
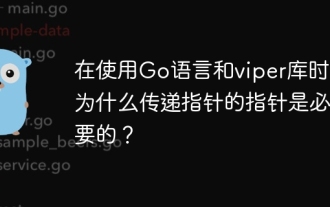
Go pointer syntax and addressing problems in the use of viper library When programming in Go language, it is crucial to understand the syntax and usage of pointers, especially in...
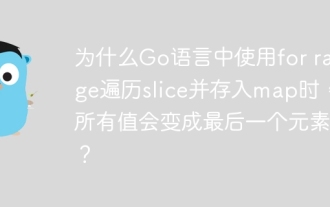
Why does map iteration in Go cause all values to become the last element? In Go language, when faced with some interview questions, you often encounter maps...
