How to write javascript methods
JavaScript is a very popular programming language that is used to develop web applications, games, desktop applications, and server-side. In JavaScript, a method is a self-contained block of code that performs a specific task. Methods can make code more modular and facilitate writing more readable and maintainable code.
This article will discuss different ways of writing JavaScript methods, including function declarations, function expressions, arrow functions and immediate execution function expressions.
- Function declaration
Function declaration is one of the most common ways to define JavaScript methods. A function declaration begins with the "function" keyword, followed by the method name, then the parameter list enclosed in parentheses, and finally the method body enclosed in curly braces. For example:
function greet(name) { console.log("Hello, " + name + "!"); }
In this example, the greet method accepts a "name" parameter and outputs Hello, {name}! to the console. This method can be run by calling greet("World"), which outputs "Hello, World!".
The advantage of function declaration is that the method can be defined anywhere in the code and the method will have global scope. The disadvantage is that when there are many function declarations, it can become difficult to read and maintain.
- Function expressions
Function expressions are another way to define JavaScript methods. A function expression starts with the "var" or "let" keyword, followed by the assignment operator and a function. In this case, the function is an anonymous function. For example:
var greet = function(name) { console.log("Hello, " + name + "!"); };
Note that there are no parentheses added after the method name. In this case, JavaScript handles the function as a value and assigns it to the variable greet. Methods defined this way exist only after they are defined, and can only be called after they are defined. This method cannot be accessed outside the global function.
The advantage of function expressions is greater flexibility when defining methods. Because a function is a value, it can be used like any other variable. The disadvantage is that function expressions in code can be difficult to read, and these methods can be more difficult to maintain since the scope of the variables may be smaller.
- Arrow function
The arrow function is a new type of function expression introduced in ES6. Arrow functions are more concise and, in some cases, easier to relate to than traditional function expressions. For example:
let greet = (name) => console.log(`Hello, ${name}!`);
Arrow functions omit the "function" keyword and curly braces in the function declaration. In contrast, arrow functions use the "=>" notation to indicate the beginning of the function body and ignore parentheses, curly braces, and the "return" keyword. The argument list of an arrow function is defined in parentheses, but if there is only one argument, the parentheses can be omitted.
The main advantage of arrow functions is that their syntax is more concise than traditional function expressions. Arrow functions are also easier to use because they omit some extra symbols and keywords. They make code more readable and maintainable. The disadvantage is that arrow functions can be inflexible and cannot be used with callback functions that require the "this" keyword.
- Immediately execute function expression
Immediately execute function expression (IIFE) is a special type of function expression that can execute the function immediately at the same time of declaration. For example:
(function(number) { console.log(`The number is ${number}`); })(42);
In this example, an anonymous function is defined and a parameter 42 is passed immediately within the parentheses of the function definition. The method will be executed immediately and the results of the method will be output to the console. IIFE is often used to create a new scope between code segments, mainly to avoid creating global variables. Because variables defined in an IIFE will only exist within the scope, there are fewer conflicts and naming issues between code blocks.
The advantages of IIFEs are that they can execute functions at the same time they are declared, and they can create private scopes for the code. The disadvantage is that IIFE's syntax is more complex than other methods and can be more difficult to read and write.
Summary
JavaScript methods are the key to writing modular, readable, and maintainable code. This article introduces four common JavaScript method writing methods, including function declaration, function expression, arrow function and IIFE. Which method you choose depends on the specific needs and style of your code. No matter which way you choose, functions are a core part of JavaScript programming and provide logic and organization to your code.
The above is the detailed content of How to write javascript methods. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


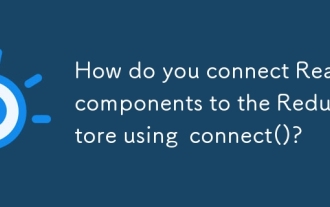
Article discusses connecting React components to Redux store using connect(), explaining mapStateToProps, mapDispatchToProps, and performance impacts.

React combines JSX and HTML to improve user experience. 1) JSX embeds HTML to make development more intuitive. 2) The virtual DOM mechanism optimizes performance and reduces DOM operations. 3) Component-based management UI to improve maintainability. 4) State management and event processing enhance interactivity.
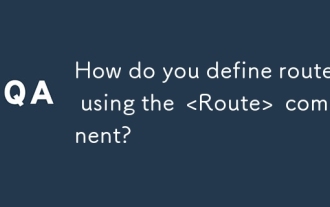
The article discusses defining routes in React Router using the <Route> component, covering props like path, component, render, children, exact, and nested routing.
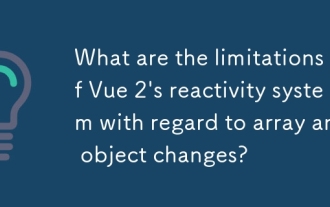
Vue 2's reactivity system struggles with direct array index setting, length modification, and object property addition/deletion. Developers can use Vue's mutation methods and Vue.set() to ensure reactivity.
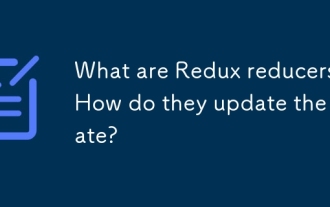
Redux reducers are pure functions that update the application's state based on actions, ensuring predictability and immutability.
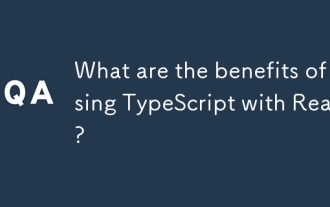
TypeScript enhances React development by providing type safety, improving code quality, and offering better IDE support, thus reducing errors and improving maintainability.
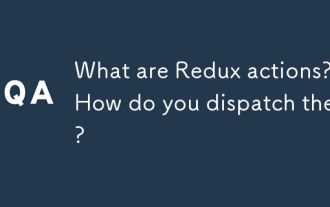
The article discusses Redux actions, their structure, and dispatching methods, including asynchronous actions using Redux Thunk. It emphasizes best practices for managing action types to maintain scalable and maintainable applications.
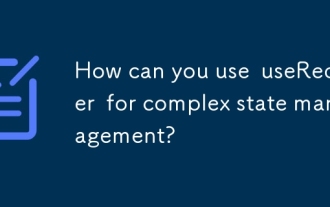
The article explains using useReducer for complex state management in React, detailing its benefits over useState and how to integrate it with useEffect for side effects.
