How to use Java multi-thread synchronization tool class CyclicBarrier
What is CyclicBarrier
CyclicBarrier is a synchronization tool class provided in the Java concurrency package, which allows multiple threads to wait at a certain barrier , execution will not continue until all threads reach the barrier. The implementation principle of CyclicBarrier is based on ReentrantLock and Condition, and the waiting and waking up of threads are realized by calling the await() method multiple times.
Basic usage of CyclicBarrier
The basic usage of CyclicBarrier is very simple. You only need to create a CyclicBarrier object and set the counter value to the number of waiting threads. After each thread finishes executing, call the await() method of CyclicBarrier to wait for other threads to finish executing. When all threads reach the barrier, the barrier will be opened and all threads will continue to execute.
Source code implementation of CyclicBarrier
The implementation principle of CyclicBarrier is based on ReentrantLock and Condition, and the waiting and waking up of threads are realized by calling the await() method multiple times. The source code implementation of CyclicBarrier mainly includes two parts: initialization of the barrier and waiting and waking up of the barrier.
(1) Initialization of CyclicBarrier
When creating a CyclicBarrier object, you need to specify the number of waiting threads and the execution operation of the barrier. The construction method of the CyclicBarrier object is as follows:
public CyclicBarrier(int parties, Runnable barrierAction)
Among them, parties represents the number of waiting threads, and barrierAction represents the operation performed by the barrier.
In the construction method, a parties-sized ReentrantLock array and a Condition object will be created based on the number of waiting threads. The ReentrantLock array is used to ensure that multiple threads can reach the barrier and wait at the same time, and the Condition object is used to wait and wake up threads.
(2) Waiting and waking up of CyclicBarrier
When the thread executes the await() method, it will first try to acquire the lock of the ReentrantLock object. If the acquisition fails, the thread will be added to the waiting queue. Waiting for the lock to be released. After acquiring the lock, the thread will determine whether the current counter has reached the number of waiting threads. If so, it will perform barrier operations and reset the counter to parties, waking up all threads in the waiting queue. If the counter does not reach the number of waiting threads, the thread will be added to the waiting queue to wait for the arrival of other threads.
The source code of CyclicBarrier's await() method is as follows:
public int await() throws InterruptedException, BrokenBarrierException { try { // 获取锁 lock.lock(); // 计数器减1 int index = --count; if (index == 0) { // 如果计数器为0,执行屏障操作并唤醒等待队列中的所有线程 final Runnable command = barrierCommand; if (command != null) { command.run(); } next trip.signalAll(); } else { try { // 等待其他线程到达屏障处 int phase = generation; trip.await(); // 如果是最后一个到达屏障的线程,执行屏障操作并唤醒等待队列中的所有线程 if (phase == generation) { command = barrierCommand; if (command != null) { command.run(); } } // 计数器重置 nextGeneration(); } catch (InterruptedException ie) { // 如果线程在等待时被中断,抛出InterruptedException异常 cancel(); throw ie; } catch (BrokenBarrierException bbe) { // 如果屏障被破坏,抛出BrokenBarrierException异常 broken = true; trip = new Condition[parties]; throw bbe; } } return index; } finally { // 释放锁 lock.unlock(); } }
In the await() method of CyclicBarrier, first acquire the lock of the ReentrantLock object and decrement the counter by 1. If the counter is 0, perform the barrier operation and wake up all threads in the waiting queue. If the counter is not 0, wait for other threads to arrive at the barrier.
During the waiting process, if the thread is interrupted, an InterruptedException will be thrown. If the barrier is broken, a BrokenBarrierException will be thrown. If it is the last thread to reach the barrier, the barrier operation will be performed and all threads in the waiting queue will be woken up and the counter will be reset to parties.
Usage scenarios of CyclicBarrier
CyclicBarrier is suitable for scenarios where multiple threads need to wait for each other to reach a certain barrier point before continuing to execute. For example, if multiple threads need to execute a certain task at the same time, but a certain task needs to wait for other tasks to complete before it can continue to execute, then you can use CyclicBarrier to achieve thread synchronization and collaboration.
In addition, CyclicBarrier can also be used to implement pipeline processing. For example, in the producer-consumer model, multiple producers can add data to the queue at the same time. When the queue is full, all producers need to wait for consumption. After the user has finished processing the data, continue adding data.
The above is the detailed content of How to use Java multi-thread synchronization tool class CyclicBarrier. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


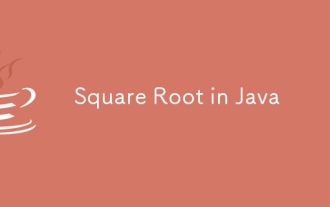
Guide to Square Root in Java. Here we discuss how Square Root works in Java with example and its code implementation respectively.
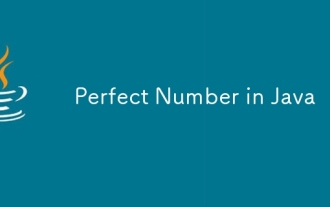
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
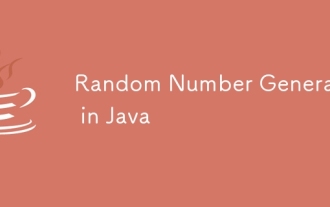
Guide to Random Number Generator in Java. Here we discuss Functions in Java with examples and two different Generators with ther examples.
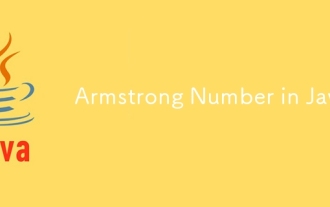
Guide to the Armstrong Number in Java. Here we discuss an introduction to Armstrong's number in java along with some of the code.
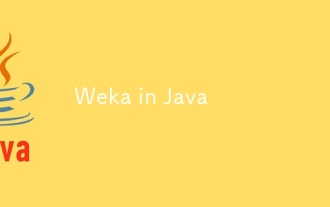
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
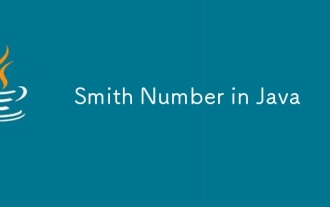
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
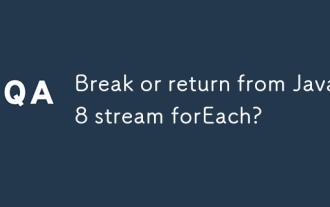
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
