


Automatic generation method of function documentation and test coverage for Golang functions
Golang is an efficient and reliable programming language. During the development process, the automatic generation of function documentation and test coverage is very necessary. However, manually writing these documentation and test codes is tedious and time-consuming. This article will introduce how to use tools such as godoc, go test and go cover to automatically generate function documentation and test coverage.
1. Use godoc to generate function documentation
Godoc is Golang’s own document generation tool, which can automatically generate HTML documents for Go programs. Enter "godoc -http=:6060" on the command line to start godoc, and then visit "http://localhost:6060/pkg/" to see the documentation for all Go packages in the current working directory.
To generate function documentation, just add a comment before each function declaration. The format of the comment is:
// 函数名 // // 函数作用或功能说明 // // 参数1: 参数1说明 // 参数2: 参数2说明 // ... // // 返回值1: 返回值1说明 // 返回值2: 返回值2说明 // ... func functionName(param1, param2, ...) (return1, return2, ...) { ... }
For example:
// Add adds two integers and returns the result. // // x: the first integer. // y: the second integer. // // the sum of x and y. func Add(x, y int) int { return x + y }
Then use the command " godoc -http=:6060" starts godoc, and you can see the documentation for each function on the page of the package where the comment is located.
2. Use go test to generate test code
Testing is an important means to ensure code quality. Using the go test command, you can automatically generate test code and run tests. The file name of the test code must end with "_test.go".
For example, there is an add.go file:
package math func Add(x, y int) int { return x + y }
Next, we create a new add_test.go file in the same directory and write the test code:
package math import "testing" func TestAdd(t *testing.T) { if Add(2, 3) != 5 { t.Error("Add(2, 3) should be 5") } }
In Run "go test" on the command line to run the test. If the test passes, "PASS" is output; if the test fails, an error message is output.
3. Use go cover to generate test coverage
Test coverage is an important indicator to measure the quality of test cases. It can tell you which parts of your code are not being tested. Golang has a built-in test coverage tool go cover, which can be used to generate test coverage reports.
When using the go test command, add the -cover option to enable the test coverage function. For example:
go test -cover
It will generate a coverage report similar to the following:
PASS coverage: 100.0% of statements ok example.com/math 0.001s
Where, "coverage: 100.0%" means that the test coverage is 100%.
In addition to using the command line, you can also use go tool cover to view the test coverage report. Run the command "go tool cover -html=c.out" to generate a coverage report in HTML format.
4. Use go generate to automatically generate function documentation and test code
It is tedious to manually write comments and test code for each function. Use go generate to automatically generate function documentation and test code.
First, add the "//go:generate" comment in the code file:
//go:generate go doc -all //go:generate go test -cover -run=^$ github.com/yourname/yourrepo/yourpackage
Among them, "go doc -all" is used to generate function documentation, "go test -cover -run =^$" is used to generate test code and test coverage reports.
Then, run "go generate" in the command line to automatically generate function documentation and test code.
5. Conclusion
Automatically generating function documents and test codes can greatly improve development efficiency and reduce error rates. This article introduces how to automatically generate function documentation and test coverage using tools such as godoc, go test, and go cover. I hope it will be helpful to readers, promote automated development, and improve collaborative development efficiency and code quality.
The above is the detailed content of Automatic generation method of function documentation and test coverage for Golang functions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


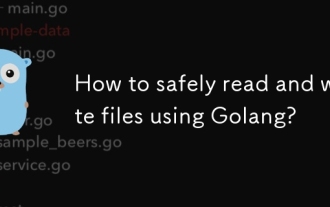
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
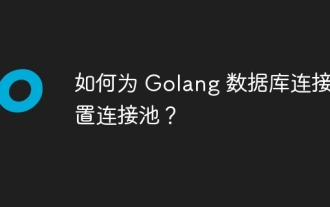
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
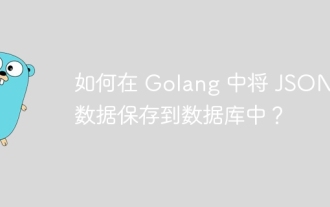
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.
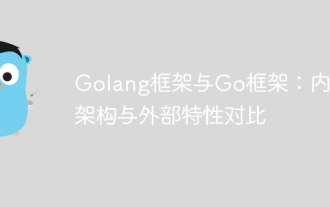
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
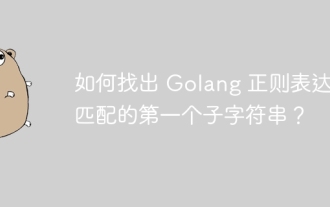
The FindStringSubmatch function finds the first substring matched by a regular expression: the function returns a slice containing the matching substring, with the first element being the entire matched string and subsequent elements being individual substrings. Code example: regexp.FindStringSubmatch(text,pattern) returns a slice of matching substrings. Practical case: It can be used to match the domain name in the email address, for example: email:="user@example.com", pattern:=@([^\s]+)$ to get the domain name match[1].
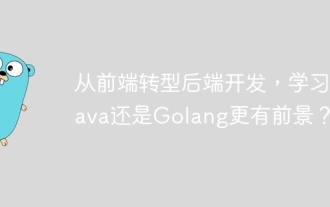
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
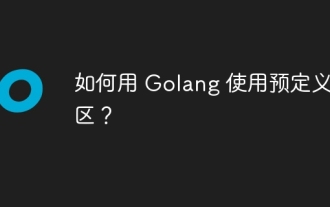
Using predefined time zones in Go includes the following steps: Import the "time" package. Load a specific time zone through the LoadLocation function. Use the loaded time zone in operations such as creating Time objects, parsing time strings, and performing date and time conversions. Compare dates using different time zones to illustrate the application of the predefined time zone feature.
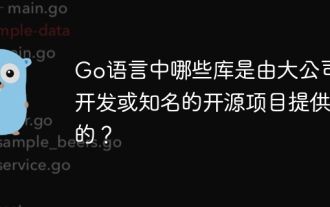
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
