Redis Session Management in PHP Applications
Session management of Redis in PHP applications
With the rapid development of the Internet, Web applications have become more and more popular, and PHP, as one of the most commonly used languages in the field of Web development, is used in applications. Status is also becoming more and more important. In the development of web applications, Session is a very common function used to implement user state management.
For Session management in PHP applications, Redis is a very practical solution. Redis is a fast key-value storage technology, generally used for functions such as caching, queuing, message publishing and subscription. In PHP applications, Redis can handle Session-related issues very well.
This article will introduce how to configure, optimize and ensure data security when using Redis to store Sessions in PHP applications.
1. Basic ideas of Redis Session management
Before using Redis to store Session, you first need to determine the installation location and Session management method of Redis. Redis usually runs as a standalone server, and the interaction between PHP and Redis usually relies on libraries such as Predis. In PHP, there are two ways to use Redis to store Session:
- Redis native Session storage method: directly store Session data in Redis, and PHP communicates with Redis through the redis.so extension.
- Redis proxy Session storage method: Store the Session in the PHP local file system, use Redis to proxy this storage, and PHP communicates with Redis through the RedisSessionHandler class.
Generally, the Redis proxy Session storage method is more stable and secure, because even if there is a problem with the Redis server, the Session data is still saved in the local file system. Therefore, we will take the Redis proxy Session storage method as an example to introduce how to use Redis to store Sessions in PHP applications.
2. Redis proxy Session storage implementation
Redis proxy Session storage implementation requires the use of the PHP built-in function session_set_save_handler, which is used to set the Session storage method and parameters. The Redis proxy Session storage needs to manage the Session data, ID and expiration time, which mainly includes the following aspects:
- Set the Session saving path and Redis server address
Use the session_set_save_handler function to set the Session save path and Redis server address to ensure that PHP can read and write Session data normally. The specific code is as follows:
$redisHost = "127.0.0.1"; // Redis服务器地址 $redisPort = 6379; // Redis端口号 $sessionDir = "/path/to/session"; // Session保存路径 // 打开Session function sessionOpen($savePath, $sessionName) { global $redisHost, $redisPort, $sessionDir; $redis = new Redis(); $redis->connect($redisHost, $redisPort); return true; } // 关闭Session function sessionClose() { global $redis; return $redis->close(); } // 读取Session function sessionRead($sessionId) { global $redis, $sessionDir; return $redis->get($sessionDir . "/sess_" . $sessionId); } // 写入Session function sessionWrite($sessionId, $sessionData) { global $redis, $sessionDir; return $redis->set($sessionDir . "/sess_" . $sessionId, $sessionData); } // 销毁Session function sessionDestroy($sessionId) { global $redis, $sessionDir; return $redis->del($sessionDir . "/sess_" . $sessionId); } // 清除过期Session function sessionGc($maxLifetime) { global $redis, $sessionDir; return true; } // 设置Session存储方式 session_set_save_handler('sessionOpen', 'sessionClose', 'sessionRead', 'sessionWrite', 'sessionDestroy', 'sessionGc');
- Set the expiration time of the Redis storage session
In Redis, the expiration time of the storage session can be achieved by setting the expire command of Redis. In PHP, to set the expiration time of the Redis storage session, you need to use the PHP built-in function session_set_cookie_params to set the Session ID and expiration time. The specific code is as follows:
$sessionName = 'my_session_id'; // Session ID $expireTime = 86400; // Session过期时间 session_name($sessionName); session_set_cookie_params($expireTime);
- Ensuring Session data security
When using Redis to store Session, you need to consider data security issues. Redis acts as an in-memory cache and may leak sensitive data stored in it to the outside. Therefore, some measures need to be taken to ensure the security of Session data. Specific methods include:
- Encrypt the Session ID to ensure the uniqueness and security of the Session data;
- Encrypt the Session data stored in Redis to prevent data leakage Leak;
- Set the httponly and secure attributes of the Session Cookie to ensure that the Session data is only delivered under HTTPS and cannot be accessed through JS.
3. Optimization solution for Redis Session management
When using Redis to store Sessions in PHP applications, you need to consider optimizing Session management. If the amount of Session data is too large, or there are too many concurrent Session requests, it will have a certain impact on the performance of the Redis server. In order to optimize Session management, the following solutions can be adopted:
- Set the Session expiration time to prevent the session from wasting resources and reduce the burden on the Redis server.
- Use Session compression algorithm to reduce the memory space occupied by Session. Session compression algorithms mainly include LZF, gzip, lzma, etc. You can choose the appropriate compression algorithm according to the actual application scenario.
- Realize distributed storage of Session data, disperse Session data to multiple Redis servers, and improve the reliability and scalability of Session data.
- Set the maximum memory limit of Redis to prevent Redis from causing memory overflow due to large amounts of Session data.
4. Summary
Using Redis to store Sessions in PHP applications can greatly improve the performance and reliability of Web applications. When implementing Redis proxy session storage, you need to pay attention to setting the session storage path, expiration time and Redis server address. In order to ensure the security of Session data, you can take measures such as encryption processing and setting httponly and secure attributes. In the process of optimizing Redis Session management, you can set the Session expiration time, use Session compression algorithm, implement distributed storage and other solutions to improve the performance of the Redis server and reduce memory usage.
The above is the detailed content of Redis Session Management in PHP Applications. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


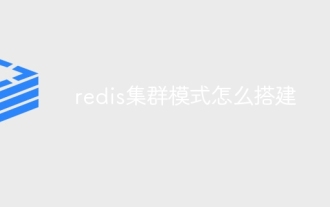
Redis cluster mode deploys Redis instances to multiple servers through sharding, improving scalability and availability. The construction steps are as follows: Create odd Redis instances with different ports; Create 3 sentinel instances, monitor Redis instances and failover; configure sentinel configuration files, add monitoring Redis instance information and failover settings; configure Redis instance configuration files, enable cluster mode and specify the cluster information file path; create nodes.conf file, containing information of each Redis instance; start the cluster, execute the create command to create a cluster and specify the number of replicas; log in to the cluster to execute the CLUSTER INFO command to verify the cluster status; make
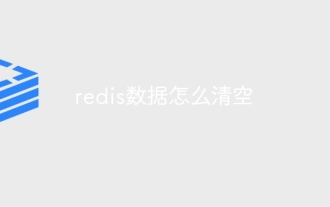
How to clear Redis data: Use the FLUSHALL command to clear all key values. Use the FLUSHDB command to clear the key value of the currently selected database. Use SELECT to switch databases, and then use FLUSHDB to clear multiple databases. Use the DEL command to delete a specific key. Use the redis-cli tool to clear the data.
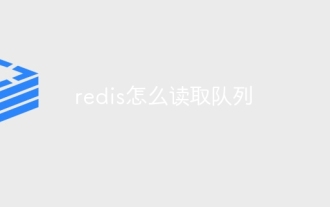
To read a queue from Redis, you need to get the queue name, read the elements using the LPOP command, and process the empty queue. The specific steps are as follows: Get the queue name: name it with the prefix of "queue:" such as "queue:my-queue". Use the LPOP command: Eject the element from the head of the queue and return its value, such as LPOP queue:my-queue. Processing empty queues: If the queue is empty, LPOP returns nil, and you can check whether the queue exists before reading the element.
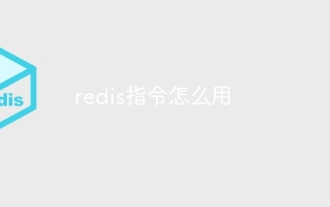
Using the Redis directive requires the following steps: Open the Redis client. Enter the command (verb key value). Provides the required parameters (varies from instruction to instruction). Press Enter to execute the command. Redis returns a response indicating the result of the operation (usually OK or -ERR).
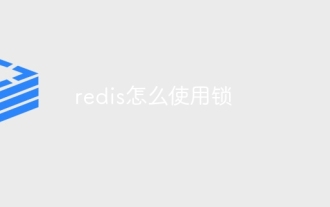
Using Redis to lock operations requires obtaining the lock through the SETNX command, and then using the EXPIRE command to set the expiration time. The specific steps are: (1) Use the SETNX command to try to set a key-value pair; (2) Use the EXPIRE command to set the expiration time for the lock; (3) Use the DEL command to delete the lock when the lock is no longer needed.
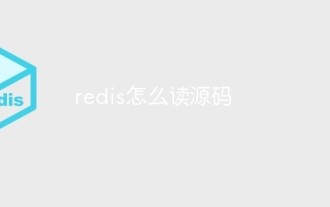
The best way to understand Redis source code is to go step by step: get familiar with the basics of Redis. Select a specific module or function as the starting point. Start with the entry point of the module or function and view the code line by line. View the code through the function call chain. Be familiar with the underlying data structures used by Redis. Identify the algorithm used by Redis.

Redis data loss causes include memory failures, power outages, human errors, and hardware failures. The solutions are: 1. Store data to disk with RDB or AOF persistence; 2. Copy to multiple servers for high availability; 3. HA with Redis Sentinel or Redis Cluster; 4. Create snapshots to back up data; 5. Implement best practices such as persistence, replication, snapshots, monitoring, and security measures.
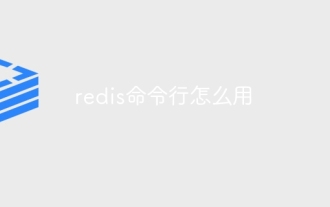
Use the Redis command line tool (redis-cli) to manage and operate Redis through the following steps: Connect to the server, specify the address and port. Send commands to the server using the command name and parameters. Use the HELP command to view help information for a specific command. Use the QUIT command to exit the command line tool.
