How to select the current row in jquery
jQuery is a very popular JavaScript library that can help developers easily write highly interactive and responsive Web pages. In jQuery, selecting elements is a very common operation, and selecting the current row (also called current row highlighting) is an important requirement during the development process. In this article, we will explore how to select and style the current row using jQuery.
1. Requirements Analysis
In web development, we usually use tables to display data. When we move the mouse to a row (tr) of the table, we hope that the row will be highlighted to remind the user of the currently selected row. Therefore, we need to write a piece of jQuery code to achieve this requirement.
2. Code Implementation
In the process of realizing this requirement, we can use jQuery's on() method and siblings() method to select the current row and add styles to it. The specific implementation steps are as follows:
-
Bind a mouse over event for each row (tr) of the table, and the event is triggered when the mouse moves to a certain row.
$('table tr').on('mouseover', function() { // 鼠标滑过事件处理逻辑 });
Copy after login In the event processing logic, use the siblings() method to select the sibling elements of the current row, and then add styles to them.
$('table tr').on('mouseover', function() { $(this).siblings().removeClass('currentRow'); // 移除其他行的样式 $(this).addClass('currentRow'); // 为当前行添加样式 });
Copy after loginDefine the .currentRow class in CSS to set the style of the current row.
.currentRow { background-color: #f0f0f0; }
Copy after login
The implementation principle of this code is that when the mouse slides over a certain row, first use the siblings() method to select the sibling elements of the current row, that is, other rows, and then use removeClass( ) method to remove the existing styles of other rows, and finally use the addClass() method to add a highlight style to the current row.
3. Example demonstration
The following is a simple example to demonstrate how to use jQuery to select the current row and add styles to it.
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>jQuery选择当前行</title> <style> table { width: 100%; border-collapse: collapse; } table th, table td { padding: 10px; border: 1px solid #ccc; text-align: left; } .currentRow { background-color: #f0f0f0; } </style> </head> <body> <table> <thead> <tr> <th>姓名</th> <th>年龄</th> <th>性别</th> <th>地址</th> </tr> </thead> <tbody> <tr> <td>张三</td> <td>18</td> <td>男</td> <td>北京市海淀区</td> </tr> <tr> <td>李四</td> <td>20</td> <td>女</td> <td>上海市浦东新区</td> </tr> <tr> <td>王五</td> <td>22</td> <td>男</td> <td>广州市天河区</td> </tr> </tbody> </table> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script> $('table tr').on('mouseover', function() { $(this).siblings().removeClass('currentRow'); $(this).addClass('currentRow'); }); </script> </body> </html>
In the above example, we bind the mouse rollover event to each row of the table. When the mouse moves to a certain row, use the siblings() method to select other elements of the current row (i.e. other rows ), remove its existing styles, and finally add a highlight style to the current row.
4. Summary
Selecting the current row is a very common operation in web development and can be easily implemented using jQuery. By using the on() method and siblings() method, we can select the current row and add styles to it. At the same time, when adding styles to the current row, you should pay attention to defining the corresponding style class in CSS to ensure the ease of modifying the style.
The above is the detailed content of How to select the current row in jquery. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


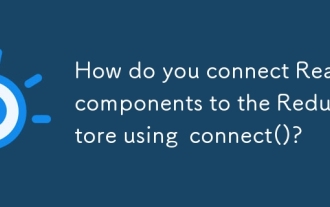
Article discusses connecting React components to Redux store using connect(), explaining mapStateToProps, mapDispatchToProps, and performance impacts.

React combines JSX and HTML to improve user experience. 1) JSX embeds HTML to make development more intuitive. 2) The virtual DOM mechanism optimizes performance and reduces DOM operations. 3) Component-based management UI to improve maintainability. 4) State management and event processing enhance interactivity.
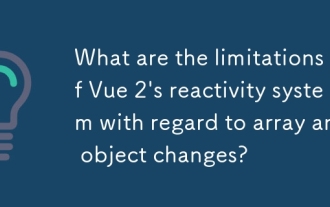
Vue 2's reactivity system struggles with direct array index setting, length modification, and object property addition/deletion. Developers can use Vue's mutation methods and Vue.set() to ensure reactivity.
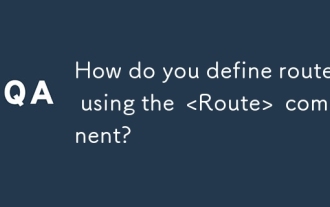
The article discusses defining routes in React Router using the <Route> component, covering props like path, component, render, children, exact, and nested routing.
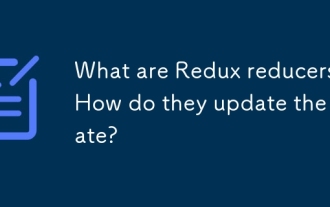
Redux reducers are pure functions that update the application's state based on actions, ensuring predictability and immutability.
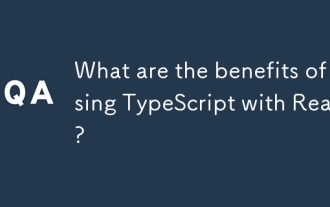
TypeScript enhances React development by providing type safety, improving code quality, and offering better IDE support, thus reducing errors and improving maintainability.
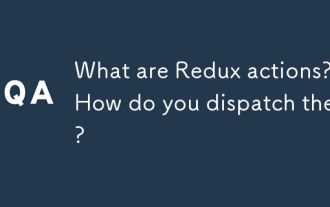
The article discusses Redux actions, their structure, and dispatching methods, including asynchronous actions using Redux Thunk. It emphasizes best practices for managing action types to maintain scalable and maintainable applications.
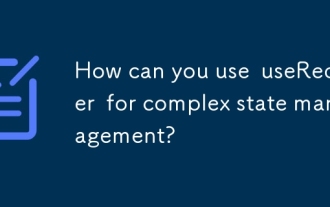
The article explains using useReducer for complex state management in React, detailing its benefits over useState and how to integrate it with useEffect for side effects.
