Basic process of building big data applications using PHP
In recent years, with the explosive growth of data volume, the demand for big data applications is increasing. As a popular programming language, PHP is widely used in web development and can also be used to build big data applications.
This article will introduce the basic process of using PHP to build big data applications, including data processing, storage and analysis.
1. Data processing
Data processing is the first step in big data application. Its purpose is to collect data from various sources and perform preliminary processing and cleaning for storage and analysis. . PHP can collect data in various ways, such as through APIs, crawlers, etc.
1.1 Use third-party API to collect data
Most websites provide API interfaces through which data can be obtained. Building an API client using PHP is very simple. You can use curl or the file_get_contents function to request the API, and use the json_decode function to convert the response into a PHP array.
For example, you can use the API interface provided by GitHub to obtain the user's warehouse information:
$username = 'Your_GitHub_Username'; $url = "https://api.github.com/users/{$username}/repos"; $response = file_get_contents($url); // 将JSON响应转换为数组 $repos = json_decode($response, true);
1.2 Use a crawler to collect data
If you cannot obtain the API interface, you can also use a crawler Technology collects data. PHP provides multiple crawler frameworks, such as Goutte and Symfony DomCrawler. Using these frameworks you can easily extract the required data from the target website.
For example, you can use Goutte to collect free book data:
require_once 'vendor/autoload.php'; // 创建一个新的Goutte对象 $goutte = new GoutteClient(); // 访问目标网页并获取HTML $crawler = $goutte->request('GET', 'http://www.gutenberg.org/ebooks/search/?query=free+books'); // 查找所有书籍链接 $links = $crawler->filter('.booklink a')->links(); foreach ($links as $link) { // 访问每个链接并获取书籍标题 $crawler = $goutte->click($link); $title = $crawler->filter('.biblio h1')->text(); // 保存数据到数据库或文件 echo "Title: {$title} "; }
2. Data storage
The processed data needs to be stored in a database or file for subsequent analysis. . For big data applications, you need to choose an efficient storage method, such as a NoSQL database or a distributed file system.
2.1 Using MongoDB to store data
MongoDB is a popular NoSQL database that supports high scalability and performance. PHP provides a MongoDB extension that can use MongoDB for data storage.
For example, you can use MongoDB to store GitHub warehouse data:
// 连接到MongoDB服务器 $client = new MongoDBClient('mongodb://localhost:27017'); // 获取数据库和集合对象 $database = $client->selectDatabase('my_database'); $collection = $database->selectCollection('my_collection'); // 插入数据 $collection->insertMany($repos);
2.2 Use Hadoop distributed file system to store data
Hadoop is a popular distributed file system that can support Large-scale data storage and analysis. PHP provides the PHP-Hadoop extension, which can use Hadoop for data storage.
For example, Hadoop can be used to store free book data collected by crawlers:
// 连接到Hadoop文件系统 $conf = new HadoopConfiguration(); $conf->set('fs.defaultFS', 'hdfs://localhost:9000'); $fs = HadoopFilesystemFileSystem::createFromConfiguration($conf); // 创建目录 $fs->mkdir('/books'); // 存储数据 $filename = '/books/free_books.txt'; $file = $fs->create($filename); $file->write("Title: {$title} "); $file->close();
3. Data analysis
After the data is stored, the data needs to be statistically and analyzed in order to Understand the characteristics and trends of the data. PHP provides a variety of data analysis tools, such as the PHP extension php-r of the R language, and the MapReduce framework based on Hadoop.
3.1 Use php-r for data analysis
php-r is a PHP extension that allows PHP to use the functions of the R language for data analysis. Using php-r, you can easily perform data visualization, distributed computing and other operations.
For example, you can use php-r to visualize GitHub warehouse data:
// 连接到R语言进程 $r = new PHPRServeEngineRserve(); // 加载R包 $ggplot = $r->evaluate('library(ggplot2)'); // 创建数据框 $dataFrame = $r->dataFrame($repos); // 生成散点图 $plot = $r->plot("ggplot({$dataFrame}, aes(x=language, y=stargazers_count)) + geom_point()"); // 输出图片 echo $plot->getImageDataUri();
3.2 Use MapReduce for data analysis
MapReduce is a distributed computing framework that can be used in Hadoop etc. to run on the big data platform. MapReduce can automatically divide work into multiple steps and distribute these steps for execution on different computers.
For example, you can use Hadoop's MapReduce framework to count website visits in a certain region:
// 定义Map函数 function mapFunction($url, $count) { $domain = parse_url($url, PHP_URL_HOST); yield $domain => $count; } // 定义Reduce函数 function reduceFunction($key, $values) { yield $key => array_sum($values); } // 创建MapReduce任务 $job = new HadoopJobMapReduceJob(); $job->setMapper('mapFunction'); $job->setReducer('reduceFunction'); $job->setInput('/logs/access.log'); $job->setOutput('/logs/access.out'); // 提交任务并等待结果 $result = $job->submitAndWait();
Summary
The basic process of using PHP to build big data applications includes data processing and storage and analyze three aspects. In terms of data processing, you can use third-party APIs and crawler technology to collect data; in terms of data storage, you can choose NoSQL databases or distributed file systems; in terms of data analysis, you can use php-r for data visualization and MapReduce for distributed computing. . With the continuous development of database and distributed computing technology, the way of building big data applications using PHP is also constantly evolving.
The above is the detailed content of Basic process of building big data applications using PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


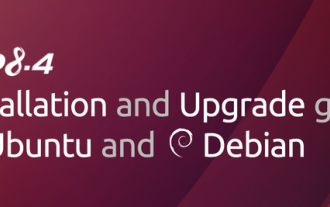
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
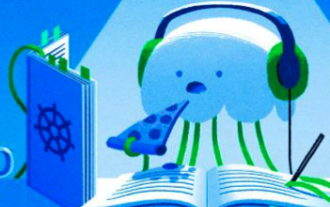
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
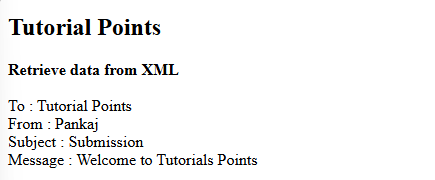
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
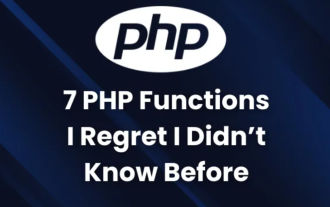
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
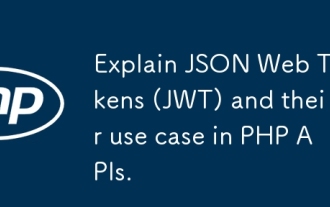
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
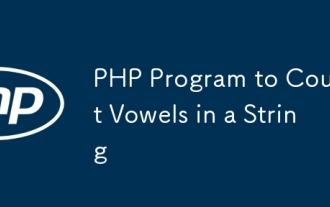
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
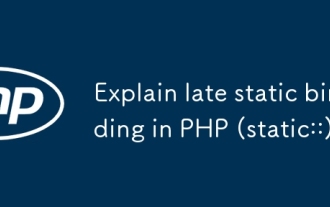
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
