You should pay attention to directly calling the service business class in the multi-threaded run method
Using the service business class in the Java multi-threaded run method will cause a java.lang.NullPointerException exception. This is due to the business class injected by spring. is null, or the direct new business object is also null.
Multi-threading will prevent injection for thread safety. Therefore, when you want to use the service business class, you need to use the ApplicationContext method to obtain the bean method to obtain the service class.
To obtain the ApplicationContext class, you must implement the ApplicationContextAware interface, as follows:
import org.springframework.beans.BeansException;
import org.springframework.context.ApplicationContext;
import org.springframework.context.ApplicationContextAware;
public class ApplicationContextUtil implements ApplicationContextAware {
private static ApplicationContext context;
public void setApplicationContext(ApplicationContext context) throws BeansException {
this.context = context;
}
public static ApplicationContext getContext() {
return context;
}
}
Copy after login
Then use the above method in the run method to create a business object, as follows:
XXXServiceI xxxService = ApplicationContextUtil.getContext.getBean(XXXServiceI.class);
Copy after login
This way it can be used normally The business class is over.
The diagram is as follows
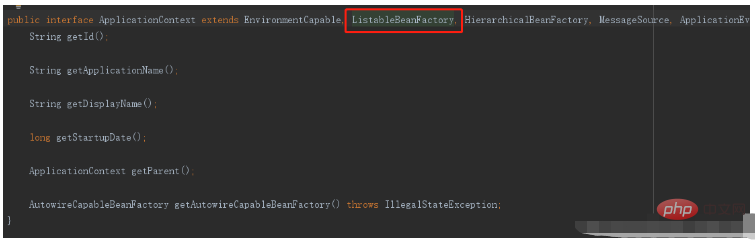

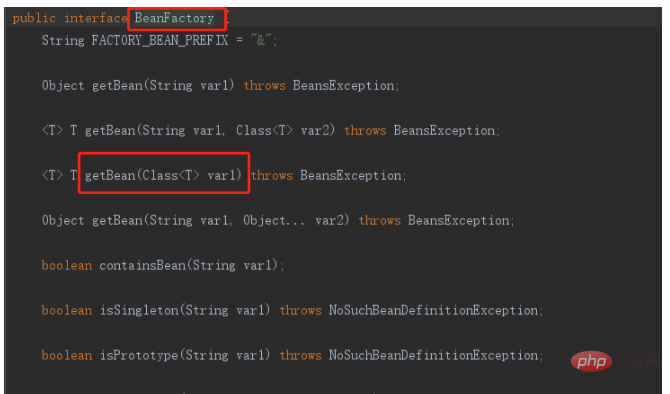
#Multi-threading knowledge points
Four ways to start threads
1. Inherit the Thread class and rewrite the run method of Thread, perform operations in the run method, and use the start method to start the thread
2. Inherit the Runnable interface, implement the run method, and perform operations in the run method. You need to pass in the instance object of the current class to create a Thread instance, and then call the start method to start the thread
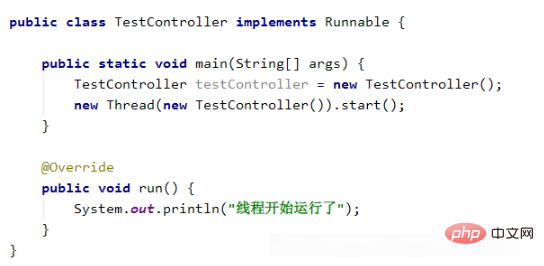
3. Implement the Callable interface and rewrite the call() method. It should be noted that the first two methods do not require a response and are executed directly. However, if you implement the Callable interface and rewrite the call() method, You need to wait for the thread to respond, so although other threads are started, one thread is executing, and it cannot be considered a standard multi-thread.
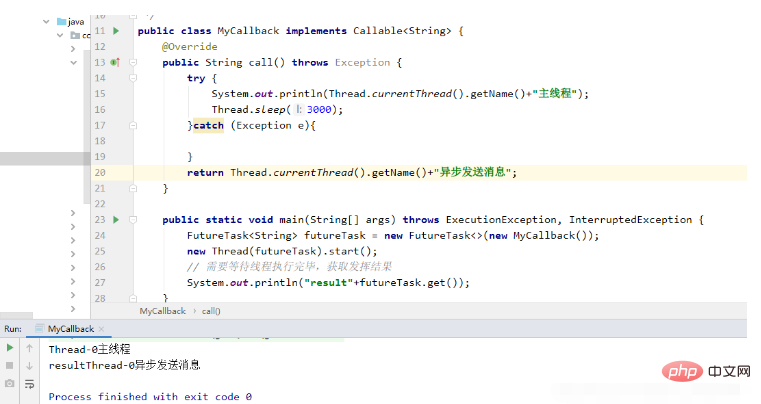
4. Thread pool

#Use @Aysnc annotation to implement multi-threading
The same class , method A refers to method B. Adding asynchronous @Async annotation to method B will not be effective
The added @Async method and the caller cannot be in the same class
The difference between user threads and daemon threads
Threads created in Java default to creating user threads, such as new Thread (thread object).start
Thread thread = new Thread();
// 默认为false,都是用户线程
thread.setDaemon(true); // 表示设置为守护线程
thread.setDaemon(false); // 表示设置为用户线程
Copy after login
User thread: No It dies as other threads die. There are only two situations where it dies. One is that it terminates due to an exception during operation, and the other is that the program is executed normally and the thread dies
Daemon thread: Dies as the user thread dies. When the user thread dies, the daemon thread also dies, such as the gc garbage collection thread. If the user thread exists, then gc needs to be alive, otherwise it will be useless.
Six states of threads
1. New: Initial state, the thread is created, start() is not called
2. Runnable: Run Status, Java threads call the two states of ready and running in the operating system "running"
3. Blocked: blocked, the thread enters the waiting state, and the thread gives up the use of the CPU for some reason
- Several situations of blocking:
- A. Waiting for blocking: The running thread executes wait(), and the JVM will Put it in the waiting queue
- B. Synchronous blocking: When the running thread acquires the synchronization lock of the object, if the synchronization lock is occupied by other threads, the JVM will put the current thread into the lock Pool
- C. Other blocking: When the running thread executes sleep(), join() or issues an IO request, the JVM will set the current thread to the blocking state. When sleep() After execution, the join() thread terminates, and the thread resumes again after IO processing
4. Waiting: waiting state
5. timed_waiting: timeout waiting state, automatically after timeout Return
6. terminated: Termination status, the current thread has completed execution
Reentrancy of Java lock
The reentrancy mechanism of Java lock can solve the following problem , go directly to the code:
public class Demo1 {
public synchronized void functionA(){
System.out.println("iAmFunctionA");
functionB();
}
public synchronized void functionB(){
System.out.println("iAmFunctionB");
}
Copy after login
Assume that Java does not provide a synchronized internal lock mechanism that forces atomicity: functionA() and functionB() are both synchronized methods. When the thread enters funcitonA(), it will obtain the object lock of this class. , this lock "new Demo1()" calls the method functionB() in functionA(), but functionB() is also synchronized, so the thread needs to obtain the object lock (new Demo1()) again, but the JVM will It is considered that this thread has acquired the lock of this object and cannot acquire it again, so it cannot call the functionB() method, causing a deadlock.
Four rejection strategies of thread pool
When the task cache queue of the thread pool is full and the number of threads in the thread pool reaches maximumPoolSize, if there are still tasks arriving, the task rejection strategy will be adopted. Usually there are The following four strategies:
ThreadPoolExecutor.AbortPolicy
: Discard the task and throw RejectedExecutionException.
ThreadPoolExecutor.DiscardPolicy
: Discard the task, but do not throw an exception.
ThreadPoolExecutor.DiscardOldestPolicy
: Discard the frontmost task of the queue, and then resubmit the rejected task
ThreadPoolExecutor.CallerRunsPolicy
: By the calling thread ( The thread that submitted the task) processes the task
The difference between sleep and wait
sleep is a method in the thread, but wait is a method in Object
The sleep method will not release the lock, but wait will release it and will be added to the waiting queue
-
sleep does not need to be awakened, but wait does
Why wait(), notify(), notifyAll() are in the object and not in the Thread class
The lock level in java is the object level instead of the thread level. Each object has a lock, which is obtained through the thread. If the wait() method is in a thread, it is not obvious which lock the thread is waiting for.
The above is the detailed content of How to directly call service business class in Java multi-threaded run method. For more information, please follow other related articles on the PHP Chinese website!