How to switch animation between elements and components in Vue3
Example analysis
Animation switching between elements
The animation switching between elements refers to two dom# Switching between ## elements, for example, one
div disappears and another
div is displayed. The disappearance corresponds to the fade-out effect, and the display corresponds to the fade-in effect. In this example, we use two
div, one displays
hello world, the other displays
bye world, and then uses a button to control the switching of the animation. The code is as follows:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>元素切换动画的实现</title> <style> .v-enter-from{ opacity: 0; } .v-enter-active{ transition: opacity 1s ease-in; } .v-enter-to{ opacity: 1; } .v-leave-from{ opacity: 1; } .v-leave-active{ transition:opacity 1s ease-in } .v-leave-to{ opacity: 0; } </style> <script src="https://unpkg.com/vue@next"></script> </head> <body> <div id="root"></div> </body> <script> const app = Vue.createApp({ data() { return { show:false } }, methods: { handleClick(){ this.show = !this.show; } }, template: ` <transition mode="out-in" appear> <div v-if="show">hello world </div> <div v-else="show" >bye world </div> </transition> <button @click="handleClick">switch</button> ` }); const vm = app.mount('#root'); </script>
div we want to animate into
Boolean
variable show
to control the display and hiding of elements. When we click the button, the handleClick
function is executed to show
The variable is inverted to achieve the switching effect. In the code, we also see that a mode="out-in"
is used on the transition tag. The value of this mode
is actually mode=" in-out"
, the difference between the two is as follows:
: means that when two elements are switched, the current element disappears first and is to be displayed. The element is displayed againmode="in-out"
: Indicates that when two elements are switched, the element to be displayed is displayed first, and the current element disappearsReaders can switch these two elements Try all the attributes and see the effect, the impression will be deeper
In the code we see that there is an attribute appear. This attribute means that when we open the interface in the browser, the animation will be executed. Otherwise, the page will be in There is no animation when loading
Animation switching between components
In Vue, we will use components more. In fact, animation switching can also be implemented between components. Here we You can modify the above example to achieve the animation effect of switching between the above elements in the form of dynamic components. The code is as follows:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>组件间切换动画的实现</title> <style> .v-enter-from{ opacity: 0; } .v-enter-active{ transition: opacity 1s ease-in; } .v-enter-to{ opacity: 1; } .v-leave-from{ opacity: 1; } .v-leave-active{ transition:opacity 1s ease-in } .v-leave-to{ opacity: 0; } </style> <script src="https://unpkg.com/vue@next"></script> </head> <body> <div id="root"></div> </body> <script> // 多个单组件之间的动画 const ComponentA = { template:'<div>hello world</div>' } const ComponentB = { template:'<div>bye world</div>' } const app = Vue.createApp({ data() { return { component:'component-a' } }, methods: { handleClick(){ if(this.component === 'component-a'){ this.component = 'component-b'; }else{ this.component = 'component-a'; } } }, components:{ 'component-a':ComponentA, 'component-b':ComponentB }, // 动态组件的方式 template: ` <transition mode="out-in" appear> <component :is="component" /> </transition> <button @click="handleClick">switch</button> ` }); const vm = app.mount('#root'); </script>
The above is the detailed content of How to switch animation between elements and components in Vue3. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


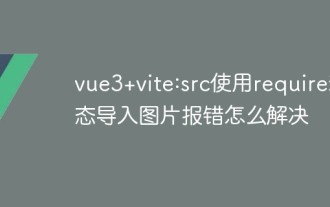
vue3+vite:src uses require to dynamically import images and error reports and solutions. vue3+vite dynamically imports multiple images. If vue3 is using typescript development, require will introduce image errors. requireisnotdefined cannot be used like vue2 such as imgUrl:require(' .../assets/test.png') is imported because typescript does not support require, so import is used. Here is how to solve it: use awaitimport
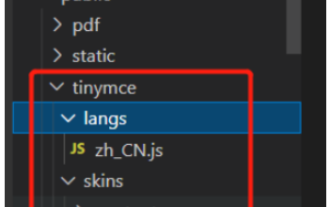
tinymce is a fully functional rich text editor plug-in, but introducing tinymce into vue is not as smooth as other Vue rich text plug-ins. tinymce itself is not suitable for Vue, and @tinymce/tinymce-vue needs to be introduced, and It is a foreign rich text plug-in and has not passed the Chinese version. You need to download the translation package from its official website (you may need to bypass the firewall). 1. Install related dependencies npminstalltinymce-Snpminstall@tinymce/tinymce-vue-S2. Download the Chinese package 3. Introduce the skin and Chinese package. Create a new tinymce folder in the project public folder and download the
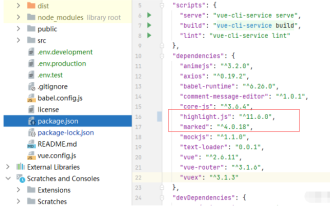
Vue implements the blog front-end and needs to implement markdown parsing. If there is code, it needs to implement code highlighting. There are many markdown parsing libraries for Vue, such as markdown-it, vue-markdown-loader, marked, vue-markdown, etc. These libraries are all very similar. Marked is used here, and highlight.js is used as the code highlighting library. The specific implementation steps are as follows: 1. Install dependent libraries. Open the command window under the vue project and enter the following command npminstallmarked-save//marked to convert markdown into htmlnpmins
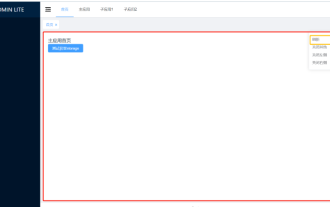
To achieve partial refresh of the page, we only need to implement the re-rendering of the local component (dom). In Vue, the easiest way to achieve this effect is to use the v-if directive. In Vue2, in addition to using the v-if instruction to re-render the local dom, we can also create a new blank component. When we need to refresh the local page, jump to this blank component page, and then jump back in the beforeRouteEnter guard in the blank component. original page. As shown in the figure below, how to click the refresh button in Vue3.X to reload the DOM within the red box and display the corresponding loading status. Since the guard in the component in the scriptsetup syntax in Vue3.X only has o
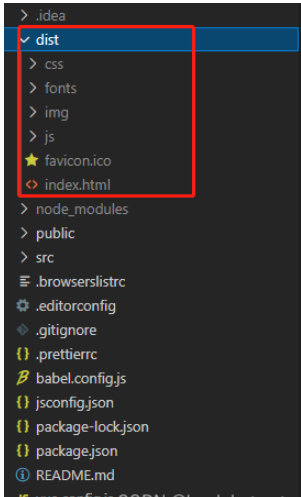
After the vue3 project is packaged and published to the server, the access page displays blank 1. The publicPath in the vue.config.js file is processed as follows: const{defineConfig}=require('@vue/cli-service') module.exports=defineConfig({publicPath :process.env.NODE_ENV==='production'?'./':'/&
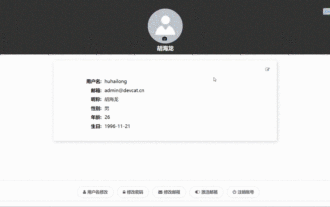
The final effect is to install the VueCropper component yarnaddvue-cropper@next. The above installation value is for Vue3. If it is Vue2 or you want to use other methods to reference, please visit its official npm address: official tutorial. It is also very simple to reference and use it in a component. You only need to introduce the corresponding component and its style file. I do not reference it globally here, but only introduce import{userInfoByRequest}from'../js/api' in my component file. import{VueCropper}from'vue-cropper&
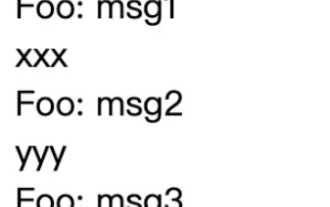
Preface Whether it is vue or react, when we encounter multiple repeated codes, we will think about how to reuse these codes instead of filling a file with a bunch of redundant codes. In fact, both vue and react can achieve reuse by extracting components, but if you encounter some small code fragments and you don’t want to extract another file, in comparison, react can be used in the same Declare the corresponding widget in the file, or implement it through renderfunction, such as: constDemo:FC=({msg})=>{returndemomsgis{msg}}constApp:FC=()=>{return(
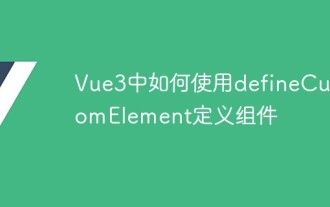
Using Vue to build custom elements WebComponents is a collective name for a set of web native APIs that allow developers to create reusable custom elements (customelements). The main benefit of custom elements is that they can be used with any framework, even without one. They are ideal when you are targeting end users who may be using a different front-end technology stack, or when you want to decouple the final application from the implementation details of the components it uses. Vue and WebComponents are complementary technologies, and Vue provides excellent support for using and creating custom elements. You can integrate custom elements into existing Vue applications, or use Vue to build
