How to use the new, make and append keywords of Golang functions
Golang is a programming language with high development efficiency, fast running speed, and high concurrent processing capabilities. It is widely used in network programming, server development, cloud computing and other fields. In the Golang programming process, new, make, and append are keywords we often use, and learning to use them correctly will make us more comfortable in writing efficient and robust code.
This article will introduce in detail the use of new, make and append in Golang to help readers better master these keywords.
1. New keyword
new is a built-in function, its function is to allocate a memory space on the heap and return the address of the space. That is, new returns a pointer of type pointing to the zero value of a value.
Generally, we use the new function to allocate the memory space of a structure, as follows:
package main import "fmt" type Person struct { Name string Age int } func main() { p := new(Person) fmt.Println(p.Name, p.Age) }
The output result is:
"" 0
As you can see from the output result , the fields in the structure memory space allocated using the new function are set to zero values. It should be noted that the new function only allocates memory space and does not automatically initialize the allocated memory space.
2. Make keyword
make is also a built-in function, but its function is slightly different from that of the new function. The function of make is to create a slice, map or channel and return a value of that type.
Slicing refers to a variable-length sequence of elements of the same type. Using slicing can manage memory space more efficiently. The following code shows the use of the make function to create a string slice containing 3 elements:
slice := make([]string, 3)
When creating a slice, you can specify its capacity through the second parameter. For example:
slice := make([]string, 3, 5)
The string slice created here has a length of 3 and a capacity of 5. It should be noted that when using make to create a slice, the system will initialize the newly allocated memory space and initialize all elements in it to zero values.
In addition to slicing, the make function can also create maps and channels. The following introduces how to use them:
- Create mapping
Mapping refers to a set of key-value structures, in which each element has a unique key and a corresponding value. You can easily create mappings using the make function, as follows:
m := make(map[string]string)
The mapping created using the make function has been initialized and can be operated directly.
- Create channel
Channel is a concurrent data exchange method, which is very important in Golang. Using the make function to create a channel can be achieved by specifying the type of the channel element, as follows:
ch := make(chan int)
When creating a channel, you can also set the buffer size for it by specifying the second parameter:
ch := make(chan int, 10)
3. append keyword
append is also a built-in function, its function is to append one or more values to the end of the slice and return the updated slice.
The syntax of the append function is as follows:
append(slice []type, elems ...type) []type
Among them, slice represents the slice to be appended, and elems represents the value to be appended to the slice. It should be noted that elems is a variable parameter and any number of values can be passed in.
The following is an example of using the append function to dynamically add elements to a slice:
package main import "fmt" func main() { var slice []int for i := 0; i < 10; i++ { slice = append(slice, i) fmt.Println(slice) } }
The output result is:
[0] [0 1] [0 1 2] ...
As can be seen from the output result, each time append is called The function appends a new element to the end of the slice.
It should be noted that if the number of elements to be appended is more than the remaining space of the slice, the append function will reallocate the memory space and copy the original data to the new memory space. Therefore, when using the append function, you should try to avoid insufficient slice capacity to avoid performance losses.
Summary
This article introduces in detail the use of new, make and append keywords in Golang. By learning the use of these keywords, we can better master the basic syntax of Golang and Write efficient, robust code. In actual projects, we should use these keywords appropriately according to specific needs to make the code more elegant and efficient.
The above is the detailed content of How to use the new, make and append keywords of Golang functions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


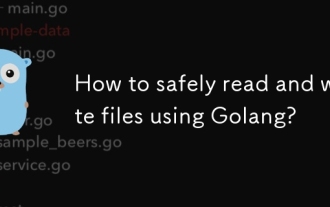
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
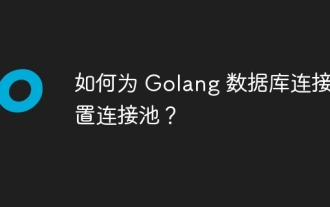
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
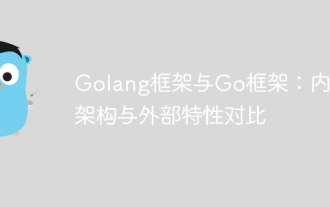
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
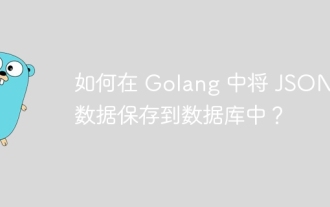
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.
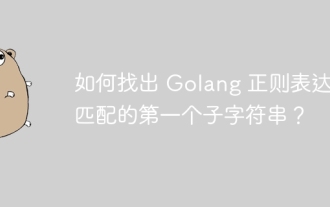
The FindStringSubmatch function finds the first substring matched by a regular expression: the function returns a slice containing the matching substring, with the first element being the entire matched string and subsequent elements being individual substrings. Code example: regexp.FindStringSubmatch(text,pattern) returns a slice of matching substrings. Practical case: It can be used to match the domain name in the email address, for example: email:="user@example.com", pattern:=@([^\s]+)$ to get the domain name match[1].
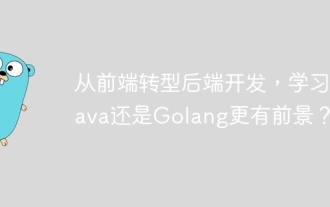
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
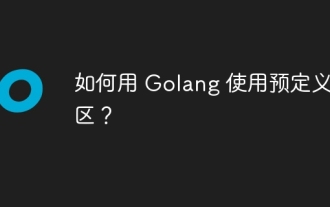
Using predefined time zones in Go includes the following steps: Import the "time" package. Load a specific time zone through the LoadLocation function. Use the loaded time zone in operations such as creating Time objects, parsing time strings, and performing date and time conversions. Compare dates using different time zones to illustrate the application of the predefined time zone feature.
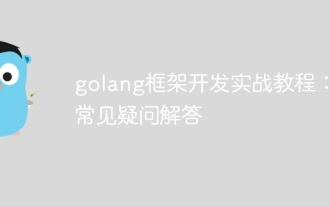
Go framework development FAQ: Framework selection: Depends on application requirements and developer preferences, such as Gin (API), Echo (extensible), Beego (ORM), Iris (performance). Installation and use: Use the gomod command to install, import the framework and use it. Database interaction: Use ORM libraries, such as gorm, to establish database connections and operations. Authentication and authorization: Use session management and authentication middleware such as gin-contrib/sessions. Practical case: Use the Gin framework to build a simple blog API that provides POST, GET and other functions.
