How to transfer copied files through JAVA NIO channel
通过JAVA NIO 通道传输拷贝文件
方式一
/** * 通过JAVA NIO 通道传输拷贝文件 * * @param sourcePath 源文件路径 * @param targetPath 目标文件路径 */ public static void copyFileByChannelTransfer(String sourcePath, String targetPath) { FileChannel inChannel = null; FileChannel outChannel = null; try { //获取通道 inChannel = FileChannel.open(Paths.get(sourcePath), StandardOpenOption.READ); outChannel = FileChannel.open(Paths.get(targetPath),StandardOpenOption.WRITE,StandardOpenOption.READ,StandardOpenOption.CREATE); inChannel.transferTo(0,inChannel.size(),outChannel); } catch (IOException e) { e.printStackTrace(); }finally { //关闭流 try { if (outChannel != null) { outChannel.close(); } if (inChannel != null) { inChannel.close(); } } catch (IOException e) { e.printStackTrace(); } } }
方式二
/** * 通过JAVA NIO 通道传输拷贝文件 * * @param sourcePath 源文件路径 * @param targetPath 目标文件路径 */ public static void copyFileByChannelTransfer2(String sourcePath, String targetPath) { FileInputStream fis = null; FileOutputStream fos = null; FileChannel inChannel = null; FileChannel outChannel = null; try { fis = new FileInputStream(sourcePath); fos = new FileOutputStream(targetPath); //获取通道 inChannel = fis.getChannel(); outChannel = fos.getChannel(); inChannel.transferTo(0,inChannel.size(),outChannel); } catch (IOException e) { e.printStackTrace(); }finally { //关闭流 try { if (outChannel != null) { outChannel.close(); } if (inChannel != null) { inChannel.close(); } } catch (IOException e) { e.printStackTrace(); } } }
使用示例
String source = "e:\\demo\\纵天神帝.txt"; String target = "e:\\demo\\"; long time1 = System.currentTimeMillis(); copyFileByStream(source, target + "1.txt"); System.out.println("通过字节流实现文件的拷贝耗时:" + (System.currentTimeMillis() - time1)); long time2 = System.currentTimeMillis(); copyFileByReaderAndWriter(source, target + "2.txt"); System.out.println("通过字符流实现文件的拷贝耗时:" + (System.currentTimeMillis() - time2)); long time3 = System.currentTimeMillis(); copyFileByBuffered(source, target + "3.txt"); System.out.println("通过字节缓冲流实现文件的拷贝耗时:" + (System.currentTimeMillis() - time3)); long time4 = System.currentTimeMillis(); copyFileByBufferedChar(source, target + "4.txt"); System.out.println("通过字符缓冲流实现文件的拷贝耗时:" + (System.currentTimeMillis() - time4)); long time5 = System.currentTimeMillis(); copyFileByChannel(source, target + "5.txt"); System.out.println("通过JAVA NIO通道(非直接缓冲区)实现文件的拷贝耗时:" + (System.currentTimeMillis() - time5)); long time6 = System.currentTimeMillis(); copyFileByChannelBufferd(source, target + "6.txt"); System.out.println("通过JAVA NIO通道(直接缓冲区)实现文件的拷贝耗时:" + (System.currentTimeMillis() - time6)); long time7 = System.currentTimeMillis(); copyFileByChannelTransfer(source, target + "7.txt"); System.out.println("通过JAVA NIO通道传输实现文件的拷贝耗时:" + (System.currentTimeMillis() - time7)); long time8 = System.currentTimeMillis(); copyFileByChannelTransfer(source, target + "8.txt"); System.out.println("通过JAVA NIO通道传输2实现文件的拷贝耗时:" + (System.currentTimeMillis() - time8));
The above is the detailed content of How to transfer copied files through JAVA NIO channel. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




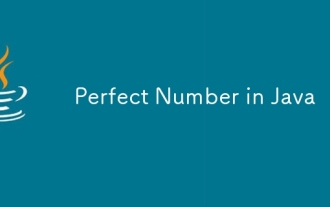
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
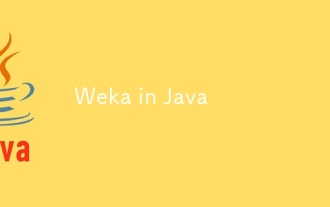
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
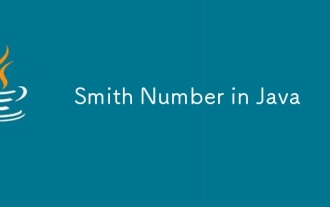
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
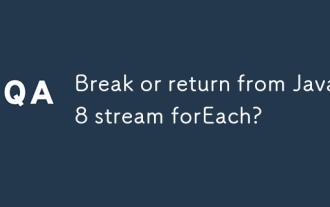
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
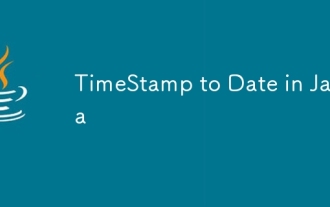
Guide to TimeStamp to Date in Java. Here we also discuss the introduction and how to convert timestamp to date in java along with examples.
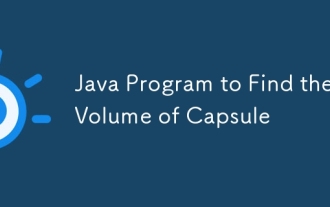
Capsules are three-dimensional geometric figures, composed of a cylinder and a hemisphere at both ends. The volume of the capsule can be calculated by adding the volume of the cylinder and the volume of the hemisphere at both ends. This tutorial will discuss how to calculate the volume of a given capsule in Java using different methods. Capsule volume formula The formula for capsule volume is as follows: Capsule volume = Cylindrical volume Volume Two hemisphere volume in, r: The radius of the hemisphere. h: The height of the cylinder (excluding the hemisphere). Example 1 enter Radius = 5 units Height = 10 units Output Volume = 1570.8 cubic units explain Calculate volume using formula: Volume = π × r2 × h (4
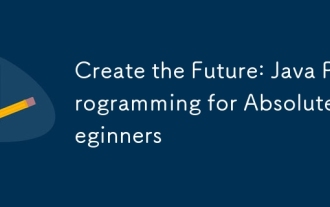
Java is a popular programming language that can be learned by both beginners and experienced developers. This tutorial starts with basic concepts and progresses through advanced topics. After installing the Java Development Kit, you can practice programming by creating a simple "Hello, World!" program. After you understand the code, use the command prompt to compile and run the program, and "Hello, World!" will be output on the console. Learning Java starts your programming journey, and as your mastery deepens, you can create more complex applications.
