nodejs file request
Node.js File Request
Node.js is a JavaScript running environment based on the Chrome V8 engine. It enables JavaScript to run on the server side and process files, network requests and other operations to achieve high efficiency and high performance. back-end application.
In Node.js, we can use the module system to introduce other JavaScript files. For example, require('fs') can introduce the file system module of Node.js, through which we can read and write Import the file. In this article, we'll cover how to make file requests in Node.js.
Use Node.js to initiate file requests
In Node.js, we can use the http module to initiate http requests. The process of using the http module is generally as follows:
- Introduce the http module
Use require('http') to introduce the http module of Node.js.
const http = require('http');
- Create Server
Use the http.createServer() method to create a server. This method accepts a callback function that will be called when a request is made.
const server = http.createServer((req, res) => { // ... });
- Handle the request
Handle the request in the callback function. We can get the requested information, request parameters, etc. In this article, we need to get the path to the requested file.
const url = req.url; // 获取请求的路径
- Initiate a file request
In the callback function that handles the request, we can use the fs module to read the file content and send the content to the client.
const fs = require('fs'); fs.readFile('file_path', (err, data) => { if (err) throw err; res.write(data.toString()); // 发送数据给客户端 res.end(); // 结束响应 });
Complete sample code:
const http = require('http'); const fs = require('fs'); const server = http.createServer((req, res) => { const url = req.url; fs.readFile('file_path', (err, data) => { if (err) throw err; res.write(data.toString()); res.end(); }); }); server.listen(8080);
In the above code, we listen to a local port (8080), and when there is a request, read the file content and send it to the client. Note that we need to replace file_path with the actual file path.
Summary
In Node.js, we can use the http module to initiate http requests, and the fs module to read and write files. Using the above method, we can initiate file requests in Node.js. In this way, we can directly send the file content to the client on the backend, so that the client can load the file faster and improve the page response speed.
The above is the detailed content of nodejs file request. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
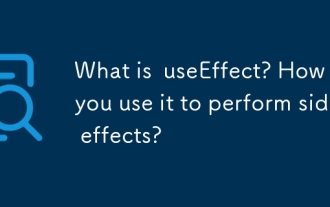
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.

Lazy loading delays loading of content until needed, improving web performance and user experience by reducing initial load times and server load.

Higher-order functions in JavaScript enhance code conciseness, reusability, modularity, and performance through abstraction, common patterns, and optimization techniques.
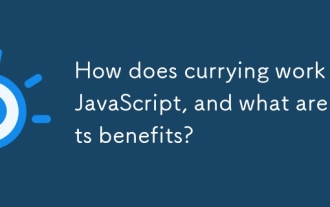
The article discusses currying in JavaScript, a technique transforming multi-argument functions into single-argument function sequences. It explores currying's implementation, benefits like partial application, and practical uses, enhancing code read
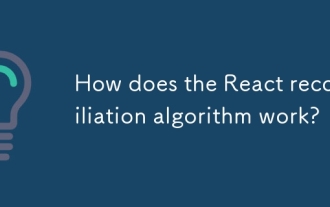
The article explains React's reconciliation algorithm, which efficiently updates the DOM by comparing Virtual DOM trees. It discusses performance benefits, optimization techniques, and impacts on user experience.Character count: 159
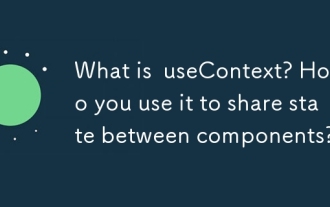
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
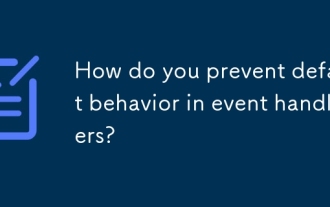
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.
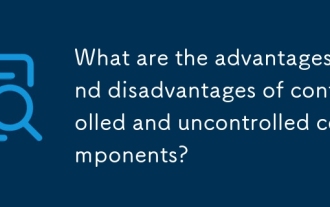
The article discusses the advantages and disadvantages of controlled and uncontrolled components in React, focusing on aspects like predictability, performance, and use cases. It advises on factors to consider when choosing between them.
