Golang implements factory mode
Go language is a very popular statically compiled language. Its unique syntax and features make it very suitable for application in many scenarios. Among them, the factory pattern is one of the very common design patterns in the Go language. This article will introduce how to implement the factory pattern in Go language.
- Introduction to Factory Pattern
Factory pattern is a common creation pattern. It defines an interface for creating objects and lets subclasses decide which objects to instantiate. a class. The factory pattern can separate the creation and use of objects, thereby improving the scalability and maintainability of the code.
Factory pattern often has two implementation methods: simple factory pattern and factory method pattern. The simple factory pattern generates all required objects from a factory class. It returns the corresponding objects by judging the type of the incoming parameters. The factory method pattern defines an interface for creating objects and lets subclasses decide which classes need to be instantiated.
- Simple Factory Pattern Implementation
Let’s first look at the implementation of a simple factory pattern. Suppose we have a shape interface (Shape), which has a method (Draw) for drawing shapes. We hope to generate different shape objects, such as Circle and Rectangle, based on the different parameters passed in.
First we define a shape interface:
type Shape interface { Draw() string }
Then we define two shape objects - circle and rectangle:
type Circle struct {} func (c *Circle) Draw() string { return "draw circle" } type Rectangle struct {} func (r *Rectangle) Draw() string { return "draw rectangle" }
Finally we define a factory class (ShapeFactory) , to generate different shape objects based on the different parameters passed in:
type ShapeFactory struct {} func (sf *ShapeFactory) CreateShape(shapeType string) Shape { switch shapeType { case "circle": return &Circle{} case "rectangle": return &Rectangle{} default: panic("wrong shape type") } }
One thing to note here is that the factory class needs to return a shape interface, not a specific shape object. This avoids unnecessary dependence on the user of the specific type of the return value.
The following is a code example using a simple factory pattern:
sf := &ShapeFactory{} circle := sf.CreateShape("circle") fmt.Println(circle.Draw()) // 输出:draw circle rectangle := sf.CreateShape("rectangle") fmt.Println(rectangle.Draw()) // 输出:draw rectangle
- Factory method pattern implementation
Next let’s look at a factory method pattern accomplish. Still taking shapes as an example, we change the original shape interface to a shape factory interface (ShapeFactoryInterface), which defines a method (CreateShape) for creating shape objects:
type ShapeFactoryInterface interface { CreateShape() Shape }
Then we define two Shape factory - Circle Factory (CircleFactory) and Rectangle Factory (RectangleFactory). They all implement the shape factory interface, which is used to create corresponding shape objects:
type CircleFactory struct {} func (cf *CircleFactory) CreateShape() Shape { return &Circle{} } type RectangleFactory struct {} func (rf *RectangleFactory) CreateShape() Shape { return &Rectangle{} }
As you can see, each shape has a corresponding factory, which is used to create instances of the shape. In this way, we can create different shape objects very flexibly without having to worry about coupling issues between different shape objects.
Finally, let’s look at a complete code example for creating factory objects of different shapes:
cf := &CircleFactory{} circle := cf.CreateShape() fmt.Println(circle.Draw()) // 输出:draw circle rf := &RectangleFactory{} rectangle := rf.CreateShape() fmt.Println(rectangle.Draw()) // 输出:draw rectangle
- Summary
That’s it, we Two ways to implement the factory pattern in Go language have been introduced: simple factory pattern and factory method pattern. In practical applications, we can choose the appropriate implementation method based on specific needs and scenarios.
The factory pattern can greatly improve the scalability and maintainability of the code, especially in scenarios where objects need to be created frequently. Therefore, it is very necessary for Go language developers to master the application method of factory pattern.
The above is the detailed content of Golang implements factory mode. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
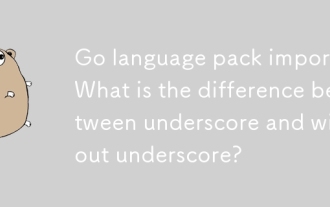
This article explains Go's package import mechanisms: named imports (e.g., import "fmt") and blank imports (e.g., import _ "fmt"). Named imports make package contents accessible, while blank imports only execute t

This article explains Beego's NewFlash() function for inter-page data transfer in web applications. It focuses on using NewFlash() to display temporary messages (success, error, warning) between controllers, leveraging the session mechanism. Limita
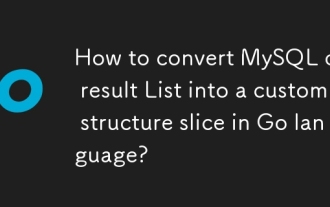
This article details efficient conversion of MySQL query results into Go struct slices. It emphasizes using database/sql's Scan method for optimal performance, avoiding manual parsing. Best practices for struct field mapping using db tags and robus
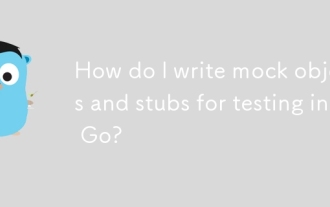
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
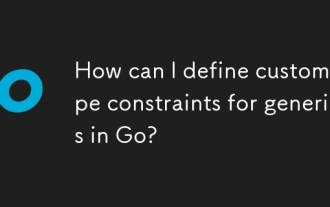
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
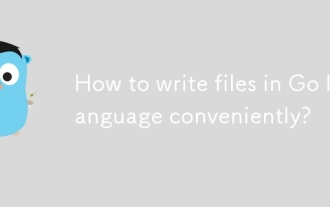
This article details efficient file writing in Go, comparing os.WriteFile (suitable for small files) with os.OpenFile and buffered writes (optimal for large files). It emphasizes robust error handling, using defer, and checking for specific errors.
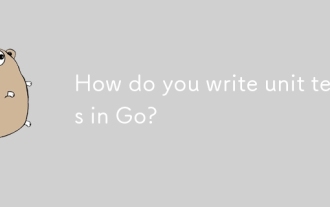
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
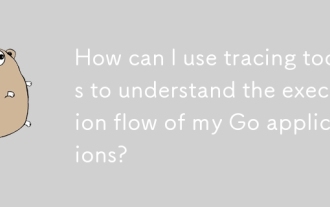
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
