How to implement golang multi-core
In today's era of continuous improvement in computer performance, multi-core processors have gradually become mainstream hardware devices. At the same time, we also need to understand the parallel computing technology on multi-core processors and master these technologies in practice. .
As a programming language with high performance, easy to learn and use, and concurrency advantages, Golang is also very flexible in supporting multi-core processors. This article will introduce how to implement multi-core parallel computing in Golang.
1. Golang concurrency model
In Golang, a concurrency model based on CSP (Communicating Sequential Processes) is adopted, including goroutine and channel.
Goroutine is Golang's lightweight coroutine. Compared with traditional threads, switching between coroutines consumes very few resources, and multiple coroutines can be run simultaneously in one thread, which greatly improves program efficiency. concurrency capabilities.
Channel is also a very important concept in Golang, which can be used for communication and data exchange between coroutines.
Compared with other programming languages such as Java, Golang's concurrent programming model is more lightweight and concise, but it has certain limitations for making full use of multi-core processors. Below we will introduce how to optimize the performance of Golang programs in a multi-core environment.
2. Multi-core optimization of Golang programs
In a multi-core environment, the CPU can run multiple tasks in parallel at the same time. However, if the program cannot reasonably utilize these tasks, it will not be able to perform multi-core processing. maximum performance of the device. In order to better utilize multi-cores, we can optimize in the following aspects:
- Concurrent execution
First of all, in Golang, we can implement the program through goroutine Execute concurrently to fully utilize the performance of the CPU. Each goroutine can run on a different CPU core, which means we can run multiple goroutines at the same time to increase processing power.
- Parallel Computing
In addition to the concurrent execution of goroutine, we can also implement parallel computing of the program by using Golang's built-in sync package. This package provides some useful tools, such as WaitGroup, Mutex, etc., which can lock between multiple goroutines to avoid race conditions. In this way, we can execute tasks in parallel on multiple CPU cores, thereby improving the computing performance of the program.
When using the sync package for parallel computing, we need to consider the following factors:
2.1 Divide tasks
First, we need to divide the tasks to be processed into several Subtasks are assigned to different goroutines. Doing so takes full advantage of the multi-core processor's performance as much as possible.
2.2 Merging results
After the goroutine is executed in parallel, we need to merge the results of each subtask to get the final result. This requires us to design appropriate data structures and algorithms so that corresponding operations can be performed efficiently when results are collected.
- GOMAXPROCS
The last optimization point is GOMAXPROCS. This environment variable can limit the number of CPU cores that the Golang program can run at the same time. When the value is set to 1, the program will only run on one CPU core, and when the value is set to a number greater than 1, the program can run in parallel on multiple CPU cores.
The value of GOMAXPROCS can be modified in the following ways:
import "runtime"
func init() {
runtime.GOMAXPROCS(4) // 设置使用4个CPU核心
}
It should be noted that GOMAXPROCS should not be set to a value that is too high, as this may lead to resource conflicts and race conditions.
3. Conclusion
Golang is a programming language with powerful performance and excellent concurrency. Its concurrency model can help us make full use of the performance of multi-core processors. When optimizing Golang programs in a multi-core environment, we can optimize program performance by dividing the task into multiple subtasks, using the sync package for parallel computing and the GOMAXPROCS environment variable to limit the number of CPU cores.
Of course, for Golang's multi-core optimization, the gods are above and you must have practical experience. In the actual development process, we need to constantly try, adjust, and find the best optimization strategy according to our own situation, so as to maximize the advantages of the Golang language on multi-core processors.
The above is the detailed content of How to implement golang multi-core. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


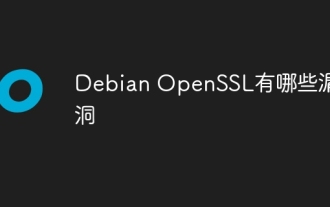
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
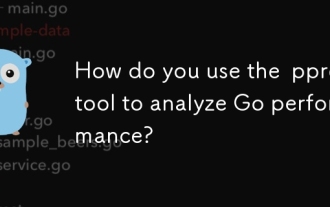
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
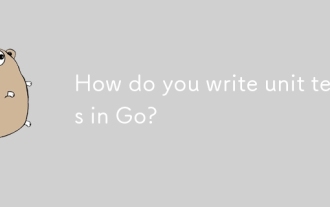
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
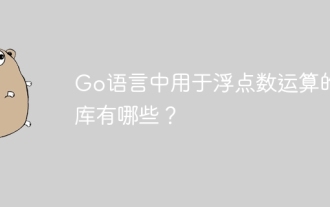
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
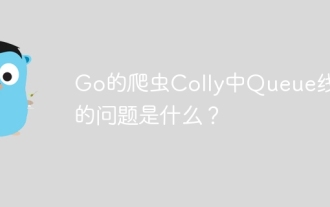
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
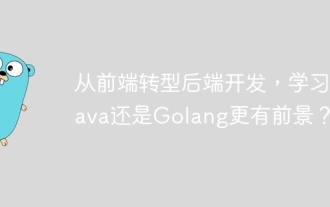
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
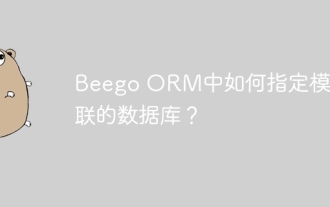
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
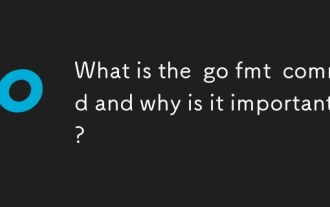
The article discusses the go fmt command in Go programming, which formats code to adhere to official style guidelines. It highlights the importance of go fmt for maintaining code consistency, readability, and reducing style debates. Best practices fo
