How to use Elasticsearch technology in PHP?
With the rise of Web applications, search engines have become an essential feature of modern applications. In the past, we used SQL queries to search data, but SQL was not designed specifically for searching. In order to make up for this shortcoming, full-text search engines were created, such as Apache Solr, Elasticsearch, etc.
Elasticsearch is a popular Lucene-based full-text search engine that provides out-of-the-box distributed search and analysis capabilities for real-time data analysis and search engines. Compared with traditional relational databases, Elasticsearch can perform queries faster, can better handle highly dynamic data structures, and supports richer query languages.
In this article, we will introduce how to use Elasticsearch in PHP applications.
Environment preparation
First, we need to install Elasticsearch on the local environment or remote server. Elasticsearch supports all common operating systems, including Windows, macOS, and Linux. You can get various versions of the installer on the official website, or you can use the package manager to install it.
In order to use PHP's elasticsearch client library, we also need to install PHP's elasticsearch client extension. It can be downloaded and compiled and installed via PECL or manually. The following is an example of using PECL:
pecl install elasticsearch
Then add the following line in php.ini:
extension=elasticsearch.so
After the installation is complete, we can use PHP to operate Elasticsearch.
Using Elasticsearch in PHP
Using Elasticsearch in PHP requires using an Elasticsearch client class or library. Currently, there are many PHP Elasticsearch client libraries available, including Elasticsearch-PHP, Elasticsearch-DSL, and Elastica, among others.
In this article, we will use the Elasticsearch-PHP library to demonstrate the use of Elasticsearch.
First, we need to create an Elasticsearch client object:
$client = ElasticsearchClientBuilder::create()->build();
Now, we can use this client object to establish a connection with Elasticsearch and perform various operations.
Indexing and searching documents
In Elasticsearch, documents refer to data in JSON format. Using the PHP Elasticsearch client library, we can easily convert PHP arrays to JSON format and index them into Elasticsearch. First, we need to select an index (similar to a table in a relational database) and then add data to that index.
$params = [ 'index' => 'my_index', 'type' => 'my_type', 'id' => '1', 'body' => ['title' => 'My first document', 'content' => 'Hello World'] ]; $response = $client->index($params);
In the above code, we used the index
method to index the document. The index
method requires a parameter array containing at least the following keys:
index
: The name of the indextype
: Type of documentid
: Unique identifier of the documentbody
: Array or JSON format string containing document data
The above code example creates an index named my_index
, of type my_type
, with a document unique identifier of 1, and contains a title
and content
fields. Once the documents are indexed and stored in Elasticsearch, we can search them.
$params = [ 'index' => 'my_index', 'type' => 'my_type', 'body' => [ 'query' => [ 'match' => [ 'title' => 'My first document' ] ] ] ]; $response = $client->search($params);
In the above code, we use the search
method to search for documents. The search
method requires an argument array containing at least the following keys:
index
: The name of the index to searchtype
: The type of document to search forbody
: The array containing the actual search query
The above code example searched for my_index
In the index, documents of type my_type
and title
contain My first document
. The search result is a JSON-formatted response containing documents matching the query.
Paging and Sorting
When the search result set is large, we may want to paginate or sort the results. We can use the parameters provided by Elasticsearch to achieve these two functions.
$params = [ 'index' => 'my_index', 'type' => 'my_type', 'body' => [ 'query' => [ 'match' => [ 'title' => 'document' ] ] ], 'size' => 10, 'from' => 0, 'sort' => ['title' => ['order' => 'asc']] ]; $response = $client->search($params);
In the above code, we added the following additional parameters:
size
: The number of documents per pagefrom
: The position of the starting document, used for pagingsort
: Sort in ascending order by thetitle
field
The above example obtains the first 10 documents matching document
and sorts them in ascending order by the title
field.
Aggregation Search
Elasticsearch also supports aggregate search, a technique that performs various analyzes on a search result set. For example, we can extract all unique values for the author
field from the search results.
$params = [ 'index' => 'my_index', 'type' => 'my_type', 'body' => [ 'aggs' => [ 'unique_authors' => [ 'terms' => [ 'field' => 'author.keyword', 'size' => 10 ] ] ] ] ]; $response = $client->search($params);
In the above code, we use aggs
as the new key in the parameter array and define an aggregate search named unique_authors
in it. terms
means that we will group and aggregate based on the value of the author
field. The field
key is used to specify the field to be aggregated, and the size
key specifies the size limit of the aggregated grouping.
in conclusion
Elasticsearch is a powerful full-text search engine that has become an indispensable part of many modern web applications. It can help us better process data and conduct real-time searches. This article explains how to use Elasticsearch in PHP and how to index, search, paginate, and sort documents. In addition, it also introduces how to use Elasticsearch for aggregate search. Now, you have learned how to use Elasticsearch to implement efficient and fast searches in your PHP applications.
The above is the detailed content of How to use Elasticsearch technology in PHP?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


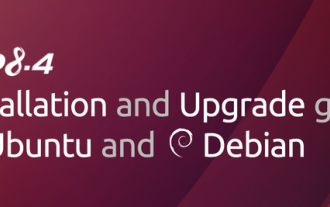
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
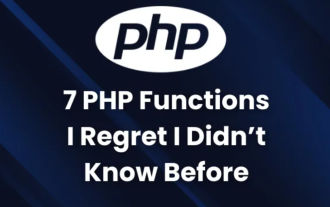
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
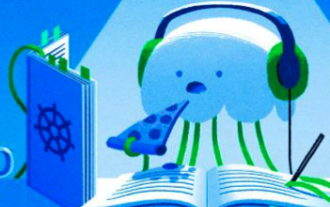
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
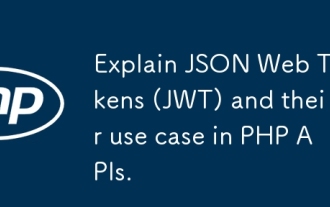
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
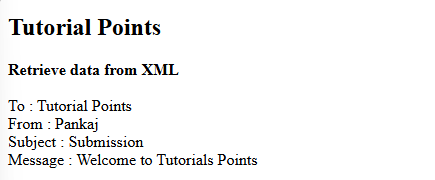
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
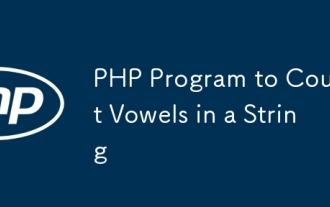
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
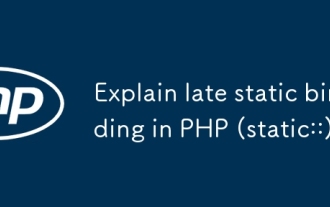
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
