golang scheduled stop
More and more software systems require periodic operations, such as data backup, log cleaning, scheduled tasks, etc. When implementing these periodic operations, we usually need to use timers. Golang provides a built-in timer, but when performing timing operations, how can we stop the timer at the required time?
Generally speaking, we can use the time package provided by golang to generate a timer. For example:
timer1 := time.NewTimer(time.Second * 5) <-timer1.C fmt.Println("Timer 1 expired")
The above code will output "Timer 1 expired" after 5 seconds. The program here will receive a time signal from the timer channel (timer1.C) after waiting for 5 seconds, thereby realizing the timer's task. It should be noted that the timer created using the time.NewTimer() function will automatically repeat by default. You need to use timer.Stop() to end the timer, otherwise the timer will keep running.
But what if we need to stop the timer within a specified time? For example, when we are backing up data, if the specified backup time is exceeded, we need to forcefully stop the timer and end the backup task. At this time, we need to bind the stop signal when the timer is generated, and send abort information to the signal when it needs to be stopped.
// 定时任务函数 func doDataBackup(stopSignal chan bool){ // 模拟数据备份,并每10秒执行一遍 for { select { case <-time.After(time.Second * 10): backupData() case stop := <-stopSignal: if stop { fmt.Println("Data backup stopped.") return } } } } func main() { stopSignal := make(chan bool) // 每10秒备份一次数据,规定备份时间为50秒 go doDataBackup(stopSignal) time.Sleep(time.Second * 50) // 操纵停止信号,结束任务 stopSignal <- true }
In the above code, we added a stop signal stopSignal in the doDataBackup() function to accept forced stop information. When we need to stop the backup task, we only need to send true to the stopSignal channel, and the backup task will be forcibly stopped.
It should be noted that before sending a stop signal to the stopSignal channel, some necessary operations such as data saving and resource release may be required. These operations need to be performed before sending a stop signal to the channel, otherwise problems such as data loss may occur.
It should be emphasized that the above backup tasks are performed within a single coroutine. If we need to execute tasks regularly in multiple coroutines and need to stop them regularly, we can use golang's sync.WaitGroup and context packages to achieve this. For example:
func doTask(ctx context.Context, wg *sync.WaitGroup, id int){ defer wg.Done() fmt.Printf("goroutine %d started. ", id) for { select { case <-time.After(time.Second * 1): fmt.Printf("goroutine %d is working. ", id) case <-ctx.Done(): fmt.Printf("goroutine %d is stopped. ", id) return } } } func main() { wg := sync.WaitGroup{} ctx, cancel := context.WithTimeout(context.Background(), time.Second * 5) defer cancel() for i := 0; i < 3; i++ { wg.Add(1) go doTask(ctx, &wg, i) } wg.Wait() fmt.Println("Task completed.") }
In the above code, we created three coroutines. Each coroutine will perform a task regularly and control the closing of the coroutine through the incoming context. Use sync.WaitGroup to ensure that all coroutine tasks are completed before ending the program. Use the WithTimeout() function in the context, which stipulates that the maximum time for the task to run is 5 seconds. If the task is not completed within 5 seconds, the task will be forcibly stopped and the coroutine will exit.
The above is how to use timers in golang and how to implement scheduled stops. Using these methods, we can easily implement various periodic tasks and accurately control the running time and stop time of the tasks when needed.
The above is the detailed content of golang scheduled stop. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
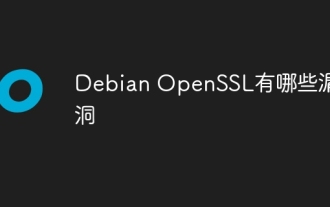
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
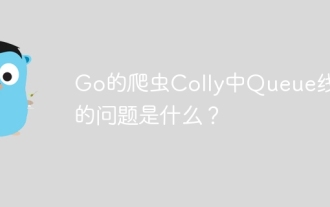
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
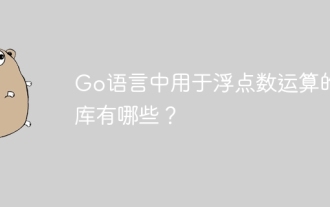
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
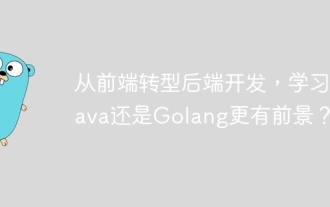
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
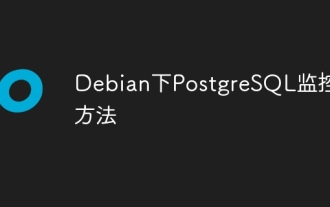
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
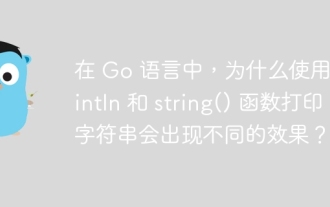
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
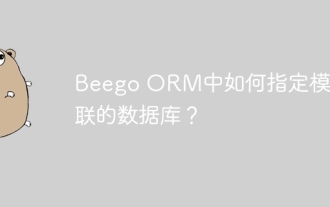
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
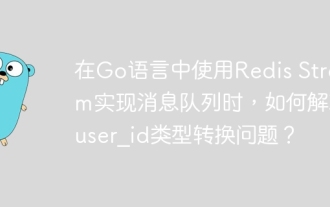
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
