golang tdd development process
With the development of the software industry, development speed and quality have become the focus of pursuit. In order to greatly reduce the cost and time of software development, TDD (Test-Driven Development) emerged as a software development concept. Currently, Go language (Golang) has been one of the main choices for TDD language developers. In this article, we will introduce you to the process of Golang TDD development.
What is TDD?
TDD stands for Test Driven Development. It is a widely used software development method. Its core idea is to write test cases before writing code, then write the smallest code that can pass the test, which is the "green light", and then expand the code to implement more functions. This process continues until the code reaches the desired functionality. TDD plays an increasing role in the software development industry. It can not only improve the quality of software, but also improve development efficiency.
Advantages of Golang TDD development
Golang is a simple and efficient language, and it is also a very popular language. It is widely used to develop web applications and cloud services. Golang was originally designed to achieve high-speed development and have better performance than other languages. When using Golang for TDD development, you will have the following advantages:
Faster testing speed
Golang testing can be completed quickly, and Golang’s speed is much faster than other languages. This allows you to quickly run tests and build and release apps faster.
Fewer dependencies
Golang projects can be written to avoid relying on other libraries and frameworks, which can simplify the build and testing process.
Higher security
Golang’s security is unmatched by other languages. When performing TDD development, enhanced security can ensure the correctness and stability of the code and reduce the occurrence of errors.
Golang TDD development process
Let’s introduce the Golang TDD development process in detail.
Step one: Write test code
In Golang, the test code is usually placed in the same directory as the code being tested, ending with _test.go
. When writing test code, you need to use the testing
library to write unit test code. Let's take a simple addition function as an example.
package main import "testing" func TestAdd(t *testing.T) { sum := Add(1, 2) if sum != 3 { t.Errorf("Add(1, 2) = %d; want 3", sum) } }
In this example, we create a new function named Add
and test it. Test code needs to have a specific Test
prefix, in this example, we wrote a test function named TestAdd
. In the test, we call the Add
function and then compare the result to the expected value.
Step 2: Run the test
Running the test code is one of the key steps in TDD development. In Golang, you can run the test code through the go test
command. During the running process, the _test.go
file will be automatically scanned and the test function whose name is prefixed with Test
will be executed. In this example, we can enter the following command in the terminal to run the test:
$ go test
If the test passes, "PASS" will be output. If the test fails, "FAIL" and specific error information will be output.
Step Three: Write Production Code
After completing the testing in the second step, the third step is to write the production code. Production code is the code we actually want to implement. Still taking the addition function as an example, here we will write the function that passes the test into a real addition function.
func Add(a, b int) int { return a + b }
Step 4: Re-run the tests
After writing the production code, you need to run the tests again to verify that it meets the expected requirements. If the test fails, you need to modify the code and rerun the test until the test passes.
Step 5: Refactor the code
The last step is to refactor the code. When the tests pass and the code runs normally, the code needs to be refactored. Refactoring code is a very important process that makes it more robust and maintainable. During the process of refactoring the code, it is best not to modify the functions or the behavior of the code, but only to optimize the organization of the code to make the code easier to understand and maintain.
Summary
TDD regards testing as an integral part of the development process. Using Golang for TDD development can build applications faster and more securely, reducing errors and discovering defects. time, saving valuable time and resources. By developing according to the above process, you can achieve higher quality code, higher development efficiency, and a more enjoyable development experience.
The above is the detailed content of golang tdd development process. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
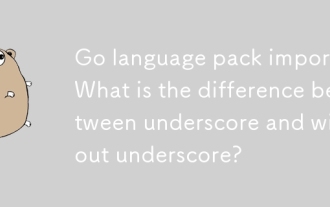
This article explains Go's package import mechanisms: named imports (e.g., import "fmt") and blank imports (e.g., import _ "fmt"). Named imports make package contents accessible, while blank imports only execute t

This article explains Beego's NewFlash() function for inter-page data transfer in web applications. It focuses on using NewFlash() to display temporary messages (success, error, warning) between controllers, leveraging the session mechanism. Limita
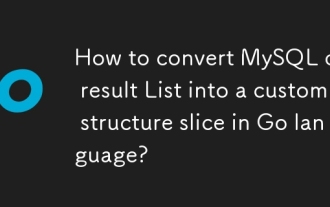
This article details efficient conversion of MySQL query results into Go struct slices. It emphasizes using database/sql's Scan method for optimal performance, avoiding manual parsing. Best practices for struct field mapping using db tags and robus
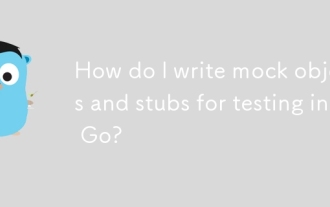
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
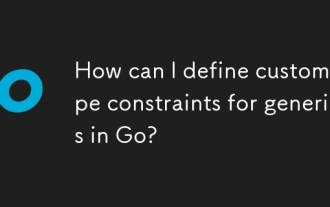
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
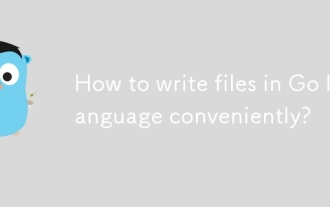
This article details efficient file writing in Go, comparing os.WriteFile (suitable for small files) with os.OpenFile and buffered writes (optimal for large files). It emphasizes robust error handling, using defer, and checking for specific errors.
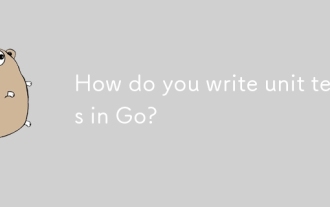
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
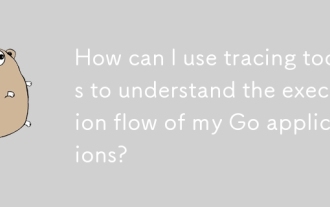
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
