golang json escape characters
In golang, json is a commonly used data exchange format. It can easily serialize structured data into json format, and can also deserialize back to the original data type from json format data. In the process of using json, sometimes you need to escape some special characters to avoid errors in the json string. So, how to escape json characters in golang? This article will introduce it to you in detail.
json in golang
In golang, you can use the encoding/json package to process json data. This package provides Marshal and Unmarshal functions, which can convert any data type in golang into a json format string, and convert a json format string back to the original data type in golang. The following is a simple example:
package main import ( "encoding/json" "fmt" ) type Person struct { Name string `json:"name"` Age int `json:"age"` } func main() { p := Person{ Name: "Tom", Age: 20, } data, _ := json.Marshal(p) fmt.Println(string(data)) var newPerson Person json.Unmarshal(data, &newPerson) fmt.Println(newPerson) }
The output result is:
{"name":"Tom","age":20} {Tom 20}
As you can see, by serializing the Person structure instance into a json format string, and then deserializing it and Restoring it to a new Person instance realizes the process of converting the golang data type into json format, and then converting it back to the golang data type in the opposite direction.
Escape characters in json strings
In json strings, some characters need to be escaped, otherwise syntax errors will occur. Common escape characters include:
Escape character | Meaning |
---|---|
\" | Double quotes |
\ | Backslash |
/ | Forward slash |
Backspace | |
Page change | |
Line feed | |
Enter | |
Horizontal tab character |
{"name": "Tom", "introduction": "I'm a "programmer""}
In the above json string, the double quotation mark does not use an escape character after the last word "programmer", causing the json encoder to not recognize it correctly. this string. This error can be handled as follows:
{"name":"Tom","introduction":"I'm a "programmer""}
In the above string, the programmer has used double quotes (") to escape the double quotes in the last word, thereby avoiding the json encoder An error occurred.
Json escape character processing
In golang, you can use json.Marshal for json escape character processing. This function will escape any required characters, including basic escape characters and Unicode code points.
For example, if you need to convert the following structure:
type Person struct { Name string `json:"name"` Gender string `json:"gender"` }
into the following json format string:
{"name":"Tom","gender":"He said, "I'm the best!""}
, you can use the following code:
p := Person{ Name: "Tom", Gender: `He said, "I'm the best!"`, } data, _ := json.Marshal(p) fmt.Println(string(data))
The output result is:
{"name":"Tom","gender":"He said, "I'm the best!""}
It can be seen that when using the json.Marshal function to serialize the p instance into a string in json format, the double quotes are automatically escaped to ", thereby avoiding json encoding Converter error.
In addition to common escape characters, the json.Marshal function provided by golang can also handle control characters and multi-byte characters. We can serialize structure data into characters in json format Before string, process the characters that need to be escaped:
p := Person{ Name: "Tom", Gender: `He said, "I'm the best!"`, } // 处理多字节字符 p.Gender = strings.Replace(p.Gender, "!", "\uff01", -1) // 处理控制字符 p.Gender = strings.Replace(p.Gender, "", "\u0001", -1) data, _ := json.Marshal(p) fmt.Println(string(data))
In the above code, first use the strings.Replace function in golang's standard library to replace the multi-byte characters in the string with the corresponding UTF-8 code point; then use the same method to replace the control characters in the string. Finally, use json.Marshal to serialize the p instance into a string in json format, and the output result is:
{"name":"Tom","gender":"He said, "I'm the best!\uff01""}
You can see that in the json string, we have escaped the characters that need to be escaped into the corresponding escape characters. This can avoid json encoder errors.
Summary
In golang, you can use the json.Marshal function to easily convert structure data into a json format string and automatically escape the characters that need to be escaped. This can avoid errors in the json string. The correctness of json data is guaranteed. When processing json data, other methods need to be used for conversion of multi-byte characters and control characters.
The above is the detailed content of golang json escape characters. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
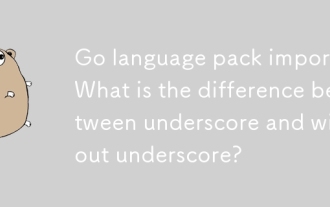
This article explains Go's package import mechanisms: named imports (e.g., import "fmt") and blank imports (e.g., import _ "fmt"). Named imports make package contents accessible, while blank imports only execute t
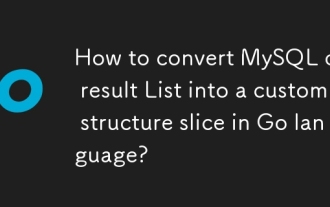
This article details efficient conversion of MySQL query results into Go struct slices. It emphasizes using database/sql's Scan method for optimal performance, avoiding manual parsing. Best practices for struct field mapping using db tags and robus

This article explains Beego's NewFlash() function for inter-page data transfer in web applications. It focuses on using NewFlash() to display temporary messages (success, error, warning) between controllers, leveraging the session mechanism. Limita
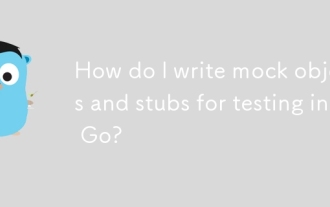
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
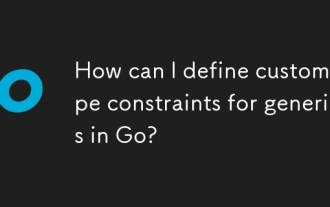
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
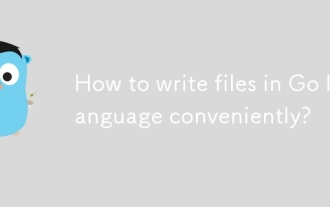
This article details efficient file writing in Go, comparing os.WriteFile (suitable for small files) with os.OpenFile and buffered writes (optimal for large files). It emphasizes robust error handling, using defer, and checking for specific errors.
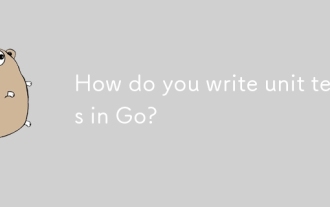
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
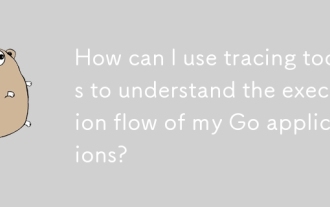
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
