golang gets query results
In order to obtain query results, Golang provides different methods and tools. In this article, we'll explore methods and techniques for obtaining query results.
- Using a database driver
Using a database driver is a common method of obtaining query results. Golang supports a variety of database drivers, including MySQL, PostgreSQL, and SQLite. Before using the database driver, you need to install the corresponding library. For example, in order to use the MySQL driver, you need to run the following command:
go get -u github.com/go-sql-driver/mysql
This will download and install the MySQL driver.
Next, you need to use the database/sql package to execute the query and get the results. Below is a sample program that uses the MySQL driver to connect to a MySQL database and execute a simple SELECT query:
package main import ( "database/sql" "fmt" _ "github.com/go-sql-driver/mysql" ) func main() { db, err := sql.Open("mysql", "user:password@tcp(hostname:port)/database") if err != nil { panic(err.Error()) } defer db.Close() rows, err := db.Query("SELECT * FROM users") if err != nil { panic(err.Error()) } defer rows.Close() var id int var name string for rows.Next() { err = rows.Scan(&id, &name) if err != nil { panic(err.Error()) } fmt.Println(id, name) } }
In the above program, we use the sql.Open() method to connect to MySQL database. In the connection string, we specify the database server’s hostname, port, and database name. Then, we use the db.Query() method to execute the SELECT query and the row data in the query's result set. For each row, we use the rows.Scan() method to read the column data in the row.
- Using the ORM framework
The ORM (Object Relational Mapping) framework is another popular method of obtaining query results in Golang. The ORM framework allows you to map database tables into structures and fields in Golang and provides various methods to perform queries and manipulate the database. Some of the popular ORM frameworks in Golang include GORM, XORM, Beego ORM, etc.
The following is a sample program that uses the GORM framework to obtain query results:
package main import ( "fmt" "gorm.io/driver/mysql" "gorm.io/gorm" ) type User struct { ID uint Name string } func main() { dsn := "user:password@tcp(hostname:port)/database" db, err := gorm.Open(mysql.Open(dsn), &gorm.Config{}) if err != nil { panic(err.Error()) } defer db.Close() var users []User db.Find(&users) for _, user := range users { fmt.Println(user.ID, user.Name) } }
In the above program, we use GORM to map the User table to the User structure in Golang. We then execute the SELECT query using the db.Find() method and save the query results into a slice. Finally, we iterate over the slice to print each user's ID and name.
- Using HTTP client
If you need to get query results from a web service, you can use Golang's built-in HTTP client. HTTP clients allow you to send requests to web services with HTTP interfaces and get results from the responses.
The following is a sample program that uses an HTTP client to obtain query results:
package main import ( "encoding/json" "fmt" "net/http" ) type User struct { ID int `json:"id"` Name string `json:"name"` } func main() { url := "https://example.com/users" resp, err := http.Get(url) if err != nil { panic(err.Error()) } defer resp.Body.Close() var users []User err = json.NewDecoder(resp.Body).Decode(&users) if err != nil { panic(err.Error()) } for _, user := range users { fmt.Println(user.ID, user.Name) } }
In the above program, we use the http.Get() method to request a user list from a Web service. We then decode the JSON formatted response using the json.NewDecoder() method and map it into the User slice. Finally, we iterate over the slice to print each user's ID and name.
Summary
The above are three common ways to obtain query results in Golang: using a database driver, using an ORM framework and using an HTTP client. These tips can help you write efficient and flexible code to better process and obtain query results. Whether you're getting query results from a relational database or from a Web service, these tips can help you easily get the results and process them.
The above is the detailed content of golang gets query results. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


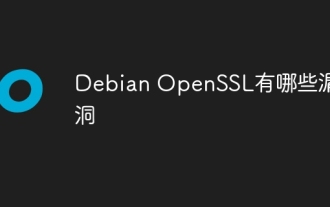
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
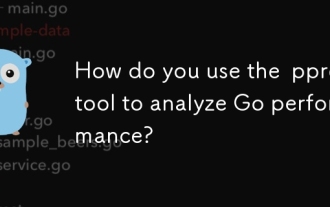
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
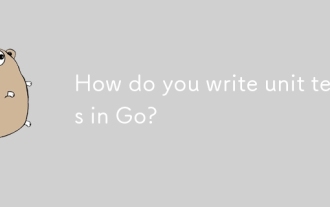
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
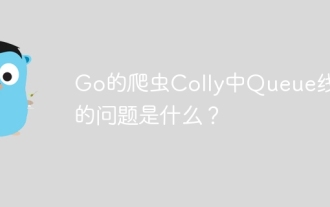
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
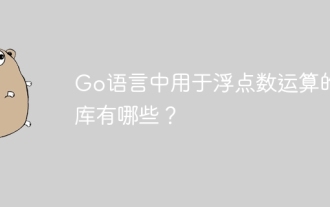
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
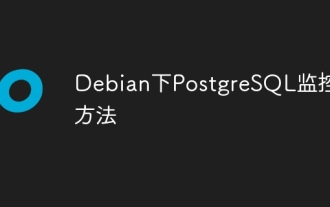
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
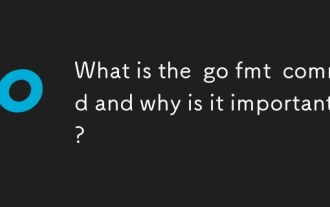
The article discusses the go fmt command in Go programming, which formats code to adhere to official style guidelines. It highlights the importance of go fmt for maintaining code consistency, readability, and reducing style debates. Best practices fo
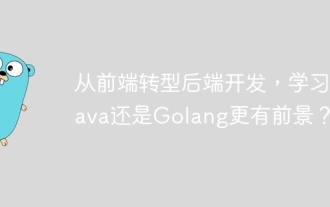
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
