nodejs dynamically export multiple methods
In Node.js, a JavaScript module usually exports only one method or object. But in some cases, we may need to export multiple methods from the same module. In this case we can use dynamic export method. This article will introduce how to dynamically export multiple methods in Node.js.
- What is dynamic export?
Dynamic export refers to exposing the members of the module to the outside as needed at runtime. This technique typically uses a factory function in the module's code, which returns an object that contains the module's public interface. This allows dynamically exported methods to be added or removed as needed.
- How to implement dynamic export
There are many ways to implement dynamic export. This article will introduce two common ways: using ES6 modules and CommonJS modules.
2.1 Using ES6 modules
In ES6 modules, we can use named exports to dynamically export multiple methods.
For example, suppose we have a module called "utils.js", which has two exportable methods foo and bar:
// utils.js export const foo = () => console.log('foo'); export const bar = () => console.log('bar');
Now, we can create a factory function, according to Different properties in the utils object need to be returned:
// index.js import * as utils from './utils'; function getUtils() { return { foo: utils.foo, bar: utils.bar }; } // 在运行时使用工厂函数 const myUtils = getUtils(); myUtils.foo(); // 打印 "foo" myUtils.bar(); // 打印 "bar"
In the above code example, we use the getUtils function to dynamically create the myUtils object and export the foo and bar methods from the utils.js module.
2.2 Using CommonJS module
In the CommonJS module, we can use module.exports to dynamically export multiple methods.
For example, suppose we have a module called "utils.js", which has two exportable methods foo and bar:
// utils.js function foo() { console.log('foo'); } function bar() { console.log('bar'); } // 将方法导出到exports对象中 exports.foo = foo; exports.bar = bar;
Now, we can create a factory function, according to Different properties in the utils object need to be returned:
// index.js const utils = require('./utils'); function getUtils() { return { foo: utils.foo, bar: utils.bar }; } // 在运行时使用工厂函数 const myUtils = getUtils(); myUtils.foo(); // 打印 "foo" myUtils.bar(); // 打印 "bar"
In the above code example, we use the require function to import the utils.js module and use the getUtils function to dynamically create the myUtils object.
- Advantages and Disadvantages of Dynamic Export
The advantage of dynamic export is that members of the module can be exposed to the outside as needed. Dynamic exports can be very useful if the members our module needs to expose are unspecified. In addition, dynamic export can also maximize code reusability.
However, the disadvantage of dynamic export is that it may confuse code readers. Without proper comments and documentation, code readers may not know which methods can be dynamically exported at runtime. Therefore, we need to follow best practices and use explicit exports where possible.
- Summary
In Node.js, dynamic exports can expose members of a module to the outside as needed. We can dynamically create objects containing the module's public interface through factory functions. In addition, we can also dynamically export multiple methods using the named export method in ES6 modules and CommonJS modules. While dynamic exports have some advantages, if not used appropriately, they can lead to confusing code readability. Therefore, we should follow best practices and use explicit exports where possible.
The above is the detailed content of nodejs dynamically export multiple methods. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
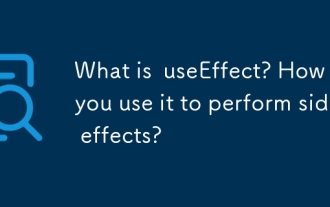
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.

Lazy loading delays loading of content until needed, improving web performance and user experience by reducing initial load times and server load.

Higher-order functions in JavaScript enhance code conciseness, reusability, modularity, and performance through abstraction, common patterns, and optimization techniques.
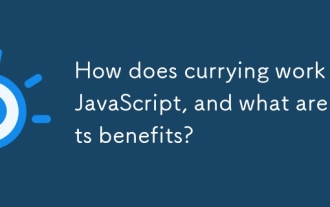
The article discusses currying in JavaScript, a technique transforming multi-argument functions into single-argument function sequences. It explores currying's implementation, benefits like partial application, and practical uses, enhancing code read
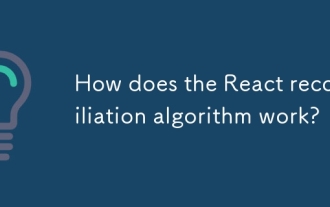
The article explains React's reconciliation algorithm, which efficiently updates the DOM by comparing Virtual DOM trees. It discusses performance benefits, optimization techniques, and impacts on user experience.Character count: 159
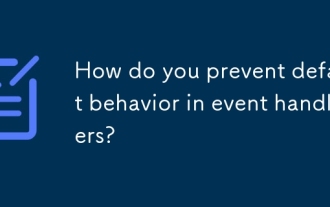
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.
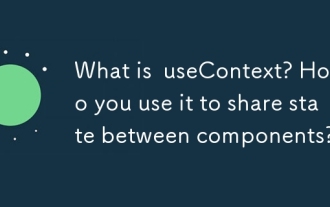
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
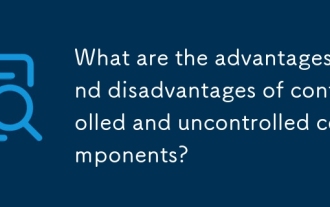
The article discusses the advantages and disadvantages of controlled and uncontrolled components in React, focusing on aspects like predictability, performance, and use cases. It advises on factors to consider when choosing between them.
