golang function is not compatible
Golang, as a modern programming language, has been widely recognized and welcomed for its simplicity, efficiency, and security. Among them, functions are one of the most basic and important components in Golang, but during use, we may encounter function incompatibility issues. This article will introduce the function incompatibility problem in Golang in detail, explore its causes and solutions.
1. Definition and characteristics of functions
Before starting to introduce the problem of function incompatibility, we need to first understand the definition and characteristics of functions in Golang.
Function is one of the most basic components in Golang, which can perform a specific task and return the result. In Golang, functions can be defined in the following way:
func function_name(parameter1 type1, parameter2 type2) return_type {
// Function content
}
Where:
- function_name: function name;
- parameter1, parameter2: parameter name, there can be multiple parameters;
- type1, type2: parameter type, each parameter needs to specify a type ;
- return_type: return value type, can be empty;
The function can be called, the calling method is as follows:
function_name(parameter1, parameter2)
The characteristics of Golang functions are as follows:
- Function is a first-class citizen, that is, the function can be transferred, assigned and other operations like other variables;
- Supports multiple return values , can return multiple values at the same time;
- supports variable parameters, and the last parameter in the parameter list is represented by "...";
- can also be used as a method;
2. Function incompatibility
Function incompatibility refers to the problem of function type mismatch or inability to be called during program compilation or running. Specifically, it is usually divided into the following situations:
- Parameter type mismatch
When a function is called, the parameter type passed does not match the parameter type defined by the function. Matching will result in the function being unable to be called normally. For example:
func add(a int, b int) int { return a + b } func main() { add(1, "2") }
In this example, the function add expects two integer parameters, but an integer and a string are passed in when called, which will cause a compilation error.
- Number of parameters does not match
When a function is called, the number of parameters passed does not match the number of parameters defined by the function, which will also cause the function to fail to be called normally. For example:
func add(a int, b int) int { return a + b } func main() { add(1) }
In this example, the function add expects two integer parameters to be passed in, but only one integer is passed in when called, which will cause a compilation error.
- Return value type mismatch
When a function is called, when the type returned by the function does not match the return type expected by the caller, it will also cause the function to fail to be called normally. . For example:
func add(a int, b int) string { return strconv.Itoa(a + b) } func main() { result := add(1, 2) fmt.Println(result + 1) }
In this example, the function add returns a string type, but an integer is used to operate the string when called, which will cause a compilation error.
3. Reasons for function incompatibility
The main reasons for function incompatibility include the following aspects:
- The function parameter transfer type does not match
Mismatch in function parameter transfer types is the main reason for function incompatibility. Because in Golang, all parameters must be passed when a function is called, and each parameter must match the parameter type defined by the function.
- The function return value type does not match
When the function return value type does not match, it will cause the function to fail to be called or compile errors. Therefore, you need to explicitly specify the return value type when writing a function, and ensure that the return value type matches the type expected by the caller.
- The function signature of the function does not match
The function signature refers to the parameter list and return value type of the function. In Golang, the function signature is part of the function type. When the function signatures do not match, it will result in the function not being called or compilation errors.
4. How to solve the problem of function incompatibility
When we encounter the problem of function incompatibility, we can use the following methods to solve it:
- Modify the function Definition
If the function parameter type, return value type, or number of parameters do not match, you can solve the problem by modifying the function definition according to actual needs. For example, you can modify the function parameter types, return value type, or number of parameters to match the types expected by the caller.
- Convert a function to a universal function
Converting a function to a universal function can make the function applicable to more situations. For example, changing the parameter type of a function to the interface{} type can accept any type of parameters, perform type conversion within the function and return the corresponding result.
- Using type assertions
Type assertions can determine whether the data type stored in the interface is the specified type and return its value. By using type assertions, you can solve the problem of function parameter type mismatch. For example:
func printValue(v interface{}) { switch v.(type) { case int: fmt.Println(v.(int)) case string: fmt.Println(v.(string)) default: fmt.Println("unknown type") } } func main() { printValue("hello") printValue(123) }
In this example, the function accepts parameters of type interface{}, determines the specific type of the parameter through type assertion and prints out the corresponding value.
- Use function callbacks
Passing a function as a parameter to another function can solve the problem of function signature mismatch. For example:
func processNumber(f func(int) int, num int) int { return f(num) } func multiplyBy2(num int) int { return num * 2 } func main() { result := processNumber(multiplyBy2, 4) fmt.Println(result) }
在这个例子中,函数processNumber接受一个函数作为参数,通过调用该函数来处理传入的数值。
五、总结
函数是Golang中最基础、最重要的组件之一,在Golang中使用函数不兼容的问题可能会导致程序无法编译或异常退出。本文对函数不兼容的原因和解决方法进行了详细的介绍,希望能够帮助读者更好地理解Golang函数的使用。
The above is the detailed content of golang function is not compatible. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




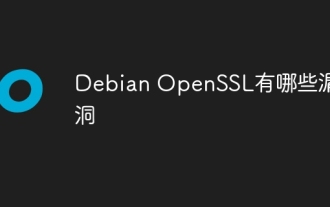
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
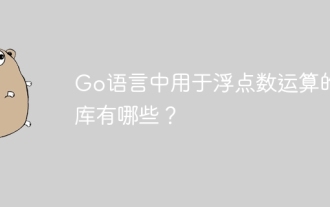
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
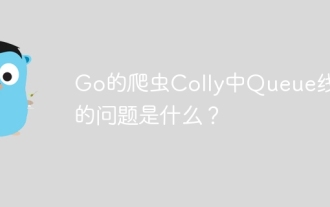
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
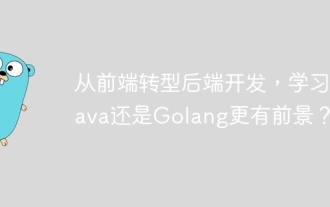
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
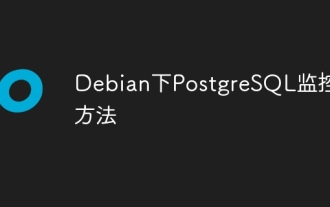
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
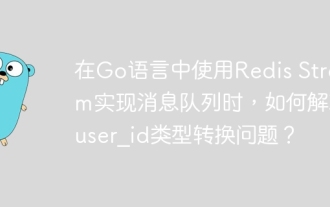
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
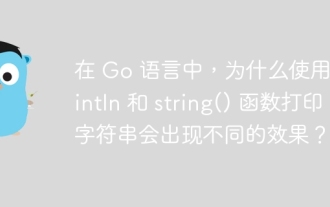
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
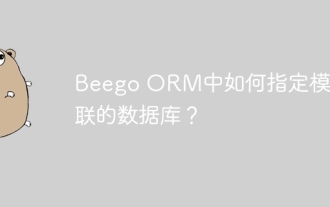
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
