How to use PHPUnit for PHP unit testing
With the development of the software development industry, testing has gradually become an indispensable part. As the most basic part of software testing, unit testing can not only improve code quality, but also speed up developers' development and maintenance of code. In the field of PHP, PHPUnit is a very popular unit testing framework that provides various functions to help us write high-quality test cases. In this article, we will cover how to use PHPUnit for PHP unit testing.
- Installing PHPUnit
Before using PHPUnit, we need to install it first. PHPUnit is a PHP library that can be installed using Composer. In the root directory of your project, run the following command:
composer require --dev phpunit/phpunit
This will add PHPUnit to your project as a development dependency.
- Writing test cases
Writing test cases is usually the first step in using PHPUnit. A test case is a script that tests one or more functions or methods. Suppose we have a Calculator class:
class Calculator { public function add($a, $b) { return $a + $b; } }
We can use a test case to test the add method in the Calculator class:
use PHPUnitFrameworkTestCase; class CalculatorTest extends TestCase { public function testAdd() { $calculator = new Calculator(); $result = $calculator->add(2, 3); $this->assertEquals(5, $result); } }
In this test case, we create a test case called CalculatorTest Test class, which inherits the PHPUnitFrameworkTestCase class. We also wrote a test method named testAdd, in which we created a Calculator instance and executed the add method, and finally used $this->assertEquals to test whether the result returned by the add method is the same as the expected value.
- Running Test Cases
Once we have written the test cases, we can use PHPUnit to run them. In the root directory of our project, run the following command:
vendor/bin/phpunit
This will run PHPUnit and execute all available test cases. If you only want to run a specific test class or test method, you can use the following command:
vendor/bin/phpunit tests/CalculatorTest.php vendor/bin/phpunit --filter testAdd tests/CalculatorTest.php
- Using assertions
PHPUnit provides a variety of assertion functions , can be used to test whether the return value of a function or method meets our expectations. The following are some of the most commonly used assertion functions in PHPUnit:
- assertTrue($value): Test whether $value is true
- assertFalse($value): Test whether $value is false
- assertEquals($expected, $actual): Test whether $actual is equal to $expected
- assertNotEquals($expected, $actual): Test whether $actual is not equal to $expected
- assertSame($expected, $actual): Test whether $actual is the same as $expected
- assertNotSame($expected, $actual): Test whether $actual is different from $expected
- assertNull($ value): Test whether $value is null
- assertNotNull($value): Test whether $value is not null
- assertInstanceOf($expected, $actual): Test whether $actual is $expected Instance of
- assertNotInstanceOf($expected, $actual): Test whether $actual is not an instance of $expected
- Use data provider
Sometimes we need to test whether a function or method can return results correctly for different inputs. At this time we can use PHPUnit's data provider function. A data provider is a method that provides a set of parameters, each of which is a collection of data that can be tested.
In the following example, we write a data provider for the add method of the Calculator class, which provides different input data and expected output.
class CalculatorTest extends TestCase { /** * @dataProvider additionProvider */ public function testAdd($a, $b, $expected) { $calculator = new Calculator(); $result = $calculator->add($a, $b); $this->assertEquals($expected, $result); } public function additionProvider() { return [ [0, 0, 0], [0, 1, 1], [1, 0, 1], [1, 1, 2], [2, 3, 5], [-1, 1, 0], [10, -5, 5] ]; } }
In this test case, we use the @DataProvider annotation to tell PHPUnit where to find a data provider. We wrote a data provider called additionProvider where we provide different input data and expected output. In the testAdd test method, we passed this data directly and tested it.
- Using Mock Objects
When we test a method in an object, we may not want to rely on other classes or external resources. At this time we can use PHPUnit's Mock object to simulate these dependencies. A Mock object is a mock object that provides the same interface, but it is not actually instantiated.
In the following example, we wrote a Mock object for the add method in the Calculator class to simulate external dependencies.
class CalculatorTest extends TestCase { public function testAdd() { $mock = $this->getMockBuilder('Dependency') ->getMock(); $mock->expects($this->once()) ->method('getValue') ->will($this->returnValue(5)); $calculator = new Calculator($mock); $result = $calculator->add(2, 3); $this->assertEquals(10, $result); } }
In this test case, we use the getMockBuilder method to create a Mock object named Dependency. Then we expect the Mock object's getValue method to be called once and return 5. Finally we pass this Mock object to the constructor of the Calculator class.
Summary
In this article, we learned how to use PHPUnit for PHP unit testing. We installed PHPUnit, wrote test cases, ran the test cases, and used assertions, data providers, and Mock objects. Through these steps, we can write high-quality test cases to test our code in order to improve code quality and speed up development and maintenance.
The above is the detailed content of How to use PHPUnit for PHP unit testing. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


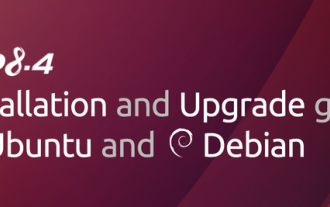
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
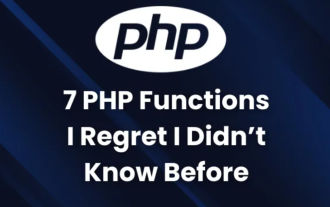
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
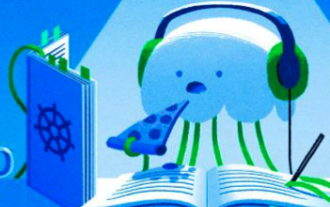
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
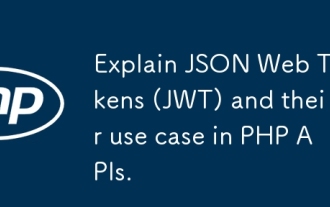
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
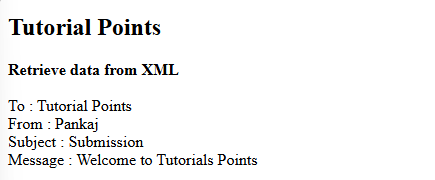
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
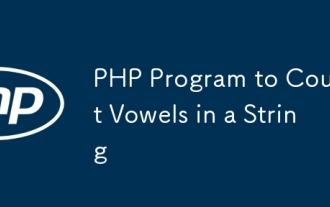
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
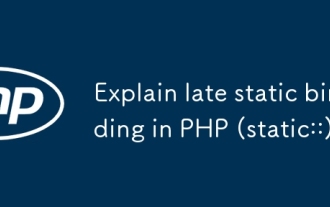
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
