Does javascript have map?
There is map() function in JavaScript, which is a higher-order function for arrays. The map() function accepts a function as a parameter, uses this function to operate on each element in the array, and finally returns a new array containing the result of executing the function on each element of the original array.
Using the map() function can conveniently perform operations such as conversion and calculation on the elements in the array.
The following is an example of using the map() function:
const numbers = [1, 2, 3, 4, 5]; const doubledNumbers = numbers.map(function(num) { return num * 2; }); console.log(doubledNumbers); // [2, 4, 6, 8, 10]
In the above code, we define a digital array numbers, and then use the map() function to perform Multiply by 2 and save the result in a new array doubleNumbers.
In addition, we can also pass in a context object this in the second parameter of the map() function to use the this keyword in the callback function.
Next let’s look at another example:
const names = ['Alice', 'Bob', 'Charlie']; const initials = names.map(function(name) { return name.charAt(0); }, this); console.log(initials); // ['A', 'B', 'C']
In this example, we define a name array names, and then use the map() function to extract the first letter of each name, And save the results in a new array initials. We also pass in a context object this through the second parameter for use in the callback function.
In general, the map() function in JavaScript is a very useful high-order function that can operate on each element in the array by passing in a callback function and return a new Arrays make processing array data easier.
The above is the detailed content of Does javascript have map?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


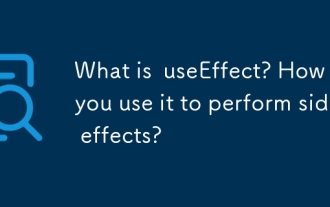
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.
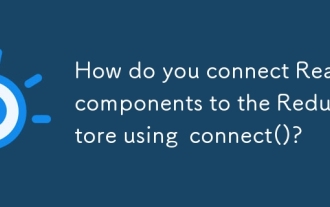
Article discusses connecting React components to Redux store using connect(), explaining mapStateToProps, mapDispatchToProps, and performance impacts.
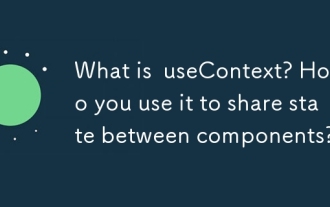
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
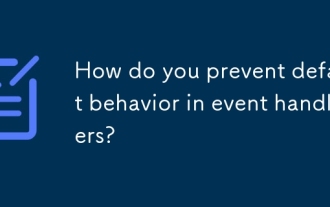
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.
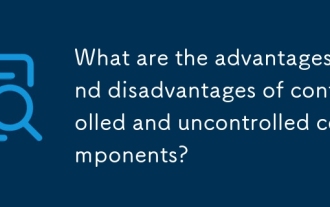
The article discusses the advantages and disadvantages of controlled and uncontrolled components in React, focusing on aspects like predictability, performance, and use cases. It advises on factors to consider when choosing between them.

React combines JSX and HTML to improve user experience. 1) JSX embeds HTML to make development more intuitive. 2) The virtual DOM mechanism optimizes performance and reduces DOM operations. 3) Component-based management UI to improve maintainability. 4) State management and event processing enhance interactivity.
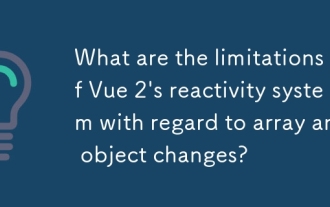
Vue 2's reactivity system struggles with direct array index setting, length modification, and object property addition/deletion. Developers can use Vue's mutation methods and Vue.set() to ensure reactivity.
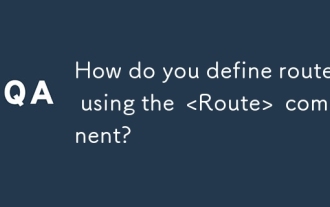
The article discusses defining routes in React Router using the <Route> component, covering props like path, component, render, children, exact, and nested routing.
