Golang implements network disk
May 15, 2023 am 09:44 AMIn today's Internet environment, people increasingly need cloud storage services such as network disks. There are currently popular cloud storage services on the market such as Baidu Cloud, Weiyun, Dropbox, etc. However, the privacy and security of these services are not very trusted by people. Therefore, developing a network disk yourself has become an increasingly popular choice.
This article will introduce how to use Golang to develop a web-based network disk service. Golang is an open source programming language developed by Google. It has the characteristics of high concurrency, high performance, simplicity and ease of use. Using Golang to develop network disks can quickly build stable and efficient services.
1. Environment preparation
First, we need to install the Golang environment. After installing Golang, we need to install several necessary libraries:
- gin: a high-performance web framework for Golang, used to quickly build RESTful APIs.
- gorm: Golang's ORM library, used to conveniently operate databases.
After the installation is complete, we can start writing code happily.
2. Use the Gin framework to build a RESTful API
In this project, we use the Gin framework to build a Web service. The Gin framework is simple and easy to use, with almost no learning cost. First create a main.go file and write the following code:
package main import "github.com/gin-gonic/gin" func main() { // 创建Gin引擎 r := gin.Default() // 路由 r.GET("/ping", func(c *gin.Context) { c.JSON(200, gin.H{ "message": "pong", }) }) // 启动服务 r.Run() }
This code creates a Gin engine and handles a /ping route. Run the code: go run main.go, then enter http://localhost:8080/ping in the browser, you can see the output: {"message":"pong"}.
3. File Operation
Next we need to upload and download files. Uploading and downloading files requires first connecting to the database, accessing the meta-information of the corresponding file, and then accessing or downloading the file itself.
- File meta information
We will use MySQL database to store file meta information, including file name, file size, upload time, file storage path on disk, etc. In this project, we use the Gorm library to connect to the MySQL database. Add the following code to main.go:
import ( "fmt" "github.com/jinzhu/gorm" _ "github.com/jinzhu/gorm/dialects/mysql" ) type File struct { ID uint `gorm:"primary_key"` Name string `gorm:"type:varchar(255)"` Size int64 Path string `gorm:"type:varchar(255)"` CreatedAt time.Time } var db *gorm.DB var err error func init() { db, err = gorm.Open("mysql", "root:password@/filestore?charset=utf8&parseTime=True&loc=Local") if err != nil { fmt.Println("连接数据库失败:", err.Error()) return } db.AutoMigrate(&File{}) }
The above code creates a File structure, which corresponds to a table in the database. Then use gorm.Open to connect to the MySQL database, and use the AutoMigrate command to automatically create a table named "files" in the database.
- File upload
In the Gin framework, use the Post method to upload files. We need to add the following code before routing to enable the middleware for uploading files:
r.Static("/static", "./static") r.POST("/upload", func(c *gin.Context) { file, header, err := c.Request.FormFile("file") if err != nil { c.JSON(http.StatusBadRequest, gin.H{ "msg": err.Error(), }) return } defer file.Close() // 保存文件到本地 filename := header.Filename filepath := fmt.Sprintf("./static/%s", filename) err = c.SaveUploadedFile(header, filepath) if err != nil { c.JSON(http.StatusInternalServerError, gin.H{ "msg": err.Error(), }) return } // 将文件元信息存入数据库 size := header.Size f := File{ Name: filename, Size: size, Path: filepath, CreatedAt: time.Now(), } _,err = f.InsertFile() if err !=nil{ c.JSON(http.StatusInternalServerError, gin.H{ "msg": err.Error(), }) return } c.JSON(http.StatusOK, gin.H{ "msg": "Upload successful", }) })
In the above code, we successfully obtain the uploaded file from the Request Form, and then save the file to our server. . After that, we store the file metainformation in the database, where InsertFile() is the database operation function we create later.
- File download
We write a simple download function to output the file content into the HTTP response for client downloading. The complete code is as follows:
func (file File) GetFile() (err error) { //打开文件并读取文件内容 f, err := os.Open(file.Path) if err != nil { return } defer f.Close() w := bufio.NewWriter(file) b := make([]byte, 100) n := 0 for { n, err = f.Read(b) if err != nil && err != io.EOF{ return } w.Write(b[:n]) if n == 0{ break } } w.Flush() return } r.GET("/download", func(c *gin.Context) { fileName := c.Query("name") if len(fileName) == 0 { c.JSON(http.StatusBadRequest, gin.H{ "msg": "invalid parameter", }) return } file := File{ Name: fileName, } err = file.GetFile() if err !=nil{ c.JSON(http.StatusInternalServerError, gin.H{ "msg": err.Error(), }) return } c.Writer.Header().Add("Content-Disposition", fmt.Sprintf("attachment;filename=%s", fileName)) c.Writer.Header().Add("Content-Type", "application/octet-stream") c.File(file.Path) })
4. Storage Optimization
In a high-concurrency environment, file upload and download must be efficient. Therefore, we can use distributed storage to optimize this problem. CDN acceleration, object storage and other methods can be used.
5. Complete code
Next, we will host the complete code on Git.
Create the project directory, and then execute the following command in the terminal:
git init
echo "# fileServer" >> README.md
git add README.md
git commit -m "first commit"
git remote add origin git@github.com:username/fileServer.git
git push -u origin master
For the complete code, please refer to: https: //github.com/username/fileServer.
Note: Replace username with your GitHub username.
6. Summary
This article mainly introduces how to use Golang to develop a Web-based network disk service. Through this article, we can understand the basic usage of the Gin framework, Gorm library, and MySQL database, as well as how to implement operations such as file upload and download. The complete code provided in this article can help readers better understand the content described in this article and help in practical applications.
The above is the detailed content of Golang implements network disk. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
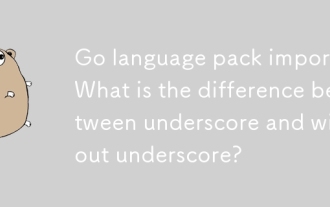
Go language pack import: What is the difference between underscore and without underscore?

How to implement short-term information transfer between pages in the Beego framework?
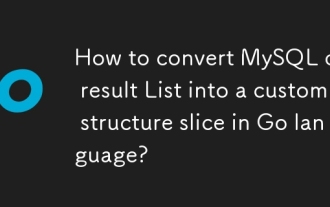
How to convert MySQL query result List into a custom structure slice in Go language?
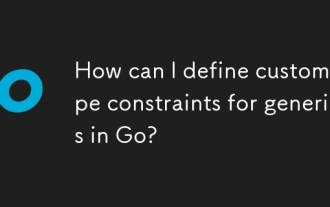
How can I define custom type constraints for generics in Go?
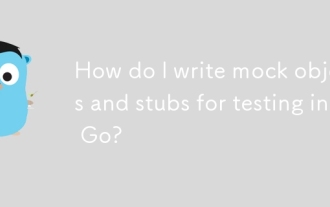
How do I write mock objects and stubs for testing in Go?
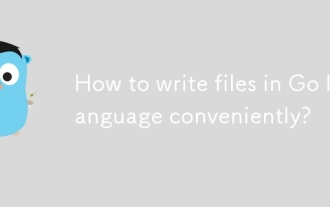
How to write files in Go language conveniently?
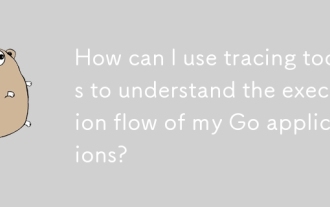
How can I use tracing tools to understand the execution flow of my Go applications?
