golang int to string
In Go programming, it is often necessary to convert int type to string type. If you are a developer who has just started learning the Go language, you may feel that this conversion operation seems very simple. You only need to use the Sprintf function provided by the fmt package of the standard library. However, the conversion operation we are going to talk about today pays more attention to details and efficiency. This article will give you a detailed introduction to how to efficiently convert int type to string type in Go language.
1. Summary of the method of converting int to string
Before introducing the specific method of converting int to string, let’s first understand how the int type is converted into a string type in the Go language. Commonly used method:
- fmt.Sprintf("%d", i), this method should be the most well-known. Its implementation is based on the strconv.FormatInt method, calling the method strconv.FormatInt(int64(i), 10). However, the disadvantage of this method is that it consumes more computing resources.
- strconv.Itoa(i) can convert the integer i into the decimal format of the string type, which is equivalent to fmt.Sprintf("%d", i).
- strconv.FormatInt(int64(i), 10) can convert the int type to the string type.
- strconv.AppendInt(buf []byte, i int64, base int), this function can convert int type data to string type and save the result to a cache (buf), where buf It is a slice of byte type, and base specifies the base number. Conversion between int type and other data types can be achieved by operating strings.
2. Using the strconv.Itoa method
The Go language provides the strconv.Itoa method, which can convert the int type into a decimal string type. The specific implementation is as follows:
func Itoa(i int) string {
return FormatInt(int64(i), 10)
}
The FormatInt method will convert the int64 type i to base format and return the string type. When the parameter base is 10, the returned string type is expressed in decimal format.
For shorter string conversions, Itoa's performance is better than other methods.
3. Use the strconv.FormatInt method
For the need to convert the int type to other hexadecimal string types, assume that we convert the int type to a hexadecimal string type and implement the code As follows:
func ConvertIntToHex(num int) string {
return strconv.FormatInt(int64(num), 16)
}
In this way, the int type can be converted into a hexadecimal string type. For the octal string type, the strconv.FormatInt() method can also be used, and you only need to set the second parameter to 10.
4. Use the strconv.AppendInt(buf []byte, i int64, base int) method
In some high-performance scenarios, we may need to use the strconv.AppendInt method to implement int Convert to string type. For example, in the scenario where bufio.Writer is used, int type data can be written to the cache, and the cached data can be refreshed to the file immediately after the cache reaches a certain level or reaches a certain time.
The following is a sample code that uses strconv.AppendInt to convert int to string type:
func AppendIntToString(i int, buf []byte) []byte {
return strconv.AppendInt(buf, int64(i), 10)
}
Among them, the third parameter represents the base number. The second parameter represents the cache of the result. The function will append the result to this cache and return the modified buf slice.
5. Performance comparison
Through performance testing, we can compare the performance of the above three methods. The first is the fmt.Sprintf method:
func BenchmarkFmtSprintf(b *testing.B) {
for i := 0; i < b.N; i++ { s := fmt.Sprintf("%d", i) _ = s }
}
The second is the strconv.Itoa method:
func BenchmarkItoa(b *testing.B) {
for i := 0; i < b.N; i++ { s := strconv.Itoa(i) _ = s }
}
Finally is the strconv.FormatInt method:
func BenchmarkFormatInt(b *testing.B) {
for i := 0; i < b.N; i++ { s := strconv.FormatInt(int64(i), 10) _ = s }
}
From the results, the strconv.Itoa method has the best performance, and fmt.Sprintf has the worst performance. In the comparison, the difference between the first two functions is not very big. Although the fmt.Sprintf method can also be used to complete the conversion, in high concurrency and performance-sensitive situations, we still recommend the strconv.Itoa method.
6. Summary
This article mainly introduces three methods for converting int type to string type in Go language: strconv.Itoa, strconv.FormatInt and strconv.AppendInt. Among them, the strconv.Itoa method is suitable for short string conversion, while the strconv.FormatInt method and strconv.AppendInt method have better performance and are more suitable for high concurrency and performance-sensitive situations. At the same time, we also use the method when using it. Use it selectively.
In short, in order to improve the performance of the application, we need to use different methods on different occasions, combined with specific business needs, to effectively complete the conversion of the int type to the string type.
The above is the detailed content of golang int to string. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


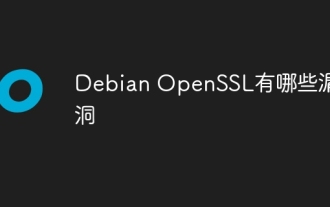
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
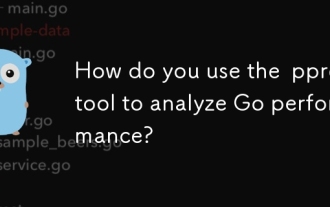
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
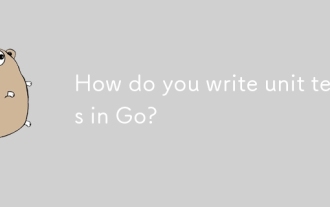
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
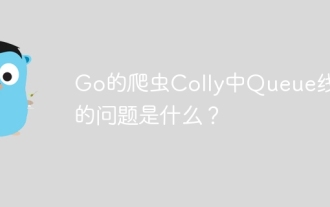
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
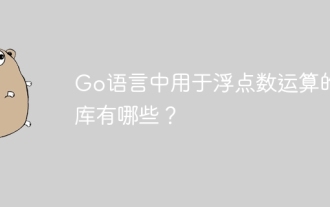
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
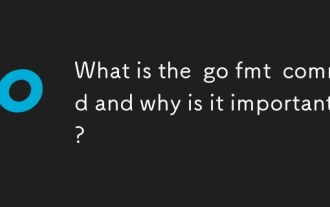
The article discusses the go fmt command in Go programming, which formats code to adhere to official style guidelines. It highlights the importance of go fmt for maintaining code consistency, readability, and reducing style debates. Best practices fo
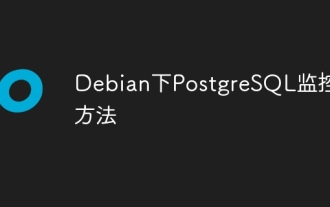
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
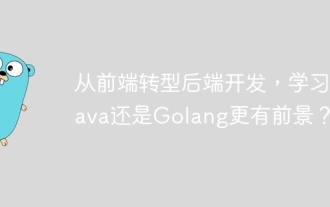
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
