How to use the Python file system module pathlib library
1. Official definition of pathlib library
pathlib is a built-in library of Python. The definition given by the Python documentation is Object-oriented filesystem paths. pathlib provides classes representing file system paths, with semantics applicable to different operating systems.
The path classes are divided between pure paths, which provide pure computational operations without I/O, and concrete paths, which inherit pure paths but also provide I/O operations.
2. Pathlib cheats
1. Basic usage
Path.iterdir() # Traverse the subdirectories of the directory or File
Path.is_dir() # Determine whether it is a directory
- ##Path.
glob () # Filter directory (return to generator)
##Path. - resolve
() # Return absolute path
Path . - exists
() # Determine whether the path exists
Path. - open
() # Open the file (supports with)
Path. - unlink
() # Delete a file or directory (the directory is not empty and triggers an exception)
2. Basic attributes
- Path.
- parts
# Splitting the path is similar to os.path.split(), but returns a tuple
Path. - drive
# Returns the drive name
Path. - root
# Returns the root directory of the path
Path. - anchor
# Automatically determine and return drive or root
Path. - parents
# Return a list of all parent directories
3. Change path
- Path.
- with_name
() # Change the path name, change the last level path name
Path. - with_suffix
() # Change path suffix
4, Splice path
- Path.
- joinpath
() #Join path
Path. - relative_to
() #Calculate relative path
##5 , Test path
- match
- () # Test whether the path matches pattern
Path.
is_dir - () # Whether it is a file
##Path.
is_absolute () # Whether it is an absolute path -
Path .
is_reserved () # Whether it is a reserved path -
Path.
exists () # Determine whether the path actually exists 6. Other methods
Path.
cwd- () # Return the path object of the current directory
-
Path.
home () # Return the current user’s home path object -
Path.
stat () # Return path information, Same as os.stat() -
Path.
chmod () # Change path permissions, similar to os.chmod() -
Path.
expanduser () # Expand~Return full path object -
Path.
mkdir () # Create directory -
Path.
rename () # Rename the path -
Path.
rglob () # Recursively traverse all Files in subdirectories 3. The difference between os and pathlib.Path
Compared with the path method of the os module, the Path of the Python3 standard library pathlib module operates on the path. It will be simpler.
1. Get the current file path
When using the os module, you can directly get the current file path through the
getcwd()method
In Pycharm, You can use os.path.dirname(__file__)
to get the current file path, because Python does not provide the concept of __file__, which is provided by Pycharm:
import os print(os.getcwd()) # C:\Users\bobin.yang\PycharmProjects\untitled print(os.path.dirname(__file__)) # C:/Users/bobin.yang/PycharmProjects/untitled
In the pathlib module, pass Path.cwd()
method can directly obtain the current file path, we can give it a try:
import pathlib print(pathlib.Path.cwd()) # C:\Users\bobin.yang\PycharmProjects\untitled
Through Pycharm we can use shortcut keys ctrl left mouse button
Click to view a detailed introduction to this method.
As can be seen from the above picture, the cwd()
method is just an encapsulation of the
method in the os library. It looks even worse. But the official launch is definitely not a fiction. Now let us reveal the secret together. 2. Get the upper/upper directory
The above case seems to make the use of the pashlib library more complicated. In order to reveal the secret, we can only feel the composition of the pathlib library and understand pathlib How libraries bring us convenience.
In the os module, if we want to get the parent directory of a certain file, the os module is written as:
import os print(os.path.dirname(os.path.dirname(os.getcwd()))) # C:\PycharmProjects
In the pathlib library, it can be achieved in this simple way:
import pathlib print(pathlib.Path.cwd().parent) # C:\PycharmProjects
And if you need to find his grandfather, just ask for another
.parent. Compared with the os module's
os.path.dirname(), is it too convenient? 3. Path splicing
If you want to splice the path in its parent directory, you may need to write such a long string of code through the os module:
import os print(os.path.join(os.path.dirname(os.getcwd()), '路径拼接', '真麻烦')) # C:\PycharmProjects\路径拼接\真麻烦
When you use pathlib, let’s experience its convenience together!
# /Users/mac/test.py import os paths = ('路径拼接', '真麻烦') print(pathlib.Path.cwd().parent.joinpath(*paths)) # C:\PycharmProjects\路径拼接\真麻烦
通过 pathlib 库拼接路径,你可以很方便的调节他在他祖辈的位置,妙哉。
4、 其他封装
pathlib 封装了很多的 os.path 中的方法,如下所示:
os.path.expanduser() --> pathlib.Path.home()
os.path.expanduser() --> pathlib.Path.expanduser()
os.stat() --> pathlib.Path.stat()
os.chmod() --> pathlib.Path.chmod()
四、pathlib.PurePath的使用
上一节的操作大部分都是通过 pathlib 库中的 Path 实现,其实他还有一个更加高大上的模块,也就是我们这一节的主角:pathlib.PurePath
。
PurePath 是一个纯路径对象,纯路径对象提供了实际上不访问文件系统的路径处理操作。
有三种方法可以访问这些类,我们也称之为 flavor 。
1、 PurePath.match
下面让我们来实现一个神奇的功能,判断当前的路径下是否有符合'*.py'规则的文件。
import pathlib print(pathlib.PurePath(__file__).match('*.py')) # True
输出为什么会是 True
呢?因为当前文件夹下不就有一个 test.py
吗?
2、 PurePath的子类:PurePosixPath(非Windows系统)、PureWindowsPath
看见 pathlib.PurePath 后面跟着 match,那是不是能说明他是个对象,而不是一个单纯的路径字符串,因此我们可以试着打印 pathlib.PurePath 看一看。
import pathlib os_path = os.path.dirname(__file__) print(os_path) # C:/PycharmProjects/untitled pure_path = pathlib.PurePath(__file__) print(pure_path) # C:\PycharmProjects\untitled\run.py print(type(pure_path)) # <class 'pathlib.PureWindowsPath'> print(pathlib.PurePath(__file__).match('*.py')) # True
通过打印 os.path 获取当前路径的结果,得到一个路径字符串;而通过 pathlib.PurePath 则获得了一个 PurePosixPath 对象,并且由此得到的路径包括了当前文件 run.py。
The above is the detailed content of How to use the Python file system module pathlib library. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
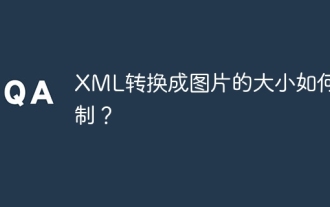
To generate images through XML, you need to use graph libraries (such as Pillow and JFreeChart) as bridges to generate images based on metadata (size, color) in XML. The key to controlling the size of the image is to adjust the values of the <width> and <height> tags in XML. However, in practical applications, the complexity of XML structure, the fineness of graph drawing, the speed of image generation and memory consumption, and the selection of image formats all have an impact on the generated image size. Therefore, it is necessary to have a deep understanding of XML structure, proficient in the graphics library, and consider factors such as optimization algorithms and image format selection.
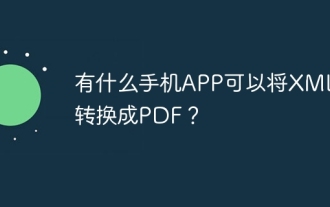
An application that converts XML directly to PDF cannot be found because they are two fundamentally different formats. XML is used to store data, while PDF is used to display documents. To complete the transformation, you can use programming languages and libraries such as Python and ReportLab to parse XML data and generate PDF documents.
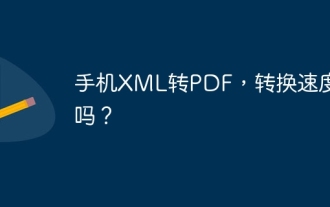
The speed of mobile XML to PDF depends on the following factors: the complexity of XML structure. Mobile hardware configuration conversion method (library, algorithm) code quality optimization methods (select efficient libraries, optimize algorithms, cache data, and utilize multi-threading). Overall, there is no absolute answer and it needs to be optimized according to the specific situation.
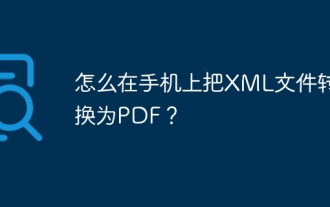
It is impossible to complete XML to PDF conversion directly on your phone with a single application. It is necessary to use cloud services, which can be achieved through two steps: 1. Convert XML to PDF in the cloud, 2. Access or download the converted PDF file on the mobile phone.
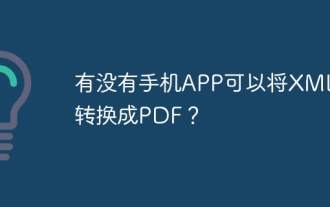
There is no APP that can convert all XML files into PDFs because the XML structure is flexible and diverse. The core of XML to PDF is to convert the data structure into a page layout, which requires parsing XML and generating PDF. Common methods include parsing XML using Python libraries such as ElementTree and generating PDFs using ReportLab library. For complex XML, it may be necessary to use XSLT transformation structures. When optimizing performance, consider using multithreaded or multiprocesses and select the appropriate library.
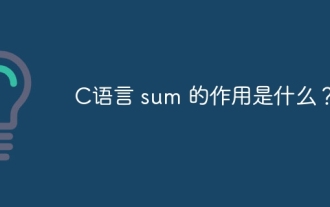
There is no built-in sum function in C language, so it needs to be written by yourself. Sum can be achieved by traversing the array and accumulating elements: Loop version: Sum is calculated using for loop and array length. Pointer version: Use pointers to point to array elements, and efficient summing is achieved through self-increment pointers. Dynamically allocate array version: Dynamically allocate arrays and manage memory yourself, ensuring that allocated memory is freed to prevent memory leaks.
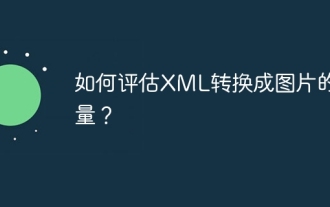
The quality evaluation of XML to pictures involves many indicators: Visual fidelity: The picture accurately reflects XML data, manual or algorithm evaluation; Data integrity: The picture contains all necessary information, automated test verification; File size: The picture is reasonable, affecting loading speed and details; Rendering speed: The image is generated quickly, depending on the algorithm and hardware; Error handling: The program elegantly handles XML format errors and data missing.
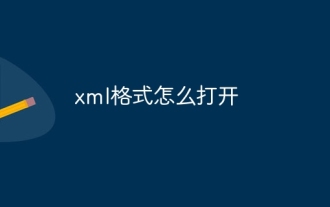
Use most text editors to open XML files; if you need a more intuitive tree display, you can use an XML editor, such as Oxygen XML Editor or XMLSpy; if you process XML data in a program, you need to use a programming language (such as Python) and XML libraries (such as xml.etree.ElementTree) to parse.
