How to write golang naming
In Golang, naming rules are very important because they directly affect the reading, writing and maintainability of the code. Therefore, writing good naming in Golang is important, it can make the code easier to read, understand and maintain. This article will introduce the rules and best practices of naming in Golang.
Naming of functions, variables and constants
In Golang, functions, variables and constants should be named using camel case (CamelCase), that is, the first letter of the first word is lowercase, and the others are lowercase. Capitalize the first letter of a word. In addition to this, variables should be named descriptively, clearly expressing their purpose. For example:
func calculateSum(x int, y int) int { return x + y } var firstName string = "John" var lastName string = "Doe" const Pi = 3.14159
Note: It is not recommended to use underscores (_) as variable or function names in Golang, but it is recommended to use camel case naming. However, if you want to implement some special functions, underscores may be used to ignore unnecessary return values.
Structure and type naming
In Golang, structure and type naming should use PascalCase, that is, the first letter of each word should be capitalized. Likewise, structure and type names should be descriptive and clearly indicate their role and characteristics. For example:
type Person struct { Name string Age int Gender string }
In the above example, we defined a structure type named Person, which contains three fields: Name, Age and Gender. The name directly expresses the role of this type (a "person").
Interface naming
Similar to structure and type names, in Golang, the naming of interfaces should follow PascalCase. At the same time, the interface name should end with "er" to clearly indicate its main function. For example:
type Reader interface { Read(p []byte) (n int, err error) } type Writer interface { Write(p []byte) (n int, err error) } type Closer interface { Close() error }
In the above example, we defined three interfaces: Reader, Writer and Closer. These names all end with "er" and clearly express the main role of the interface.
Package name naming
In Golang, the package name should be a short, descriptive name that can clearly indicate the role of the code it contains. It is recommended to use lowercase letters and avoid uppercase letters. For example:
package utils import "fmt" func SayHello() { fmt.Println("Hello, world!") }
In the above example, we created a package called utils. This package contains the SayHello function. When this function is called, the "Hello, world!" message will be printed on the console.
Summary
In Golang, naming rules have an extremely important impact on the readability and maintainability of the code. This article explains the rules and best practices for writing good names in Golang. Whether you are writing functions, variables and constants, or defining structures, types and interfaces, you should follow the above naming rules and try to make your code easier to understand, maintain and extend.
The above is the detailed content of How to write golang naming. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
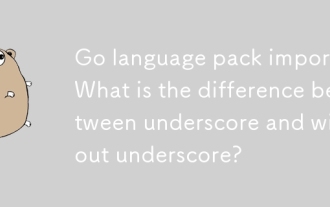
This article explains Go's package import mechanisms: named imports (e.g., import "fmt") and blank imports (e.g., import _ "fmt"). Named imports make package contents accessible, while blank imports only execute t

This article explains Beego's NewFlash() function for inter-page data transfer in web applications. It focuses on using NewFlash() to display temporary messages (success, error, warning) between controllers, leveraging the session mechanism. Limita
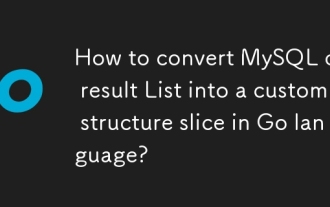
This article details efficient conversion of MySQL query results into Go struct slices. It emphasizes using database/sql's Scan method for optimal performance, avoiding manual parsing. Best practices for struct field mapping using db tags and robus
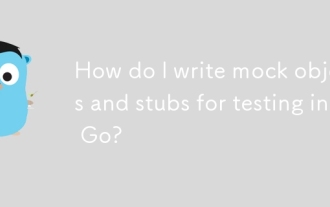
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
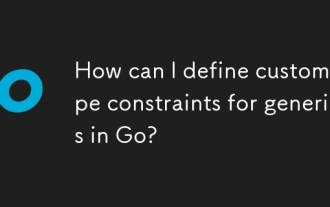
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
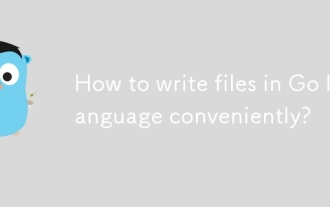
This article details efficient file writing in Go, comparing os.WriteFile (suitable for small files) with os.OpenFile and buffered writes (optimal for large files). It emphasizes robust error handling, using defer, and checking for specific errors.
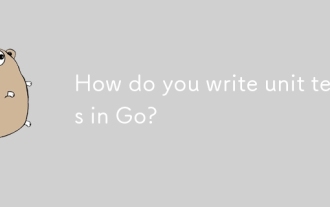
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
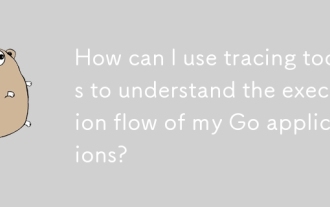
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
