Using jquery to implement paging query data
With the continuous development of Web technology, more and more websites need to support the function of paging data query. jQuery is a very popular JavaScript library that can help developers more conveniently operate DOM, events, animations, etc., so using jQuery to implement paging query data is a good choice.
This article will introduce the basic principles, steps and code implementation methods of using jQuery to implement paging query data, and provide a simple example for readers' reference.
1. Basic Principle
The basic principle of using jQuery to implement paging query data is to send an asynchronous request to the background through AJAX technology, obtain the data that needs to be displayed, and display it on the page. During the implementation process, the following technologies need to be used:
- AJAX technology: Use jQuery's AJAX method to send asynchronous requests to the background to obtain the data that needs to be displayed.
- Paging algorithm: Calculate the data to be displayed in the background, and then determine the starting position and quantity of the data to be displayed based on the current page number and the number of records displayed on each page.
- HTML, CSS and JavaScript: Use jQuery to operate DOM elements, dynamically generate paging controls, and implement paging functions.
2. Steps
The following are the basic steps to use jQuery to implement paging query data:
- Define a DIV element to display the data that needs to be queried data and set an ID for the element.
- Define a JavaScript function to send an asynchronous request to the background and display the obtained data in the specified DIV element.
- Define a JavaScript function to generate a paging control and set event listeners for each button of the paging control.
- After the page is loaded, call the above two functions to display the data and paging controls of the first page.
- When the user clicks the button on the paging control, the first function is called to obtain the data of the specified page number and display it in the specified DIV element.
3. Code Implementation
The following is a simple example code that uses jQuery to implement paging query data:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>分页查询数据示例</title> <script src="https://cdn.bootcss.com/jquery/3.3.1/jquery.min.js"></script> <script src="paging.js"></script> <link rel="stylesheet" href="paging.css"> </head> <body> <div id="data"></div> <div id="paging"></div> <script> $(document).ready(function() { // 显示第一页数据和分页控件 getDataWithPage(1); generatePaging(1); // 为分页控件上的按钮添加事件监听器 $('#paging').on('click', '.page-btn', function() { var page = parseInt($(this).data('page')); getDataWithPage(page); generatePaging(page); }); }); </script> </body> </html>
/* * 分页查询数据相关的 JavaScript 函数 */ var PAGE_SIZE = 10; // 每页显示的记录数 var TOTAL_PAGES = 20; // 总页数(假设为 20) // 向后台发送异步请求,获取指定页码的数据,并将其显示在指定的 DIV 元素中 function getDataWithPage(page) { var startIndex = (page - 1) * PAGE_SIZE + 1; var endIndex = startIndex + PAGE_SIZE - 1; $.ajax({ url: 'data.php', // 后台数据接口 URL method: 'GET', data: { startIndex: startIndex, endIndex: endIndex }, success: function(data) { // 将获取到的数据显示在指定的 DIV 元素中 $('#data').html(data); }, error: function() { alert('获取数据失败'); } }); } // 生成分页控件,并为分页控件的每个按钮设置事件监听器 function generatePaging(currentPage) { var pagingHTML = '<ul>'; if (currentPage == 1) { pagingHTML += '<li><span class="disabled">上一页</span></li>'; } else { pagingHTML += '<li><a href="javascript:void(0);" class="page-btn" data-page="' + (currentPage - 1) + '">上一页</a></li>'; } for (var i = 1; i <= TOTAL_PAGES; i++) { if (i === currentPage) { pagingHTML += '<li><span class="current">' + i + '</span></li>'; } else { pagingHTML += '<li><a href="javascript:void(0);" class="page-btn" data-page="' + i + '">' + i + '</a></li>'; } } if (currentPage == TOTAL_PAGES) { pagingHTML += '<li><span class="disabled">下一页</span></li>'; } else { pagingHTML += '<li><a href="javascript:void(0);" class="page-btn" data-page="' + (currentPage + 1) + '">下一页</a></li>'; } pagingHTML += '</ul>'; $('#paging').html(pagingHTML); }
/* * 分页控件相关的 CSS 样式 */ #paging ul { margin: 0; padding: 0; list-style-type: none; } #paging ul li { display: inline-block; margin: 0 5px; padding: 0; } #paging ul li span { display: inline-block; padding: 5px 15px; border: 1px solid #ddd; background-color: #fff; color: #333; cursor: default; } #paging ul li a { display: inline-block; padding: 5px 15px; border: 1px solid #ddd; background-color: #fff; color: #333; text-decoration: none; } #paging ul li a:hover { background-color: #f5f5f5; } #paging ul li .current { display: inline-block; padding: 5px 15px; border: 1px solid #ddd; background-color: #f5f5f5; color: #333; cursor: default; } #paging ul li .disabled { display: inline-block; padding: 5px 15px; border: 1px solid #ddd; background-color: #fff; color: #bbb; cursor: default; }
It should be noted that the above code is a A simple example, which needs to be adjusted and optimized according to actual conditions in actual applications. At the same time, it is also necessary to ensure the normal operation and data security of the background data interface to avoid SQL injection and other attacks.
The above is the detailed content of Using jquery to implement paging query data. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics



React combines JSX and HTML to improve user experience. 1) JSX embeds HTML to make development more intuitive. 2) The virtual DOM mechanism optimizes performance and reduces DOM operations. 3) Component-based management UI to improve maintainability. 4) State management and event processing enhance interactivity.
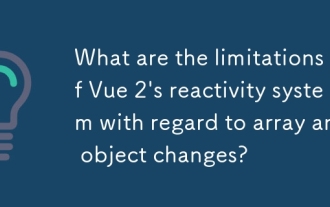
Vue 2's reactivity system struggles with direct array index setting, length modification, and object property addition/deletion. Developers can use Vue's mutation methods and Vue.set() to ensure reactivity.

React components can be defined by functions or classes, encapsulating UI logic and accepting input data through props. 1) Define components: Use functions or classes to return React elements. 2) Rendering component: React calls render method or executes function component. 3) Multiplexing components: pass data through props to build a complex UI. The lifecycle approach of components allows logic to be executed at different stages, improving development efficiency and code maintainability.
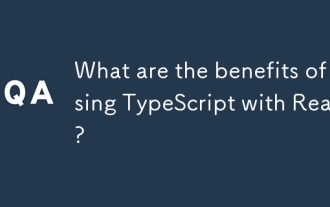
TypeScript enhances React development by providing type safety, improving code quality, and offering better IDE support, thus reducing errors and improving maintainability.

React is the preferred tool for building interactive front-end experiences. 1) React simplifies UI development through componentization and virtual DOM. 2) Components are divided into function components and class components. Function components are simpler and class components provide more life cycle methods. 3) The working principle of React relies on virtual DOM and reconciliation algorithm to improve performance. 4) State management uses useState or this.state, and life cycle methods such as componentDidMount are used for specific logic. 5) Basic usage includes creating components and managing state, and advanced usage involves custom hooks and performance optimization. 6) Common errors include improper status updates and performance issues, debugging skills include using ReactDevTools and Excellent
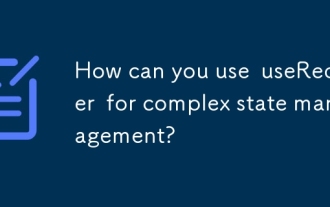
The article explains using useReducer for complex state management in React, detailing its benefits over useState and how to integrate it with useEffect for side effects.
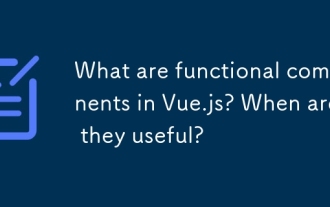
Functional components in Vue.js are stateless, lightweight, and lack lifecycle hooks, ideal for rendering pure data and optimizing performance. They differ from stateful components by not having state or reactivity, using render functions directly, a
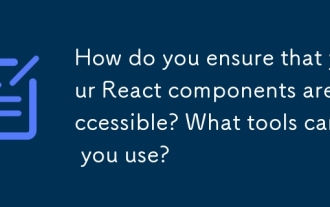
The article discusses strategies and tools for ensuring React components are accessible, focusing on semantic HTML, ARIA attributes, keyboard navigation, and color contrast. It recommends using tools like eslint-plugin-jsx-a11y and axe-core for testi
