How to read xls in golang
golang is an efficient, concise, and easy-to-use programming language that is widely used in web development, cloud computing and other fields. In actual projects, reading Excel files is a very common requirement. This article will introduce how to use golang to read xls files and give a complete code example.
1. Install dependency packages
Before using golang to read xls files, we need to install an open source library first, which is called "go-xls". go-xls is an xls parsing library for golang, which provides an API interface for reading Excel.
Use the go command to install the go-xls package:
go get github.com/extrame/go-xls
The above command will automatically download and install the dependent packages. After completion, you can start using go-xls.
2. Reading xls files
Next, we will demonstrate how to use go-xls to read Excel files and output the contents to the console.
We re-create a file named "read_xls.go", the code framework is as follows:
package main import ( "fmt" "github.com/extrame/go-xls" ) func main() { // xls 文件路径 filepath := "./test.xls" // 打开 xls 文件 xlFile, err := xls.Open(filepath, "utf-8") if err != nil { fmt.Println(err) return } // 获取 Excel 中的第一张表 sheet := xlFile.GetSheet(0) // 遍历表格中的所有行和列 for i := 0; i <= int(sheet.MaxRow); i++ { row := sheet.Rows[i] for _, cell := range row.Cells { fmt.Printf("%s ", cell.String(xlFile)) } } }
Before reading the xls file, we need to do some preparation work. Open an xls file through the Open function and specify the encoding method (usually "utf-8"). If an error occurs, a message indicating failure to open the file will be returned; otherwise, reading of the Excel file will continue.
Because an Excel file can contain multiple tables, in this article we only demonstrate how to read the first table. Use the GetSheet function to get the first sheet in Excel and iterate through all rows and columns in the sheet. For each cell, we use the String function to convert its contents into a string. Finally, use fmt.Printf to print the cell contents to the console.
3. Complete code example
The following is a complete code example, which can read any xls file and output the contents of all tables to the console. Comments in the code can help you better understand each step.
package main import ( "fmt" "github.com/extrame/go-xls" ) func main() { // xls 文件路径 filepath := "./test.xls" // 打开 xls 文件 xlFile, err := xls.Open(filepath, "utf-8") if err != nil { fmt.Println(err) return } // 遍历 xls 中的所有表格 for i := 0; i < xlFile.NumSheets(); i++ { sheet := xlFile.GetSheet(i) // 遍历表格中的所有行和列 for i := 0; i <= int(sheet.MaxRow); i++ { row := sheet.Rows[i] for _, cell := range row.Cells { fmt.Printf("%s ", cell.String(xlFile)) } } } }
4. Summary
This article introduces how to use golang to read xls files. By using the go-xls library, we can easily read the contents of Excel files and perform related processing. I hope the content of this article can help you solve problems in actual projects.
The above is the detailed content of How to read xls in golang. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
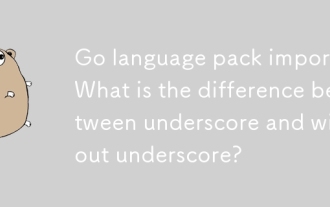
This article explains Go's package import mechanisms: named imports (e.g., import "fmt") and blank imports (e.g., import _ "fmt"). Named imports make package contents accessible, while blank imports only execute t
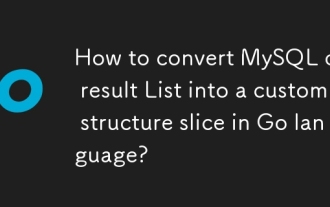
This article details efficient conversion of MySQL query results into Go struct slices. It emphasizes using database/sql's Scan method for optimal performance, avoiding manual parsing. Best practices for struct field mapping using db tags and robus

This article explains Beego's NewFlash() function for inter-page data transfer in web applications. It focuses on using NewFlash() to display temporary messages (success, error, warning) between controllers, leveraging the session mechanism. Limita
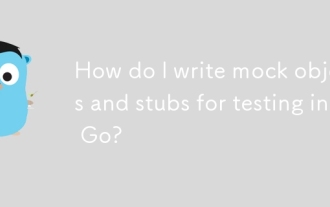
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
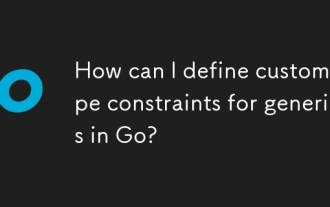
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
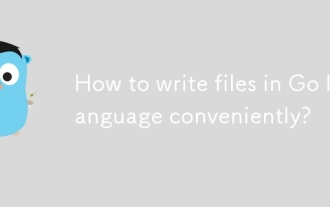
This article details efficient file writing in Go, comparing os.WriteFile (suitable for small files) with os.OpenFile and buffered writes (optimal for large files). It emphasizes robust error handling, using defer, and checking for specific errors.
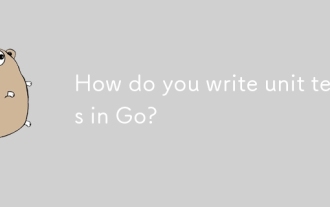
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
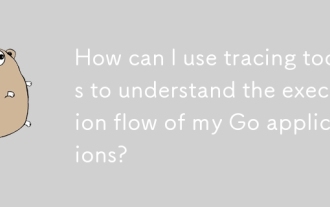
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
