


How to use PHP to connect to the database using the ORM framework
PHP uses the ORM framework to connect to the database
The ORM (Object-Relational Mapping) framework is a technology that maps the object model and the relational database model. It allows developers to use objects to operate the database, thereby avoiding the tedious and error-prone problems of hand-written SQL statements. ORM frameworks are widely used in PHP, such as Laravel's Eloquent ORM, Symfony's Doctrine ORM, etc.
In this article, we will introduce how to use Doctrine ORM to connect to the database and how to perform CRUD operations on the database. This article assumes that you are already familiar with basic PHP syntax and database operations. If you are not familiar with Doctrine ORM, you can refer to its official documentation to learn.
Step 1: Install Doctrine ORM
You can install Doctrine ORM in Composer and execute the following command:
composer require doctrine/orm
Step 2: Configure database connection
Doctrine ORM supports a variety of databases, such as MySQL, PostgreSQL, SQLite, etc. Here, we take connecting to the MySQL database as an example to illustrate.
Open the configuration file config.php and add the following content:
use DoctrineORMToolsSetup; use DoctrineORMEntityManager; require_once 'vendor/autoload.php'; $paths = array(__DIR__ . '/src'); $isDevMode = true; $dbParams = array( 'driver' => 'pdo_mysql', 'user' => 'your_database_user', 'password' => 'your_database_password', 'dbname' => 'your_database_name', ); $config = Setup::createAnnotationMetadataConfiguration($paths, $isDevMode); $entityManager = EntityManager::create($dbParams, $config);
Here, we use the Setup and EntityManager classes provided by Doctrine to configure the database connection. Among them, the $paths parameter specifies the directory where we store the entity class (Entity Class), and the $isDevMode parameter indicates whether to enable developer mode.
Step 3: Define entity classes
We need to define entity classes to map the table structure in the database. For example, define a User class to map the users table:
<?php namespace MyAppEntity; /** * @Entity @Table(name="users") **/ class User { /** * @Id @Column(type="integer") * @GeneratedValue **/ protected $id; /** * @Column(type="string") **/ protected $name; /** * @Column(type="string") **/ protected $email; // 省略 getter 和 setter 方法 }
Here, we use the annotations provided by Doctrine to define the entity class. The @Entity annotation indicates that this is an entity class, and the @Table annotation indicates that it is mapped to the table name in the database. The @Id annotation indicates that this is the primary key, and the @Column annotation indicates that this is a column in the database. In addition, we can also use other annotations to define relationships, indexes, etc.
Step 4: Perform CRUD operations
We can use EntityManager to perform CRUD operations on the database. For example, insert a piece of data:
<?php use MyAppEntityUser; $user = new User(); $user->setName('Alice'); $user->setEmail('alice@example.com'); $entityManager->persist($user); $entityManager->flush();
Here, we create a User object through the new operator and set its attribute values. Then, we use $entityManager->persist($user) to add it to the dirty unit of EntityManager, and finally use $entityManager->flush() to write it to the database.
In addition, we can also use the $entityManager->find(User::class, $id) method to find data, use the $entityManager->remove($user) method to delete data, and use The $entityManager->createQuery() method performs complex query operations and so on.
Conclusion
This article introduces the basic method of using the Doctrine ORM framework to connect to the MySQL database and perform CRUD operations. Of course, this is just an introduction, and there are many advanced uses that can be used. We recommend that you study the relevant documentation in depth and practice with actual projects.
The above is the detailed content of How to use PHP to connect to the database using the ORM framework. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


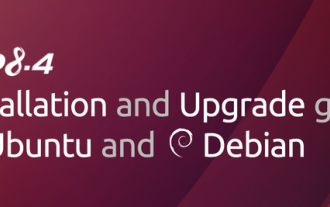
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
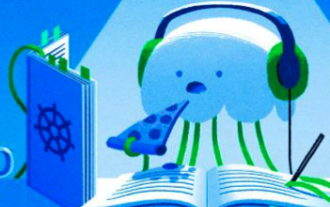
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
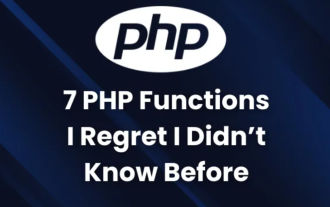
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
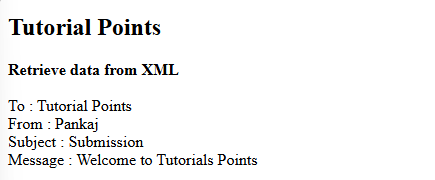
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
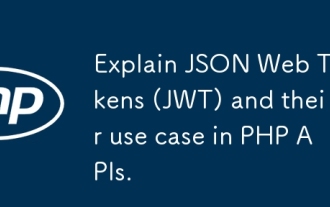
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
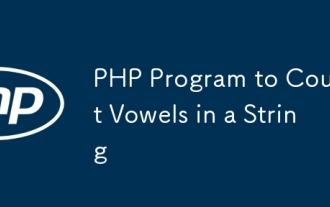
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
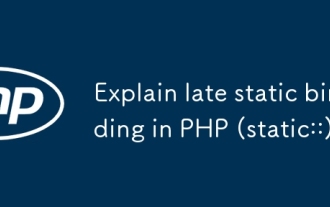
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
