Set up golang daemon
When developing and deploying Go applications, we often need to configure them as daemon processes so that they can run in the background and start automatically when the system starts. This article will explain how to set up a Go application as a daemon so that you can easily control and manage your application.
1. Using systemd
systemd is a daemon system and system manager that can be used to manage and monitor system processes in Linux systems. After installing systemd and configuring your Go application as a Systemd service, you can use the systemctl command to start, stop, and reload your service.
Here are the simple steps on how to configure a Go application as a Systemd service:
- Create the systemd service file
Use your favorite text editor Create a new file in your browser and copy and paste the following into the file:
[Unit]
Description=My Go Service
After=network.target
[Service]
User=
Group=
ExecStart=
Restart=always
[Install]
WantedBy=multi-user.target
Please note to replace the following parameters:
- Description: Description of the service.
- User: The user to run the application.
- Group: The group to which the user of the application belongs.
- ExecStart: The absolute path of the Go application.
- Restart: Automatically restart the service after it is stopped.
2. Save the file as a .service file
Save the file as "mygo.service" and place it in the "/etc/systemd/system" directory. This will enable Systemd to load the service file on startup.
3. Reload Systemd
Run the following command to reload Systemd and make it aware of the new service file:
sudo systemctl daemon-reload
4. Start the service
Run the following command to start the service:
sudo systemctl start mygo.service
5.Check the service status
Run the following command To check if your service is running:
sudo systemctl status mygo.service
If the status is "running", it means the service is running.
2. Using supervisor
Supervisor is an application that manages and monitors processes on Unix systems. It can easily manage your Go applications and also supports features such as automatic restart and logging output.
Here are the steps on how to set up Supervisor to guard Go applications on Ubuntu:
- Install Supervisor
sudo apt-get install supervisor
2. Create Supervisor Configuration
Use your favorite text editor to create a new file and copy and paste the following content into the file:
[program:mygo]
command=/usr/bin/go run /path/to/your/go/app
user=
autostart=true
autorestart=true
stderr_logfile=/var/log /mygo.err.log
stdout_logfile=/var/log/mygo.out.log
Please note to replace the following parameters:
- program: The name of the Supervisor program.
- command: The absolute path to the Go application.
- user: The user to run the application.
- stderr_logfile: The file path for recording error logs.
- stdout_logfile: The file path for recording standard output logs.
3. Save the file as a .conf file
Save the file as "mygo.conf" and place it in the "/etc/supervisor/conf.d" directory middle.
4. Reload Supervisor
Run the following command to reload Supervisor and make it aware of the new supervisor service file:
sudo supervisorctl reread
5. Start the service
Run the following command to start the service:
sudo supervisorctl start mygo
6. Check the service status
Run the following command to check your Whether the service is running:
sudo supervisorctl status mygo
If the status is "running", it means the service is running.
Summary
Whether you choose to use systemd or Supervisor, they are powerful tools for managing and monitoring Linux processes. Configuring Go applications as daemons using these tools helps you conveniently control and manage your applications and ensure that they start automatically at system startup.
The above is the detailed content of Set up golang daemon. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
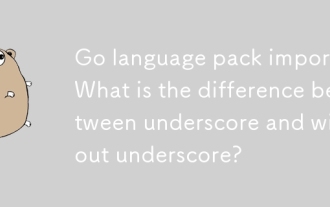
This article explains Go's package import mechanisms: named imports (e.g., import "fmt") and blank imports (e.g., import _ "fmt"). Named imports make package contents accessible, while blank imports only execute t

This article explains Beego's NewFlash() function for inter-page data transfer in web applications. It focuses on using NewFlash() to display temporary messages (success, error, warning) between controllers, leveraging the session mechanism. Limita
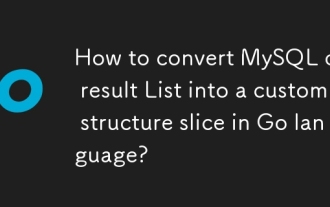
This article details efficient conversion of MySQL query results into Go struct slices. It emphasizes using database/sql's Scan method for optimal performance, avoiding manual parsing. Best practices for struct field mapping using db tags and robus
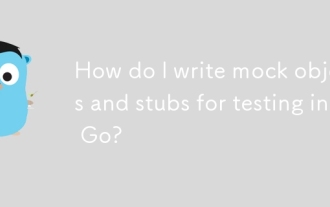
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
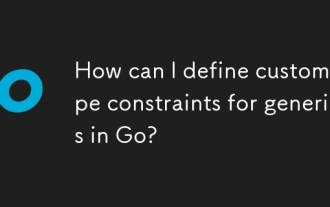
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
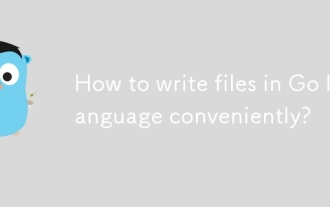
This article details efficient file writing in Go, comparing os.WriteFile (suitable for small files) with os.OpenFile and buffered writes (optimal for large files). It emphasizes robust error handling, using defer, and checking for specific errors.
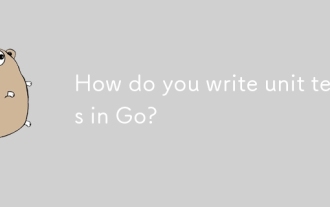
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
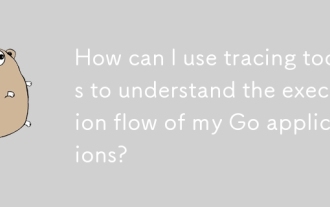
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
