Anonymous functions and closures in PHP
In PHP, an anonymous function is a function defined at runtime without a specified function name. Anonymous functions can be assigned to variables, passed as arguments to other functions, or returned as the return value of other functions. A closure is an anonymous function that contains external scope variables that can be used or modified inside the function. This mechanism makes functions in PHP more flexible and powerful.
The basic syntax of anonymous functions is as follows:
$functionName = function($arg1, $arg2, ...) { // function body };
In this syntax, $functionName is a pointer variable pointing to the anonymous function. We can call the anonymous function through this variable just like calling a normal function. $arg1, $arg2, ... represent the parameters of the function. Inside the function body, we can use these parameters for calculation and processing, and finally return the required results. For example, the following code defines an anonymous function add that calculates the sum of two numbers and returns the result:
$add = function($a, $b) { return $a + $b; }; echo $add(2, 3); // 输出5
Anonymous functions can be passed as arguments to other functions. For example, we can use the array_map function to add 1 to the elements in an array:
$arr = [1, 2, 3]; $newArr = array_map(function($item) { return $item + 1; }, $arr); print_r($newArr); // 输出 [2, 3, 4]
In this example, we use the array_map function to execute an anonymous function on each element in the $arr array. The anonymous function adds 1 to the array element and returns it, ultimately generating a new array $newArr.
A closure is an anonymous function that can reference variables in the outer scope. These variables can be passed as parameters to the closure, or they can be used directly inside the closure and are called "closure variables".
For example, the following code defines a closure function that accumulates the passed parameters and saves the result in the closure variable $count:
$sum = function($num) use(&$count) { $count += $num; }; $count = 0; $sum(1); $sum(2); echo $count; // 输出3
In this example, We define a closure function $sum and internally reference the variable $count in the outer scope. In the closure function, we accumulate the passed parameter $num and save the result in $count. Since the $count variable is defined outside the function, we need to reference it using a use statement and pass it in as a parameter of the closure function.
When using closure functions, you need to note that the life cycle of the closure variable will exist along with the life cycle of the closure function, which may cause some problems. For example, in the following code, we define an array $funcs and execute each function by traversing the array:
$funcs = []; for($i = 0; $i < 5; $i++) { $funcs[$i] = function() use($i) { return $i; }; } foreach($funcs as $func) { echo $func(); }
The expected output result should be "01234", but the actual output result is "44444" . This is because there is only one variable $i referenced in the closure function, and all functions defined in the $funcs array reference the same variable, and ultimately return the final value of $i, 4. To avoid this, we can use a different variable name instead of $i. For example, we can use variable $j to replace $i to solve this problem:
$funcs = []; for($i = 0; $i < 5; $i++) { $j = $i; $funcs[$i] = function() use($j) { return $j; }; } foreach($funcs as $func) { echo $func(); }
The output result this time is the expected "01234". We used $j to replace the variable $i referenced in the closure function, so that each closure function has its own independent closure variable.
In short, anonymous functions and closures make functions in PHP more flexible and versatile. Using anonymous functions and closures, we can define and use functions more conveniently and implement more complex functions and algorithms. At the same time, we also need to pay attention to the life cycle and scope of closure variables to avoid unexpected results.
The above is the detailed content of Anonymous functions and closures in PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


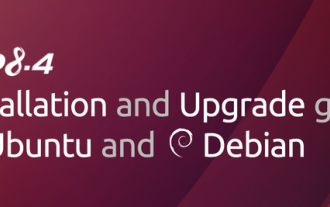
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
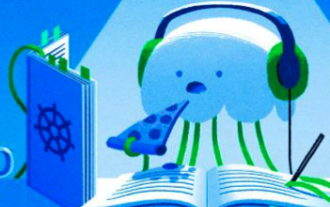
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
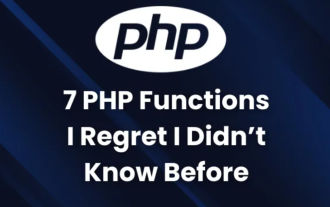
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
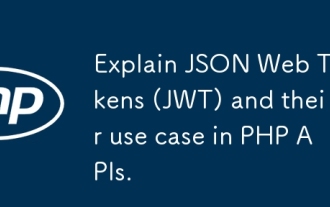
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
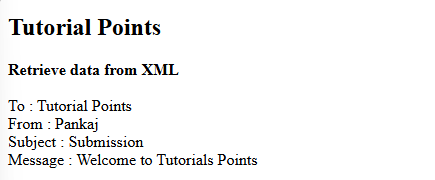
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
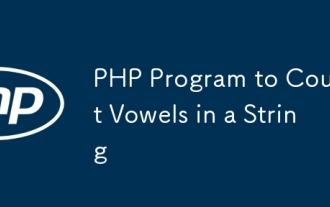
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
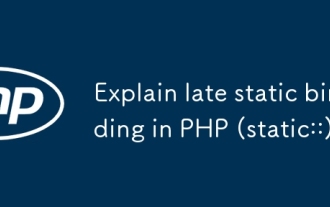
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
