golang divisor is 0
Golang is a relatively new programming language that is loved by more and more developers due to its efficiency, simplicity and powerful concurrency processing capabilities. However, just like other programming languages, Golang has its own bugs and shortcomings. Among them, a common problem is that the divisor is 0.
In mathematics, we all know that dividing by 0 is illegal. In programming, a divisor of 0 will cause the program to crash or experience an exception, affecting the stability and reliability of the program. Therefore, we need to find a solution to this problem.
There may be many reasons for the problem of divisor by 0 in Golang. Sometimes, unchecked variable values may appear as 0 in a program, resulting in a divide-by-zero situation. Sometimes, using illegal arithmetic operators in the program can also cause this problem.
In order to avoid the problem of Golang's divisor being 0, we can use the following methods:
- Check the variable value: Before performing the division operation, we should check whether the value of the variable is 0. If the variable value is 0, we need to handle it specially or throw an exception. For example, you can add an if statement to the code to determine whether the divisor is 0 and handle the situation:
if divisor == 0 { fmt.Println("Divisor cannot be zero.") return }
- Use the panic mechanism: When the program encounters a situation where the divisor is 0, we You can use the panic mechanism to cause the program to crash directly and output an error message. Although this does not completely solve the problem, it can detect errors in time when the program crashes, so as to better troubleshoot the problem.
if divisor == 0 { panic("Divisor cannot be zero.") }
- Use exception capture mechanism: In Golang, we can use an exception capture mechanism similar to try-catch, that is, defer-panic-recover mechanism. When an exception occurs in the program, the panic function will be called and propagated upward until the recover function is encountered. The recover function can capture the panic information at this time and perform specific processing. For example, you can add a recover() function to the function and handle the exception when the divisor is 0:
func divide(dividend, divisor int) int { defer func() { if r := recover(); r != nil { fmt.Println("Recovered in divide", r) } }() return dividend / divisor }
The above methods can solve the problem of divisor by 0 in Golang. However, in actual development, we need to choose the solution that suits us best, follow best practices, and ensure the maintainability and reliability of the program.
Finally, let’s summarize Golang’s solution to the problem of divisor by 0:
- Before performing the division operation, check whether the value of the variable is 0.
- Using the panic mechanism, when the program encounters a division by 0, it will directly crash and output an error message.
- Use the exception catching mechanism, add the recover() function to the function, and perform exception handling when the divisor is 0.
Through the above methods, we can better solve the problem of divisor by 0 in Golang and make our program more robust and stable.
The above is the detailed content of golang divisor is 0. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
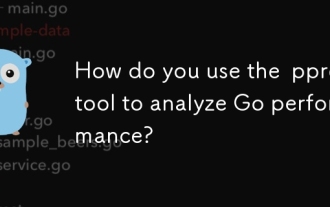
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
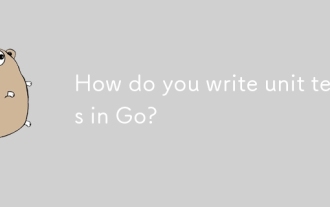
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
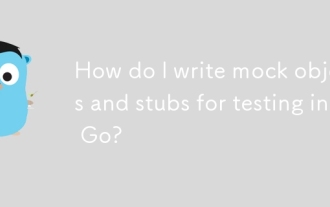
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
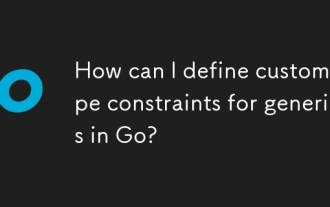
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
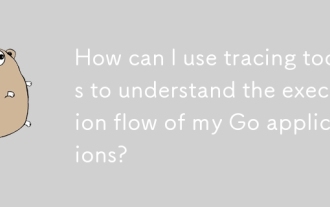
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
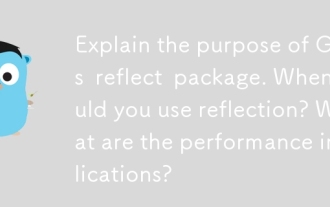
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
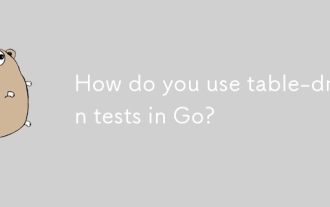
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
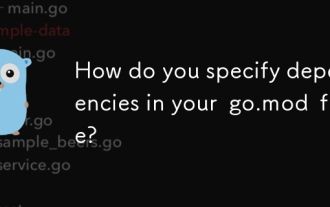
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.
