nodejs blob to file
In front-end development, we may encounter the need to convert Blob objects into files. Blob is an object type in Web API that can represent arbitrary binary data. A file is an entity with attributes such as file name, file type, file size, etc. We often need to convert the Blob object into a file type through the browser for uploading, saving and other operations.
In the Node.js environment, we can use Node's built-in module fs to perform file operations, and we can use Buffer to process binary data. Therefore, we can convert the Blob object into a file by converting the Blob object to a Buffer, and then use the fs module to write the Buffer to the file.
The specific implementation steps are as follows:
- Get the Blob object. We can get the Blob object through the following code:
let blob = new Blob([arrayBuffer], { type: 'image/png' });
Here a Blob object containing binary data is created.
- Convert Blob to Buffer. We can convert Blob objects into Buffers with the help of the buffer module in Node.js. The specific code is as follows:
const buffer = Buffer.from(await blob.arrayBuffer());
The arrayBuffer() method of Blob is used to obtain binary data and then convert it into a Buffer.
- Write to file. Using the fs module in Node.js, we can write the Buffer to a file. The specific code is as follows:
fs.writeFile('test.png', buffer, (err) => { if (err) throw err; console.log('The file has been saved!'); });
Here the Buffer is written to a file named "test.png". If written If there is an error in the file, an exception will be thrown, otherwise "The file has been saved!" will be output on the console.
The complete code is as follows:
const fs = require('fs'); const fetch = require('node-fetch'); async function downloadFile(url) { const res = await fetch(url); const blob = await res.blob(); const buffer = Buffer.from(await blob.arrayBuffer()); fs.writeFile('test.png', buffer, (err) => { if (err) throw err; console.log('The file has been saved!'); }); } downloadFile('https://www.example.com/test.png');
This code can download the image file on the remote server to the local and save it as the file "test.png".
Summary:
This article introduces how to convert Blob objects into files. In the Node.js environment, we can use the Buffer and fs modules to achieve this function. This method is very useful when we need to convert the Blob object in the front end to a file, or when we need to process binary data and save it as a file in the Node.js environment.
The above is the detailed content of nodejs blob to file. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
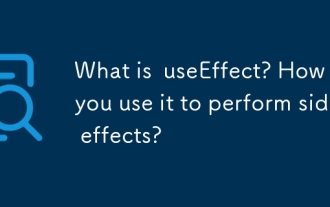
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.

Lazy loading delays loading of content until needed, improving web performance and user experience by reducing initial load times and server load.

Higher-order functions in JavaScript enhance code conciseness, reusability, modularity, and performance through abstraction, common patterns, and optimization techniques.
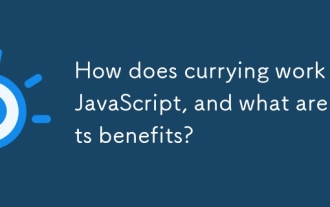
The article discusses currying in JavaScript, a technique transforming multi-argument functions into single-argument function sequences. It explores currying's implementation, benefits like partial application, and practical uses, enhancing code read
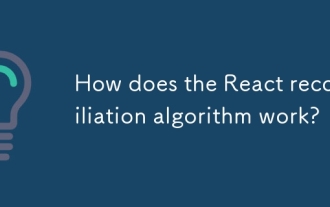
The article explains React's reconciliation algorithm, which efficiently updates the DOM by comparing Virtual DOM trees. It discusses performance benefits, optimization techniques, and impacts on user experience.Character count: 159
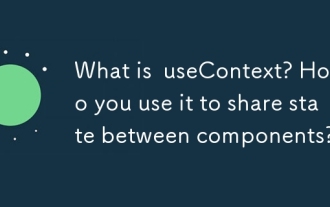
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
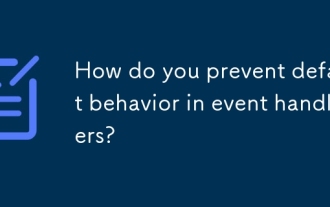
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.
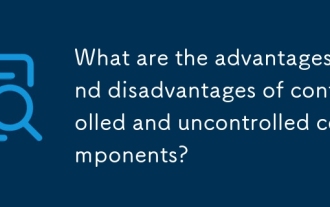
The article discusses the advantages and disadvantages of controlled and uncontrolled components in React, focusing on aspects like predictability, performance, and use cases. It advises on factors to consider when choosing between them.
