Parent class calling syntax in PHP8.0
PHP is a server-side scripting language widely used in Web development, and PHP 8.0 version introduces a new parent class calling syntax to make object-oriented programming more convenient and concise.
In PHP, we can create a parent class and one or more subclasses through inheritance. Subclasses can inherit the properties and methods of the parent class, and can modify or extend their functionality by overriding the methods of the parent class.
In ordinary PHP inheritance, if we want to call the method of the parent class in the subclass, we need to use the parent keyword to refer to the method of the parent class:
class ParentClass { public function parentMethod() { echo "Hello from parent method "; } } class ChildClass extends ParentClass { public function childMethod() { parent::parentMethod(); //使用parent关键字来引用父类方法 echo "Hello from child method "; } } $obj = new ChildClass(); $obj->childMethod(); //输出 Hello from parent method 和 Hello from child method
In the above code, childMethod () method calls the parentMethod() method in ParentClass by using parent::parentMethod(), and outputs the "Hello from child method" string at the end of the method.
In PHP 8.0, we can use a more concise syntax to call the method of the parent class. The new syntax uses the static keyword instead of the parent keyword, for example:
class ParentClass { public static function parentMethod() { echo "Hello from parent method "; } } class ChildClass extends ParentClass { public function childMethod() { static::parentMethod(); //使用static关键字代替parent关键字来引用父类方法 echo "Hello from child method "; } } $obj = new ChildClass(); $obj->childMethod(); //输出 Hello from parent method 和 Hello from child method
In the above code, the parentMethod() method becomes a static method. We can call the parent class by using static::parentMethod() parentMethod() method. This new syntax can make the code clearer and easier to read, and can also avoid some code maintenance problems, because it does not require us to think about class names in the inheritance hierarchy.
In addition to using static methods, we can also use this new syntax in other places. For example, in API calls, we use a structure composed of traits and interfaces to implement functions similar to multiple inheritance. In this case, using this new syntax can express the code intention more clearly:
interface ParentInterface { public function parentMethod(); } trait ParentTrait { public function parentMethod() { echo "Hello from parent trait method "; } } class ChildClass implements ParentInterface { use ParentTrait; public function childMethod() { static::parentMethod(); //使用新语法来调用父类trait中的方法 echo "Hello from child method "; } } $obj = new ChildClass(); $obj->childMethod(); //输出 Hello from parent trait method 和 Hello from child method
In this example, we define a ParentInterface and a ParentTrait, these two structures are implemented through ChildClass ( use) to form a structure similar to multiple inheritance. Then, in childMethod() of ChildClass, we use the new syntax to call the parentMethod() method in ParentTrait. This approach makes the code more concise and easier to understand.
To summarize, in PHP 8.0, we can use the new parent class calling syntax, that is, use the static keyword instead of the parent keyword to express the calling of the parent class method more clearly and concisely. This feature can make code more readable and easier to understand in many scenarios. Understanding and mastering this new feature is crucial for PHP developers.
The above is the detailed content of Parent class calling syntax in PHP8.0. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
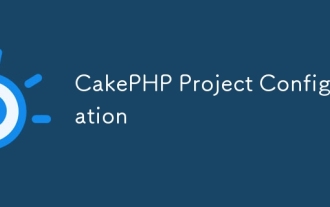
In this chapter, we will understand the Environment Variables, General Configuration, Database Configuration and Email Configuration in CakePHP.
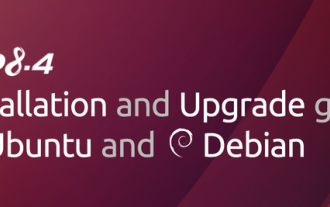
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
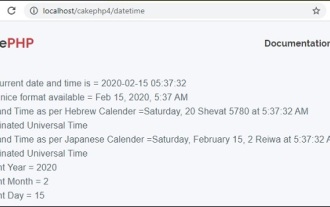
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
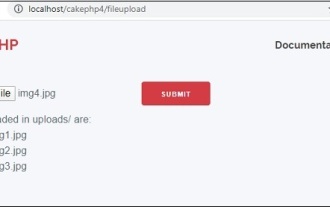
To work on file upload we are going to use the form helper. Here, is an example for file upload.
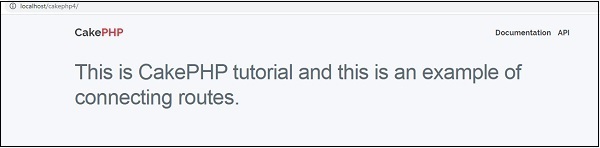
In this chapter, we are going to learn the following topics related to routing ?
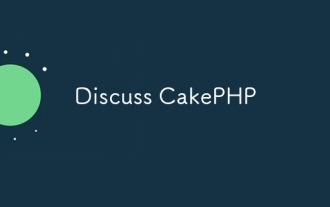
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
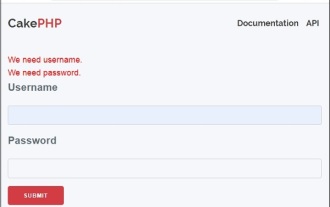
Validator can be created by adding the following two lines in the controller.
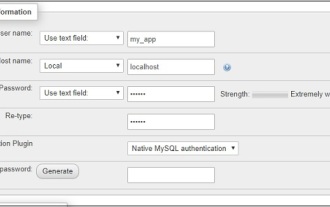
Working with database in CakePHP is very easy. We will understand the CRUD (Create, Read, Update, Delete) operations in this chapter.
