golang field type conversion
Golang field type conversion
In Golang, the type of variables is relatively strict, which is one of the reasons why the Golang language design is excellent. But in actual programming scenarios, we will encounter situations where conversion between different types of values is required. Therefore, it is important to understand type conversion in Golang.
In Golang, type conversion refers to converting a variable from one type to another type. Let's take a look at how to perform type conversion in Golang.
- Same type conversion
First, let’s look at the same type conversion. When we need to convert values of the same type into different expressions, we can use cast to achieve this. For example:
var a int = 65
var b string = string(a)
The above code converts the value of variable a to an ASCII value of one character Assign a value to variable b. In Golang, basic data types support casts. However, it should be noted that during type conversion, it is necessary to ensure that the actual types of variables before and after conversion are compatible.
- Different type conversion
When we need to convert values of different types into target types, we need to use forced type conversion. Since Golang is a statically typed language, when performing forced type conversion, you need to ensure that the converted type is compatible with the variable storage content, otherwise exceptions or unexpected results will occur. For example:
var a float32 = 3.14
var b int = int(a)
In the above code, we convert a floating point type variable a to an integer type, and Assign the result to an integer variable b. Since the precision of floating point types is higher than that of integer types, conversion of floating point types may cause data loss and needs to be used with caution.
There are also some data type conversions that need attention. For example, since the default conversion from string to integer type is not supported in Golang, you need to use the strconv package when converting a string type number to an integer type. For example:
import "strconv"
func main() {
str := "1234"
num, _ := strconv.Atoi(str)
fmt .Printf("Type: %T, Value: %d", num, num)
}
In the above code, we need to use the Atoi method in the strconv package to convert the string type number For integer type.
In addition to the strconv package, there are also JSON, protobuf and other libraries specifically used for data serialization and deserialization, which can realize conversion between different data types.
- Interface type conversion
Golang is a strongly typed language, but in some cases, we need to establish a common interface between different types. At this time, we can use interface type conversion. For example:
type MyInterface interface {
Method1()
Method2()
}
type MyStruct struct {
name string
}
func (m *MyStruct) Method1() {
fmt.Println("Method1 called")
}
func (m *MyStruct) Method2() {
fmt. Println("Method2 called")
}
func main() {
var i MyInterface
i = &MyStruct{"MyStruct"}
m := i.(*MyStruct )
fmt.Printf("Value: %#v", m)
}
In the above code, we define a MyInterface interface, which defines two methods. Then a structure MyStruct that implements the MyInterface interface is defined, and the interface type variable i is used for assignment in the main function. Finally, we use interface type conversion to convert the interface type variable i to the MyStruct type and print the value of the variable m.
It should be noted that when performing interface type conversion, you must ensure that the source type implements all methods of the target interface, otherwise a runtime exception will occur.
Summary
Type conversion is an important topic in Golang programming, which can help us quickly and accurately complete data type conversion when we need to convert different data types. However, it should be noted that when performing data type conversion, the compatibility of types before and after conversion must be ensured, otherwise unexpected results will result.
The above is the detailed content of golang field type conversion. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




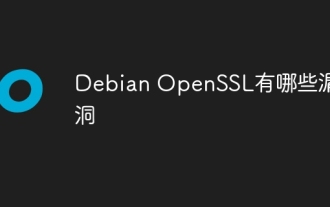
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
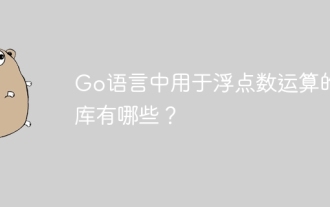
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
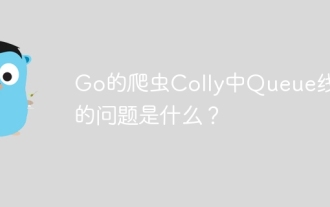
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
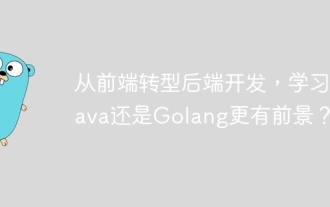
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
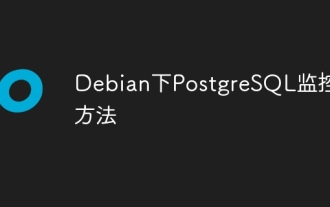
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
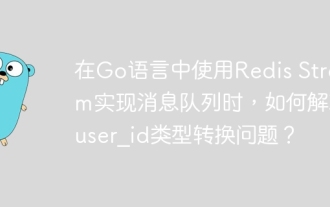
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
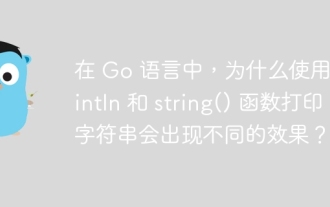
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
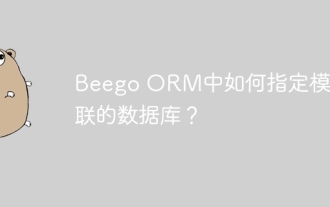
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
