jquery gets Chinese garbled characters in url
In web development, we often need to use jQuery to obtain parameters in the URL. However, when the URL contains Chinese characters, we can easily encounter the problem of Chinese garbled characters. In this article, we will introduce how to use jQuery to obtain Chinese parameters in the URL, and how to deal with the problem of Chinese garbled characters.
1. Get the parameters in the URL
There are usually two ways to get the parameters in the URL:
- Use the window.location.search method to get the parameter string , and then parsed into key-value pairs.
- Use regular expressions to match parameters in the URL.
Below we introduce these two methods respectively.
- Use the window.location.search method to obtain the parameter string
The window.location.search method can obtain the parameter string in the URL, for example:
http://example.com/index.html?id=123&name=张三
You can use the following code to get the parameter string:
var paramsStr = window.location.search; // 返回 ?id=123&name=张三
Then, we can use the JavaScript built-in function decodeURIComponent() to parse the parameter string and convert it into a key-value pair:
function getParams(url) { var paramsStr = url.split("?")[1]; // 获取参数字符串 var paramsArr = paramsStr.split("&"); // 将参数字符串转换为数组 var paramsObj = {}; for (var i = 0, len = paramsArr.length; i < len; i++) { var arr = paramsArr[i].split("="); var name = decodeURIComponent(arr[0]); // 解码中文字符 var value = decodeURIComponent(arr[1]); paramsObj[name] = value; // 将键值对添加到paramsObj中 } return paramsObj; } var params = getParams(window.location.href); console.log(params.id); // 输出 123 console.log(params.name); // 输出 张三
Pay attention here to use decodeURIComponent() to decode Chinese characters, otherwise garbled characters will occur.
- Use regular expressions to match parameters in the URL
Regular expressions can match specified parameter values in the URL, for example:
http://example.com/index.html?id=123&name=张三
can Use the following regular expression to match the value of the id parameter:
var url = window.location.href; var idReg = /id=(d+)/; var id = idReg.exec(url)[1]; console.log(id); // 输出 123
The regular expression id=(d) is used here to match the value of the id parameter, where d represents a number and represents multiple numbers, () is used for grouping Capture matching results.
2. Dealing with the problem of Chinese garbled characters
When obtaining the Chinese parameters in the URL, we often encounter the problem of Chinese garbled characters. This is because the Chinese characters in the URL need to be encoded to be transmitted correctly. Therefore, we need to decode Chinese characters when obtaining URL parameter values, otherwise garbled characters will appear.
In JavaScript, we can use the built-in functions encodeURIComponent() and decodeURIComponent() to encode and decode Chinese characters in the URL.
- encodeURIComponent()
encodeURIComponent() function is used to encode characters in the URL except letters, numbers, (,),.,!,~,*,',- Special characters other than _ are encoded. For example:
var str = "http://example.com/index.html?id=123&name=张三"; var encoded = encodeURIComponent(str); console.log(encoded); // 输出 http%3A%2F%2Fexample.com%2Findex.html%3Fid%3D123%26name%3D%E5%BC%A0%E4%B8%89
Note that the encodeURIComponent() function will not encode spaces, but if you need to encode spaces, you can use the function replace() to replace the space characters:
var str = "http://example.com/index.html?id=123&name=张 三"; var encoded = encodeURIComponent(str.replace(/s/g, "%20")); console.log(encoded); // 输出 http%3A%2F%2Fexample.com%2Findex.html%3Fid%3D123%26name%3D%E5%BC%A0%20%E4%B8%89
- decodeURIComponent()
decodeURIComponent() function is used to decode special characters in the URL, for example:
var str = "http%3A%2F%2Fexample.com%2Findex.html%3Fid%3D123%26name%3D%E5%BC%A0%E4%B8%89"; var decoded = decodeURIComponent(str); console.log(decoded); // 输出 http://example.com/index.html?id=123&name=张三
It should be noted that if the Chinese characters in the URL are not processed correctly Encoding will cause garbled characters in decoding. If you encounter this situation, you can use the function unescape() to decode the URL:
var str = "http://example.com/index.html?id=123&name=张三"; var encoded = escape(str); // 编码 var decoded = unescape(encoded); // 解码 console.log(decoded); // 输出 http://example.com/index.html?id=123&name=张三
Note here that the unescape() function has been abandoned and is not recommended. It is recommended to correctly encode Chinese characters when transmitting URLs to avoid garbled characters.
3. Conclusion
Obtaining parameters in the URL is a basic operation in Web development, and the problem of Chinese garbled characters is also a common development difficulty. To avoid these problems, we can use the encodeURIComponent() and decodeURIComponent() functions to encode and decode the URL. At the same time, Chinese characters also need to be decoded when obtaining URL parameter values, otherwise garbled characters will appear. Although these operations may be a little tedious, they are indispensable for Chinese websites.
The above is the detailed content of jquery gets Chinese garbled characters in url. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


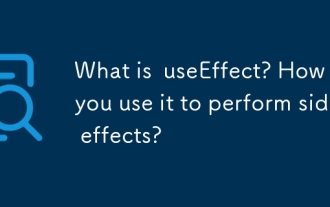
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.
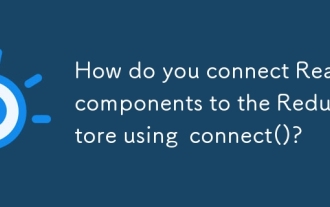
Article discusses connecting React components to Redux store using connect(), explaining mapStateToProps, mapDispatchToProps, and performance impacts.
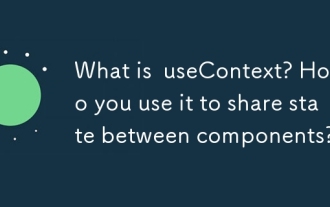
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
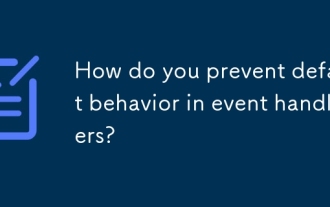
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.
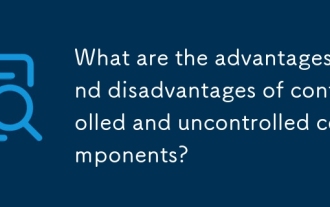
The article discusses the advantages and disadvantages of controlled and uncontrolled components in React, focusing on aspects like predictability, performance, and use cases. It advises on factors to consider when choosing between them.

React combines JSX and HTML to improve user experience. 1) JSX embeds HTML to make development more intuitive. 2) The virtual DOM mechanism optimizes performance and reduces DOM operations. 3) Component-based management UI to improve maintainability. 4) State management and event processing enhance interactivity.
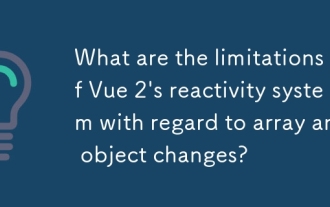
Vue 2's reactivity system struggles with direct array index setting, length modification, and object property addition/deletion. Developers can use Vue's mutation methods and Vue.set() to ensure reactivity.
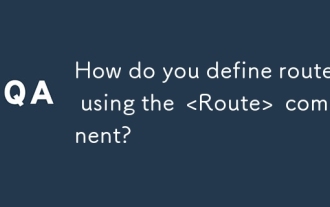
The article discusses defining routes in React Router using the <Route> component, covering props like path, component, render, children, exact, and nested routing.
