How vue3 hook reconstructs DataV's full-screen container component
Implementation
Create component
fullScreenContainer.vue
<template> <div id="dv-full-screen-container" :ref="autoBindRef"> <slot></slot> </div> </template> <script setup lang="ts"> import { useAutoResize } from '@/hooks/useAutoResize' const { autoBindRef } = useAutoResize() </script>
Customize a hook and export an autoBindRef binding ref
Custom hook file
useAutoResize.ts
import { ref } from 'vue'; export function useAutoResize() { let domRef = ref<HTMLElement | null>(); function autoBindRef() { } return { autoBindRef } }
1. Bind domRef
Make sure to get the dom element first before the logic can continue.
Create a function to detect and obtain the correct dom element
function getRefDom(ref: HTMLElement | ComponentPublicInstance): HTMLElement | null { // ref指向dom,则返回ref // isElement检查指定的值是否为DOM元素 if (isElement(ref)) { return ref as HTMLElement } // 若ref指向组件实例,通过$el获取dom元素 if (isElement((ref as ComponentPublicInstance).$el)) { return (ref as ComponentPublicInstance).$el } return null }
Automatically bind the component domRef
export function useAutoResize() { let domRef = ref<HTMLElement | null>(); const autoBindRef = once((ref: HTMLElement | ComponentPublicInstance) => { const dom = getRefDom(ref); if(!dom) { console.warn("绑定组件domRef失败!") return; } domRef.value = dom; }) return { autoBindRef } }
2. Initialization
export function useAutoResize() { onMounted(() => { initDom(domRef.value) initConifg(domRef.value) }) }
mounted period, The DOM has been mounted, so the DOM elements need to be obtained during this cycle.
2.1. Initialize dom
function initDom(dom:HTMLElement) { const { clientWidth = 0, clientHeight = 0 } = dom || {} if(!dom) { console.warn("获取dom节点失败,组件渲染可能异常!") return } else if(!clientWidth || !clientHeight) { console.warn("组件宽度或高度为0px,可能会出现渲染异常!") return } // 设置缩放比例 if(typeof setAppSacle === 'function') setAppScale(dom) }
2.2. Initialize device
After obtaining the dom, set the obtained device screen resolution width and height to the dom.
function initConfig(dom:HTMLElement) { const { width, height } = screen || {} dom.style.width = `${width}px`; dom.style.height = `${height}px`; }
2.3. Set the zoom effect
function setAppScale(dom:HTMLElement){ const currentWidth = document.body.clientWidth; const { width } = screen || {}; dom.style.transform = `scale(${currentWidth / width})`; }
This function is triggered when the dom element changes/the window size changes.
3. Listening/removal events
Need to monitor changes in dom elements and window sizes at the same time
Dom element monitoring
Here we use MutationObserver
To monitor changes in dom elements
function observerDomResize(dom: HTMLElement, callback: () => void) { const observer = new MutationObserver(callback); observer.observe(dom, { attributes: true, attributeFilter: ['style'], attributeOldValue: true, }) return observer }
Set monitoring during the mounted cycle
export function useAutoResize() { const handleInitDom = () => { initDom(domRef.value) } onMounted(() => { initDom(domRef.value) initConifg(domRef.value) observerDomResize(domRef.value, handleInitDom) window.removeEventListener('resize', handleInitDom); }) }
But if we write it directly like this, it will frequently call the handleInitDom function, causing performance It's wasteful, so use the anti-shake function to wrap the event processing function handleInitDom before calling it.
export function useAutoResize() { const domSizeEffectDisposer: (() => void)[] = []; const debounceInitDomFun = debounce(handleInitDom, 300) onMounted(() => { initDom(domRef.value) initConifg(domRef.value) observerDomResize(domRef.value, debounceInitDomFun) window.removeEventListener('resize', debounceInitDomFun); domSizeEffectDisposer.push( () => { if (!observer) return observer.disconnect(); observer.takeRecords(); observer = null; }, () => { window.removeEventListener('resize', debounceInitDomFun); } ); }) }
If you listen to the event, you must clear it when the component is uninstalled
onUnmounted(() => { domSizeEffectDisposer.forEach(disposer => disposer()) })
The above is the detailed content of How vue3 hook reconstructs DataV's full-screen container component. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
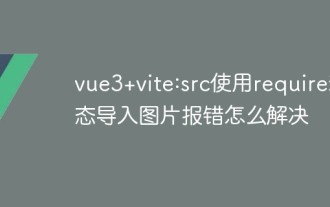
vue3+vite:src uses require to dynamically import images and error reports and solutions. vue3+vite dynamically imports multiple images. If vue3 is using typescript development, require will introduce image errors. requireisnotdefined cannot be used like vue2 such as imgUrl:require(' .../assets/test.png') is imported because typescript does not support require, so import is used. Here is how to solve it: use awaitimport
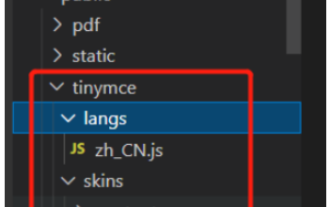
tinymce is a fully functional rich text editor plug-in, but introducing tinymce into vue is not as smooth as other Vue rich text plug-ins. tinymce itself is not suitable for Vue, and @tinymce/tinymce-vue needs to be introduced, and It is a foreign rich text plug-in and has not passed the Chinese version. You need to download the translation package from its official website (you may need to bypass the firewall). 1. Install related dependencies npminstalltinymce-Snpminstall@tinymce/tinymce-vue-S2. Download the Chinese package 3. Introduce the skin and Chinese package. Create a new tinymce folder in the project public folder and download the
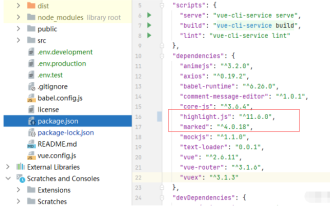
Vue implements the blog front-end and needs to implement markdown parsing. If there is code, it needs to implement code highlighting. There are many markdown parsing libraries for Vue, such as markdown-it, vue-markdown-loader, marked, vue-markdown, etc. These libraries are all very similar. Marked is used here, and highlight.js is used as the code highlighting library. The specific implementation steps are as follows: 1. Install dependent libraries. Open the command window under the vue project and enter the following command npminstallmarked-save//marked to convert markdown into htmlnpmins
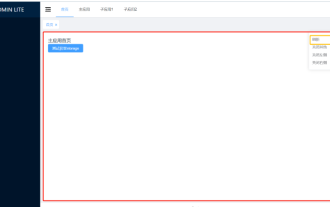
To achieve partial refresh of the page, we only need to implement the re-rendering of the local component (dom). In Vue, the easiest way to achieve this effect is to use the v-if directive. In Vue2, in addition to using the v-if instruction to re-render the local dom, we can also create a new blank component. When we need to refresh the local page, jump to this blank component page, and then jump back in the beforeRouteEnter guard in the blank component. original page. As shown in the figure below, how to click the refresh button in Vue3.X to reload the DOM within the red box and display the corresponding loading status. Since the guard in the component in the scriptsetup syntax in Vue3.X only has o
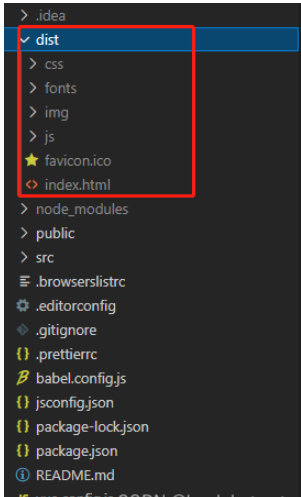
After the vue3 project is packaged and published to the server, the access page displays blank 1. The publicPath in the vue.config.js file is processed as follows: const{defineConfig}=require('@vue/cli-service') module.exports=defineConfig({publicPath :process.env.NODE_ENV==='production'?'./':'/&
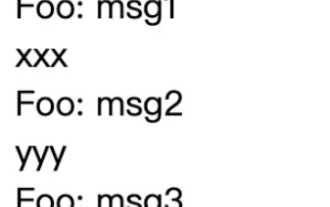
Preface Whether it is vue or react, when we encounter multiple repeated codes, we will think about how to reuse these codes instead of filling a file with a bunch of redundant codes. In fact, both vue and react can achieve reuse by extracting components, but if you encounter some small code fragments and you don’t want to extract another file, in comparison, react can be used in the same Declare the corresponding widget in the file, or implement it through renderfunction, such as: constDemo:FC=({msg})=>{returndemomsgis{msg}}constApp:FC=()=>{return(
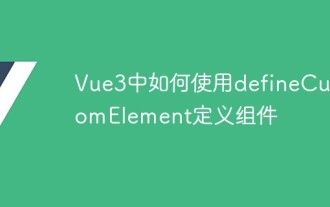
Using Vue to build custom elements WebComponents is a collective name for a set of web native APIs that allow developers to create reusable custom elements (customelements). The main benefit of custom elements is that they can be used with any framework, even without one. They are ideal when you are targeting end users who may be using a different front-end technology stack, or when you want to decouple the final application from the implementation details of the components it uses. Vue and WebComponents are complementary technologies, and Vue provides excellent support for using and creating custom elements. You can integrate custom elements into existing Vue applications, or use Vue to build
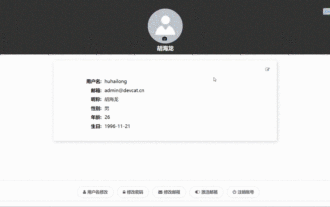
The final effect is to install the VueCropper component yarnaddvue-cropper@next. The above installation value is for Vue3. If it is Vue2 or you want to use other methods to reference, please visit its official npm address: official tutorial. It is also very simple to reference and use it in a component. You only need to introduce the corresponding component and its style file. I do not reference it globally here, but only introduce import{userInfoByRequest}from'../js/api' in my component file. import{VueCropper}from'vue-cropper&
